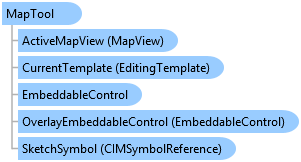
public abstract class MapTool : ArcGIS.Desktop.Framework.Contracts.Tool, System.ComponentModel.INotifyPropertyChanged
Public MustInherit Class MapTool Inherits ArcGIS.Desktop.Framework.Contracts.Tool Implements System.ComponentModel.INotifyPropertyChanged
This base class can be used to create tools to interactively identify and select features in the view or to create custom construction and editing tools. It provides virtual methods that can be overridden to perform actions when keyboard and mouse events occur in the map view, such as OnToolMouseDown and OnToolKeyDown. When overriding mouse and keyboard virtual methods if you need to execute any asynchronous code first set the handled property on the event arguments to true, override the corresponding "Handle...Async" method, and add your asynchronous code.
By setting the IsSketchTool property to true, the tool will create a sketch in the map view with a left mouse click. Use the SketchType property to set the geometry type of the sketch and use the SketchOutputMode property to specify whether the sketch geometry is in screen or map coordinates. Override OnSketchCompleteAsync to add behavior to the tool when the sketch is finished.
/* Copyright 2018 Esri Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License. */ using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using ArcGIS.Core.Geometry; using ArcGIS.Desktop.Mapping; using ArcGIS.Desktop.Framework.Threading.Tasks; using ArcGIS.Desktop.Framework.Dialogs; namespace Examples { class CustomIdentify : MapTool { public CustomIdentify() { IsSketchTool = true; SketchType = SketchGeometryType.Rectangle; //To perform a interactive selection or identify in 3D or 2D, sketch must be created in screen coordinates. SketchOutputMode = SketchOutputMode.Screen; } protected override Task<bool> OnSketchCompleteAsync(Geometry geometry) { return QueuedTask.Run(() => { var mapView = MapView.Active; if (mapView == null) return true; //Get all the features that intersect the sketch geometry and flash them in the view. var results = mapView.GetFeatures(geometry); mapView.FlashFeature(results); //Show a message box reporting each layer the number of the features. MessageBox.Show(String.Join("\n", results.Select(kvp => String.Format("{0}: {1}", kvp.Key.Name, kvp.Value.Count()))), "Identify Result"); return true; }); } } }
/* Copyright 2018 Esri Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License. */ using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using ArcGIS.Desktop.Mapping; using ArcGIS.Desktop.Framework.Threading.Tasks; namespace Examples { internal class GetMapCoordinates : MapTool { protected override void OnToolMouseDown(MapViewMouseButtonEventArgs e) { if (e.ChangedButton == System.Windows.Input.MouseButton.Left) e.Handled = true; //Handle the event args to get the call to the corresponding async method } protected override Task HandleMouseDownAsync(MapViewMouseButtonEventArgs e) { return QueuedTask.Run(() => { //Convert the clicked point in client coordinates to the corresponding map coordinates. var mapPoint = MapView.Active.ClientToMap(e.ClientPoint); ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show(string.Format("X: {0} Y: {1} Z: {2}", mapPoint.X, mapPoint.Y, mapPoint.Z), "Map Coordinates"); }); } } }
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Framework.Contracts.PlugIn
ArcGIS.Desktop.Framework.Contracts.Button
ArcGIS.Desktop.Framework.Contracts.Tool
ArcGIS.Desktop.Mapping.MapTool
Target Platforms: Windows 10, Windows 8.1, Windows 7