Summary
Finds where the source line features spatially match the target line features and transfers specified attributes from source features to matched target features.
Attribute transfer is typically used to copy attributes from features in one dataset to corresponding features in another dataset. For example, it can be used to transfer the names of road features from a previously digitized and maintained dataset to features in a new dataset that are newly collected and more accurate. The two datasets are usually referred to as source features and target features. This tool finds corresponding source and target line features within the specified search distance and transfers the specified attributes from source lines to target lines.
Illustration
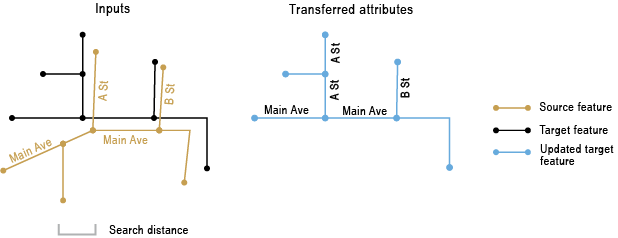
Usage
The union of input extents is used as processing extent. The counts of participating source and target features are reported in the processing messages.
One or more fields in the Transfer Field(s) parameter must be specified. If a transfer field has the same name as a field from the target feature table, the transfer field will be appended with _1 (or _2, or _3, and so on) to make it unique.
When multiple source features match one or more target features, the field values from only one of the source features are transferred to the target features. If a source field value is missing, no attribute transfer occurs.
The Search Distance parameter is used in finding match candidates. Use a distance large enough to catch most of the shifts between corresponding features, but not too large to cause unnecessary processing of too many candidates and potentially getting wrong matches.
The Output Match Table is optional. This match table provides complete feature matching information, including the source and target FIDs, match groups, match relationships, and the level of confidence of the matching derived from spatial and attribute matching conditions. This information can help you understand the match situations and facilitate postinspection, postediting, and further analysis. See About feature matching and the match table for details.
The Transfer Rule Field(s) parameter sets rules to control attribute transfer for m:n matches where multiple source features matched one or more target features. If no rules are set, attributes will be transferred from the longest of the matched source features. However, to better guide the transfer, attribute based rules can be used, and each is defined by a field name and a value.
The following field types and ruling values are supported:
Field type Ruling values Text
A string value that may exist in your source features.
Integer
Either an integer value that may exist in your source features, or MAX or MIN for the maximum or minimum value.
Date
MAX or MIN for the most recent or oldest date.
You can specify as many field/ruling value pairs as needed. The rules will be applied in the order they are listed.
When an m:n match is found, the tool will check the specified fields and ruling values and determine from which of them to transfer attributes in the following ways:
- If only one of the m source features has the ruling value in the first field in the rule list, that source feature will be used for the transfer.
- If more than one, or none, of the m source features have the ruling value, it is a tie. If no more rules are specified, the longest of them will be used for the transfer. Otherwise, the next rule in the list will be checked to break the tie.
- The process continues until all the rules have been evaluated. If no source feature can be determined for the transfer, the longest source feature will be used.
The following example explains how this process works. The ROAD_NAME field needs to be transferred from source to target for a 3:1 match. By default, source feature 3, the longest of the three source features, would be used for the transfer, and the target feature would receive the ROAD_NAME value of West Ave as shown in table (a) below.
Now, assume that the following rules are set: the field TRAVEL_DIRECTION with the rule One way and the field SPEED_LIMIT with the rule MAX. To determine the correct source feature for the transfer, the tool first evaluates the values of TRAVEL_DIRECTION for all three source features and finds that two have a match for One way. The tool then checks the SPEED_LIMIT values between these two features and settles on OBJECTID 1 which has the maximum value 40. Therefore, the ROAD_NAME of East Ave is transferred from this feature to the target feature as shown in table (b) below.
Feature matching accuracy relies on data quality, complexity, and similarities of the two inputs.
You need to minimize data errors and select relevant features as input through preprocessing. In general, it is always helpful that within an input dataset the features are topologically correct, have valid geometry, and are singlepart and nonduplicate; otherwise, unexpected results may occur.
Caution:
This tool modifies the input data. See Tools that do not create output datasets for more information and strategies to avoid undesired data changes.
Note:
All inputs must be in the same coordinate system.
Syntax
TransferAttributes(source_features, target_features, transfer_fields, search_distance, {match_fields}, {out_match_table}, {transfer_rule_fields})
Parameter | Explanation | Data Type |
source_features | Line features from which to transfer attributes. | Feature Layer |
target_features | Line features to which to transfer attributes. The specified transfer fields are added to the target features. | Feature Layer |
transfer_fields [field,...] | List of source fields to be transferred to target features. At least one field must be specified. | Field |
search_distance | The distance used to search for match candidates. A distance must be specified and it must be greater than zero. You can choose a preferred unit; the default is the feature unit. | Linear Unit |
match_fields [[source_field, target_field],...] (Optional) | Lists of fields from source and target features. If specified, each pair of fields are checked for match candidates to help determine the right match. | Value Table |
out_match_table (Optional) | The output table containing complete feature matching information. | Table |
transfer_rule_fields [[field, rule],...] (Optional) | Sets rules to control which source feature will be used to transfer attributes from when multiple source features matched target feature(s). The source feature to be used for the transfer is determined by the specified rule fields and the ruling values, which are ranked from high to low priority as they appear in the specified list. If no rules are set, the longest of the multiple matched source features will be used for the transfer. Available rule types are as follows:
| Value Table |
Derived Output
Name | Explanation | Data Type |
out_feature_class | The updated target features. | Feature Class |
Code sample
The following Python window script demonstrates how to use the TransferAttributes function in immediate mode.
import arcpy
arcpy.env.workspace = "C:/data"
arcpy.TransferAttributes_edit("source_Roads.shp",
"target_Roads.shp", ["RoadName", "PaveType"],
"25 Feet")
The following stand-alone script is an example of how to apply the TransferAttributes function in a scripting environment.
# Name: TransferAttributes_example_script2.py
# Description: Performs attribute transfer from newly updated roads (source) to existing
# base roads (target). Where the source and target features are matched in
# many-to-one or many-to-many (m:n) relationships, attributes are transferred
# from only one of the m source features to the n target features. This script
# includes a post-process that flags resulting target features with the m:n
# match relationships. You can inspect the flagged features and retrieve the
# attributes from the desired source features if necessary.
# Author: Esri
# -----------------------------------------------------------------------
# Import system modules
import arcpy
from arcpy import env
# Set environment settings
env.overwriteOutput = True
env.workspace = r"D:\conflationTools\ScriptExamples\data.gdb"
# Set local variables
sourceFeatures = "updateRoads"
targetFeatures = "baseRoads"
transfer_fields = "RD_NAME; RD_ID"
search_distance = "300 Feet"
match_fields = "RD_NAME FULLNAME"
outMatchTable = "ta_mtbl"
# Make a copy of the targetFeatures for attribute transfer
targetCopy = targetFeatures + "_Copy"
arcpy.CopyFeatures_management(targetFeatures, targetCopy)
# Performs attribute transfer
arcpy.TransferAttributes_edit(sourceFeatures, targetCopy, transfer_fields, search_distance, match_fields, outMatchTable)
# ====================================================================================
# Note 1: The result of TransferAttributes may contain errors; see tool reference.
# Additional analysis steps may be necessary to check the results; these steps
# are not included in this script.
#
# The following process identifies m:n matches between source and target features
# and flag features in targetCopy for inspection.
# ====================================================================================
# Add a field srcM_inMN to the match table to store the m count of source features in m:n relationship
field_srcM_inMN = "srcM_inMN"
arcpy.AddField_management(outMatchTable, field_srcM_inMN)
codeblock = """
def getM(fld):
x = fld.split(\":\")[0]
if x.isnumeric():
if int(x) > 0:
return int(x)
return -1
"""
# Calculate values for srcM_inMN
arcpy.CalculateField_management(outMatchTable, field_srcM_inMN, "getM(!FM_MN!)", "PYTHON_9.3", codeblock)
# Make a table view of the match table, selecting srcM_inMN values greater than 1
# (excluding 1:n relationships which don't need to be inspected)
arcpy.MakeTableView_management(outMatchTable, "mtable_view", field_srcM_inMN + "> 1")
# For the selected records, transfer srcM_inMN and SRC_FID fields and values to the targetCopy
arcpy.JoinField_management(targetCopy, "OBJECTID", "mtable_view", "TGT_FID", field_srcM_inMN + "; SRC_FID")
# ====================================================================================
# Note 2: Now the fields srcM_inMN and SRC_FID are in the copy of the target features.
# The srcM_inMN values are the counts of matched source features; the SRC_FID
# values indicate the source feature IDs from which the transferred attributes
# come from.
#
# At this point you can interactively review the transferred attributes for the
# flagged features. If you want to replace any of them by those from a different
# source feature, you would need to make the edits as needed.
# ====================================================================================
The following stand-alone script is an example of how to apply the TransferAttributes function with the use of transfer rules in a scripting environment.
# Name: TransferAttributes_example_script3.py
# Description: Performs attribute transfer from newly updated roads (source) to
# existing base roads (target). Where the source and target
# features are matched in many-to-one or many-to-many (m:n)
# relationships, attributes can be transferred based on transfer
# rules. This script shows how transfer rules are set.
# ------------------------------------------------------------------------------
# Import system modules
import arcpy
# Set environment settings
arcpy.env.overwriteOutput = True
arcpy.env.workspace = r"D:\conflationTools\ScriptExamples\data.gdb"
try:
# Set local variables
sourceFeatures = "updateRoads"
targetFeatures = "baseRoads"
transfer_fields = "RD_NAME"
search_distance = "300 Feet"
match_fields = ""
outMatchTable = ""
transfer_rules = ["TRAVEL_DIRECTION One-Way", "SPEED_LIMIT MAX"]
# Make a copy of the targetFeatures for attribute transfer
targetCopy = targetFeatures + "_Copy"
arcpy.CopyFeatures_management(targetFeatures, targetCopy)
# Performs attribute transfer
arcpy.TransferAttributes_edit(sourceFeatures, targetCopy, transfer_fields,
search_distance, match_fields, outMatchTable,
transfer_rules)
except arcpy.ExecuteError as aex:
print(arcpy.GetMessages(2))
except Exception as ex:
print(ex.args[0])
Environments
Licensing information
- Basic: No
- Standard: No
- Advanced: Yes