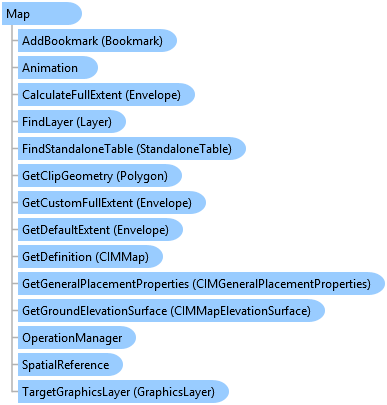
public sealed class Map : ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase, ILayerContainer, ILayerContainerEdit, IStandaloneTableContainer, IStandaloneTableContainerEdit, System.ComponentModel.INotifyPropertyChanged
Public NotInheritable Class Map Inherits ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase Implements ILayerContainer, ILayerContainerEdit, IStandaloneTableContainer, IStandaloneTableContainerEdit, System.ComponentModel.INotifyPropertyChanged
To create a Map, you must call one of the create methods of the MapFactory class.
To open a map, use ProApp.Panes.CreateMapPaneAsync method.
You can also think it as a container of layers, StandaloneTables, bookmarks etc. You need to use MapView to interact with a map. Multiple MapViews can be opened for a Map at a given time, but there can only be one active MapView which is returned by the MapView.Active static member. Use Map property of access the Map object associated with the MapView. The Map object has properties that operate on all layers within the map, such as spatial reference, reference scale, and so on, along with methods that manipulate the map's layers.
await QueuedTask.Run(() => { var map = MapFactory.Instance.CreateMap(mapName, basemap: Basemap.ProjectDefault); //TODO: use the map... });
public static async Task<Map> FindOpenExistingMapAsync(string mapName) { return await QueuedTask.Run(async () => { Map map = null; Project proj = Project.Current; //Finding the first project item with name matches with mapName MapProjectItem mpi = proj.GetItems<MapProjectItem>() .FirstOrDefault(m => m.Name.Equals(mapName, StringComparison.CurrentCultureIgnoreCase)); if (mpi != null) { map = mpi.GetMap(); //Opening the map in a mapview await ProApp.Panes.CreateMapPaneAsync(map); } return map; }); }
Map map = null; //Assume we get the selected webmap from the Project pane's Portal tab if (Project.Current.SelectedItems.Count > 0) { if (MapFactory.Instance.CanCreateMapFrom(Project.Current.SelectedItems[0])) { map = MapFactory.Instance.CreateMapFromItem(Project.Current.SelectedItems[0]); await ProApp.Panes.CreateMapPaneAsync(map); } }
/* * string url = @"c:\data\project.gdb\DEM"; //Raster dataset from a FileGeodatabase * string url = @"c:\connections\mySDEConnection.sde\roads"; //FeatureClass of a SDE * string url = @"c:\connections\mySDEConnection.sde\States\roads"; //FeatureClass within a FeatureDataset from a SDE * string url = @"c:\data\roads.shp"; //Shapefile * string url = @"c:\data\imagery.tif"; //Image from a folder * string url = @"c:\data\mySDEConnection.sde\roads"; //.lyrx or .lpkx file * string url = @"c:\data\CAD\Charlottesville\N1W1.dwg\Polyline"; //FeatureClass in a CAD dwg file * string url = @"C:\data\CAD\UrbanHouse.rvt\Architectural\Windows"; //Features in a Revit file * string url = @"http://sampleserver1.arcgisonline.com/ArcGIS/rest/services/Demographics/ESRI_Census_USA/MapServer"; //map service * string url = @"http://sampleserver6.arcgisonline.com/arcgis/rest/services/NapervilleShelters/FeatureServer/0"; //FeatureLayer off a map service or feature service */ string url = @"c:\data\project.gdb\roads"; //FeatureClass of a FileGeodatabase Uri uri = new Uri(url); await QueuedTask.Run(() => LayerFactory.Instance.CreateLayer(uri, MapView.Active.Map));
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Mapping.Map
Target Platforms: Windows 10, Windows 8.1, Windows 7