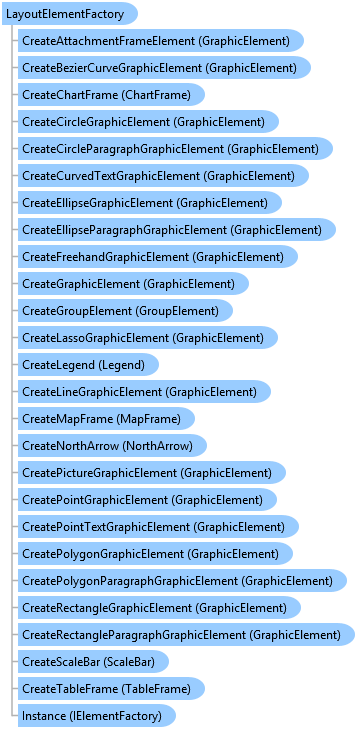
public class LayoutElementFactory : IElementFactory
Public Class LayoutElementFactory Implements IElementFactory
//Create a simple 2D point graphic and apply an existing point style item as the symbology. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D point geometry Coordinate2D coord2D = new Coordinate2D(2.0, 10.0); //(optionally) Reference a point symbol in a style StyleProjectItem ptStylePrjItm = Project.Current.GetItems<StyleProjectItem>().FirstOrDefault(item => item.Name == "ArcGIS 2D"); SymbolStyleItem ptSymStyleItm = ptStylePrjItm.SearchSymbols(StyleItemType.PointSymbol, "City Hall")[0]; CIMPointSymbol pointSym = ptSymStyleItm.Symbol as CIMPointSymbol; pointSym.SetSize(50); //Set symbolology, create and add element to layout //An alternative simple symbol is also commented out below. This would elminate the four //optional lines of code above that reference a style. //CIMPointSymbol pointSym = SymbolFactory.Instance.ConstructPointSymbol(ColorFactory.Instance.RedRGB, 25.0, SimpleMarkerStyle.Star); //Alternative simple symbol GraphicElement ptElm = LayoutElementFactory.Instance.CreatePointGraphicElement(layout, coord2D, pointSym); ptElm.SetName("New Point"); });
//Create a simple 2D line graphic and apply an existing line style item as the symbology. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2d line geometry List<Coordinate2D> plCoords = new List<Coordinate2D>(); plCoords.Add(new Coordinate2D(1, 8.5)); plCoords.Add(new Coordinate2D(1.66, 9)); plCoords.Add(new Coordinate2D(2.33, 8.1)); plCoords.Add(new Coordinate2D(3, 8.5)); Polyline linePl = PolylineBuilder.CreatePolyline(plCoords); //(optionally) Reference a line symbol in a style StyleProjectItem lnStylePrjItm = Project.Current.GetItems<StyleProjectItem>().FirstOrDefault(item => item.Name == "ArcGIS 2D"); SymbolStyleItem lnSymStyleItm = lnStylePrjItm.SearchSymbols(StyleItemType.LineSymbol, "Line with 2 Markers")[0]; CIMLineSymbol lineSym = lnSymStyleItm.Symbol as CIMLineSymbol; lineSym.SetSize(20); //Set symbolology, create and add element to layout //An alternative simple symbol is also commented out below. This would elminate the four //optional lines of code above that reference a style. //CIMLineSymbol lineSym = SymbolFactory.Instance.ConstructLineSymbol(ColorFactory.Instance.BlueRGB, 4.0, SimpleLineStyle.Solid); //Alternative simple symbol GraphicElement lineElm = LayoutElementFactory.Instance.CreateLineGraphicElement(layout, linePl, lineSym); lineElm.SetName("New Line"); });
//Create a simple 2D rectangle graphic and apply simple fill and outline symbols. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D rec_ll = new Coordinate2D(1.0, 4.75); Coordinate2D rec_ur = new Coordinate2D(3.0, 5.75); Envelope rec_env = EnvelopeBuilder.CreateEnvelope(rec_ll, rec_ur); //Set symbolology, create and add element to layout CIMStroke outline = SymbolFactory.Instance.ConstructStroke(ColorFactory.Instance.BlackRGB, 5.0, SimpleLineStyle.Solid); CIMPolygonSymbol polySym = SymbolFactory.Instance.ConstructPolygonSymbol(ColorFactory.Instance.GreenRGB, SimpleFillStyle.DiagonalCross, outline); GraphicElement recElm = LayoutElementFactory.Instance.CreateRectangleGraphicElement(layout, rec_env, polySym); recElm.SetName("New Rectangle"); });
//Create a simple point text element and assign basic symbology and text settings. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D point geometry Coordinate2D coord2D = new Coordinate2D(3.5, 10); //Set symbolology, create and add element to layout CIMTextSymbol sym = SymbolFactory.Instance.ConstructTextSymbol(ColorFactory.Instance.RedRGB, 32, "Arial", "Regular"); string textString = "Point text"; GraphicElement ptTxtElm = LayoutElementFactory.Instance.CreatePointTextGraphicElement(layout, coord2D, textString, sym); ptTxtElm.SetName("New Point Text"); //Change additional text properties ptTxtElm.SetAnchor(Anchor.CenterPoint); ptTxtElm.SetX(4.5); ptTxtElm.SetY(9.5); ptTxtElm.SetRotation(45); });
//Create rectangle text with background and border symbology. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D polygon geometry List<Coordinate2D> plyCoords = new List<Coordinate2D>(); plyCoords.Add(new Coordinate2D(3.5, 7)); plyCoords.Add(new Coordinate2D(4.5, 7)); plyCoords.Add(new Coordinate2D(4.5, 6.7)); plyCoords.Add(new Coordinate2D(5.5, 6.7)); plyCoords.Add(new Coordinate2D(5.5, 6.1)); plyCoords.Add(new Coordinate2D(3.5, 6.1)); Polygon poly = PolygonBuilder.CreatePolygon(plyCoords); //Set symbolology, create and add element to layout //Also notice how formatting tags are using within the text string. CIMTextSymbol sym = SymbolFactory.Instance.ConstructTextSymbol(ColorFactory.Instance.GreyRGB, 10, "Arial", "Regular"); string text = "Some Text String that is really long and is <BOL>forced to wrap to other lines</BOL> so that we can see the effects." as String; GraphicElement polyTxtElm = LayoutElementFactory.Instance.CreatePolygonParagraphGraphicElement(layout, poly, text, sym); polyTxtElm.SetName("New Polygon Text"); //(Optionally) Modify paragraph border CIMGraphic polyTxtGra = polyTxtElm.Graphic; CIMParagraphTextGraphic cimPolyTxtGra = polyTxtGra as CIMParagraphTextGraphic; cimPolyTxtGra.Frame.BorderSymbol = new CIMSymbolReference(); cimPolyTxtGra.Frame.BorderSymbol.Symbol = SymbolFactory.Instance.ConstructLineSymbol(ColorFactory.Instance.GreyRGB, 1.0, SimpleLineStyle.Solid); polyTxtElm.SetGraphic(polyTxtGra); });
//Create a picture element and also set background and border symbology. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D pic_ll = new Coordinate2D(6, 1); Coordinate2D pic_ur = new Coordinate2D(8, 2); Envelope env = EnvelopeBuilder.CreateEnvelope(pic_ll, pic_ur); //Create and add element to layout string picPath = @"C:\Temp\WhitePass.jpg"; GraphicElement picElm = LayoutElementFactory.Instance.CreatePictureGraphicElement(layout, env, picPath); picElm.SetName("New Picture"); //(Optionally) Modify the border and shadow CIMGraphic picGra = picElm.Graphic; CIMPictureGraphic cimPicGra = picGra as CIMPictureGraphic; cimPicGra.Frame.BorderSymbol = new CIMSymbolReference(); cimPicGra.Frame.BorderSymbol.Symbol = SymbolFactory.Instance.ConstructLineSymbol(ColorFactory.Instance.BlueRGB, 2.0, SimpleLineStyle.Solid); cimPicGra.Frame.ShadowSymbol = new CIMSymbolReference(); cimPicGra.Frame.ShadowSymbol.Symbol = SymbolFactory.Instance.ConstructPolygonSymbol(ColorFactory.Instance.BlackRGB, SimpleFillStyle.Solid); //Update the element picElm.SetGraphic(picGra); });
//Create a map frame and set its camera by zooming to the extent of an existing bookmark. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D mf_ll = new Coordinate2D(6.0, 8.5); Coordinate2D mf_ur = new Coordinate2D(8.0, 10.5); Envelope mf_env = EnvelopeBuilder.CreateEnvelope(mf_ll, mf_ur); //Reference map, create MF and add to layout MapProjectItem mapPrjItem = Project.Current.GetItems<MapProjectItem>().FirstOrDefault(item => item.Name.Equals("Map")); Map mfMap = mapPrjItem.GetMap(); MapFrame mfElm = LayoutElementFactory.Instance.CreateMapFrame(layout, mf_env, mfMap); mfElm.SetName("New Map Frame"); //Zoom to bookmark Bookmark bookmark = mfElm.Map.GetBookmarks().FirstOrDefault(b => b.Name == "Great Lakes"); mfElm.SetCamera(bookmark); });
//Create a legend for an associated map frame. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D leg_ll = new Coordinate2D(6, 2.5); Coordinate2D leg_ur = new Coordinate2D(8, 4.5); Envelope leg_env = EnvelopeBuilder.CreateEnvelope(leg_ll, leg_ur); //Reference MF, create legend and add to layout MapFrame mapFrame = layout.FindElement("New Map Frame") as MapFrame; if (mapFrame == null) { ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show("Map frame not found", "WARNING"); return; } Legend legendElm = LayoutElementFactory.Instance.CreateLegend(layout, leg_env, mapFrame); legendElm.SetName("New Legend"); });
System.Object
ArcGIS.Desktop.Layouts.LayoutElementFactory
Target Platforms: Windows 10, Windows 8.1, Windows 7