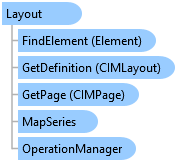
public sealed class Layout : ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase, ILayoutElementContainer, ArcGIS.Desktop.Mapping.IElementParent, System.ComponentModel.INotifyPropertyChanged, System.IDisposable
Public NotInheritable Class Layout Inherits ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase Implements ILayoutElementContainer, ArcGIS.Desktop.Mapping.IElementParent, System.ComponentModel.INotifyPropertyChanged, System.IDisposable
The Layout class provides access to all elements on a page layout. Use FindElement to reference an individual element on page layout. It is the best method to use because it also finds elements that are in group or nested group elements whereas a standard c# method used with Elements will only find non-grouped elements.
The size and positioning of a page layout can be modified. First use the GetPage property to retrieve the CIMPage, modify the page properties, and then use SetPage to the apply the changes back to the layout.
//Reference layout project items and their associated layout. //A layout project item is an item that appears in the Layouts folder in the Catalog pane. //Reference all the layout project items IEnumerable<LayoutProjectItem> layouts = Project.Current.GetItems<LayoutProjectItem>(); //Or reference a specific layout project item by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("MyLayout"));
//Import a pagx into a project. //Create a layout project item from importing a pagx file IProjectItem pagx = ItemFactory.Instance.Create(@"C:\Temp\Layout.pagx") as IProjectItem; Project.Current.AddItem(pagx);
//Create a new, basic layout and open it. //Create layout with minimum set of parameters on the worker thread Layout newLayout = await QueuedTask.Run<Layout>(() => { newLayout = LayoutFactory.Instance.CreateLayout(8.5, 11, LinearUnit.Inches); newLayout.SetName("New 8.5x11 Layout"); return newLayout; }); //Open new layout on the GUI thread await ProApp.Panes.CreateLayoutPaneAsync(newLayout);
//Create a new layout using a modified CIM and open it. //The CIM exposes additional members that may not be available through the managed API. //In this example, optional guides are added. //Create a new CIMLayout on the worker thread Layout newCIMLayout = await QueuedTask.Run<Layout>(() => { //Set up a CIM page CIMPage newPage = new CIMPage { //required parameters Width = 8.5, Height = 11, Units = LinearUnit.Inches, //optional rulers ShowRulers = true, SmallestRulerDivision = 0.5, //optional guides ShowGuides = true }; CIMGuide guide1 = new CIMGuide { Position = 1, Orientation = Orientation.Vertical }; CIMGuide guide2 = new CIMGuide { Position = 6.5, Orientation = Orientation.Vertical }; CIMGuide guide3 = new CIMGuide { Position = 1, Orientation = Orientation.Horizontal }; CIMGuide guide4 = new CIMGuide { Position = 10, Orientation = Orientation.Horizontal }; List<CIMGuide> guideList = new List<CIMGuide> { guide1, guide2, guide3, guide4 }; newPage.Guides = guideList.ToArray(); //Construct the new layout using the customized cim definitions newCIMLayout = LayoutFactory.Instance.CreateLayout(newPage); newCIMLayout.SetName("New 8.5x11 Layout"); return newCIMLayout; }); //Open new layout on the GUI thread await ProApp.Panes.CreateLayoutPaneAsync(newCIMLayout);
//Export a single page layout to PDF. //Create a PDF format with appropriate settings //BMP, EMF, EPS, GIF, JPEG, PNG, SVG, TGA, and TFF formats are also available for export PDFFormat PDF = new PDFFormat() { OutputFileName = filePath, Resolution = 300, DoCompressVectorGraphics = true, DoEmbedFonts = true, HasGeoRefInfo = true, ImageCompression = ImageCompression.Adaptive, ImageQuality = ImageQuality.Best, LayersAndAttributes = LayersAndAttributes.LayersAndAttributes }; //Check to see if the path is valid and export if (PDF.ValidateOutputFilePath()) { await QueuedTask.Run(() => layout.Export(PDF)); //Export the layout to PDF on the worker thread }
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Layouts.Layout
Target Platforms: Windows 10, Windows 8.1, Windows 7