Summary
Calculates the optimal connection of paths between two or more input regions.
Illustration
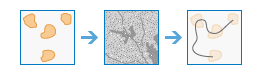
Usage
This raster analysis portal tool is available when you are signed in to an ArcGIS Enterprise
portal that has an ArcGIS Image Server
configured for Raster Analysis
. When the tool is invoked, ArcGIS Pro serves as a client and the processing happens in the servers federated with ArcGIS Enterprise. The portal tool accepts layers from your portal as input and creates output in your portal.
The input raster layer supports the following: a layer from the portal, a URI or URL to an image service, or the output from the Make Image Server Layer tool. The input feature layer can be a layer from the portal. It can also be a URI or URL to a feature service. This tool does not support local raster data or layers. While you can use local feature data and layers as input to this portal tool, best practice is to use layers from your portal as input.
In a raster, a region is a group of cells with the same value that are contiguous to one another (adjacent). When your input regions are identified by a raster, if any zones (cells with the same value) are composed of multiple regions, first run the Spatial Analyst Region Group tool as a preprocessing step to assign unique values to each region. Use the resulting raster as the input regions to the Optimal Region Connections tool.
If the input regions are raster and the range of the row IDs is very large (even if there are only a few regions), the performance of this tool may be negatively impacted.
When the region input is a feature service, the regions are converted internally to a raster before performing the analysis.
The resolution of the raster can be controlled with the Cell Size environment. By default, if no other rasters are specified in the tool, the resolution will be determined by the shorter of the width or height of the extent of the input feature, in the input spatial reference, divided by 250.
When the region input is a feature, the ObjectID field will be used as the region identifiers.
Locations identified by the Input Barrier Raster or Features parameter or cell locations with NoData in the Input Cost Raster parameter act as barriers.
The default processing extent is the same as that of the Input Cost Raster if one is provided; otherwise, it will be set to the extent of the input regions.
The Input Cost Raster cannot contain values of zero since the algorithm is a multiplicative process. If your cost raster does contain values of zero, and these values represent areas of lowest cost, change those cells to a small positive value (such as 0.01) before running this tool.
For the Output Neighboring Connections Name parameter, if a cost surface is not specified, the neighbors are identified by Euclidean distance and a region's closest neighbor is the one that is closest in distance. However, when a cost surface is provided, the neighbors are identified by cost distance and a region's closest neighbor is the least expensive one to travel to. A cost allocation operation is performed to identify which regions are neighbors to one another.
See Analysis environments and Spatial Analyst for additional details on the geoprocessing environments that apply to this tool.
Syntax
OptimalRegionConnections(inputRegionRasterOrFeatures, outputOptimalLinesName, {inputBarrierRasterOrFeatures}, inputCostRaster, {outputNeighborConnectionsName}, {distanceMethod}, {connectionsWithinRegions})
Parameter | Explanation | Data Type |
inputRegionRasterOrFeatures | The input regions to be connected by the optimal network. Regions can be defined by either raster or feature data. If the region input is raster, the regions are defined by groups of contiguous (adjacent) cells of the same value. Each region must be uniquely numbered. The cells that are not part of any region must be NoData. The raster type must be integer, and the values can be either positive or negative. If the region input is feature data, it can be polygons, lines, or points. Polygon feature regions cannot be composed of multipart polygons. | Raster Layer; Image Service; Feature Layer; String |
outputOptimalLinesName | The name of the output line feature service that connects each input region. Each path (or line) is uniquely numbered and additional fields in the attribute table store specific information about the path. Those additional fields are the following:
This information provides insight into the paths within the network. Since each path is represented by a unique line, there will be multiple lines in locations where paths travel the same route. | String |
inputBarrierRasterOrFeatures (Optional) | The dataset that defines the barriers. The barriers can be defined by an integer or a floating-point image service, or by a feature service. For an image service barrier, the barrier must have a valid value, including zero, and the areas that are not barriers must be NoData. | Raster Layer; Image Service; Feature Layer; String |
inputCostRaster | An image service defining the impedance or cost to move planimetrically through each cell. The value at each cell location represents the cost-per-unit distance for moving through the cell. Each cell location value is multiplied by the cell resolution while also compensating for diagonal movement to obtain the total cost of passing through the cell. The values of the cost raster can be integer or floating point, but they cannot be negative or zero (you cannot have a negative or zero cost). | Raster Layer; Image Service; String |
outputNeighborConnectionsName (Optional) | The output polyline feature class identifying all paths from each region to each of its closest or cost neighbors. Each path (or line) is uniquely numbered and additional fields in the attribute table store specific information about the path. Those additional fields are the following:
This information provides insight into the paths within the network and is useful when deciding which paths should be removed if necessary. Since each path is represented by a unique line, there will be multiple lines in locations where paths travel the same route. | String |
distanceMethod (Optional) | Specifies whether to calculate the distance using a planar (flat earth) or a geodesic (ellipsoid) method.
| String |
connectionsWithinRegions (Optional) | Specifies whether the paths will continue and connect within the input regions.
| String |
Derived Output
Name | Explanation | Data Type |
outputOptimalLinesFeatures |
The lines that optimally connect the regions. | Feature Class |
outputNeighborConnectionFeatures | The output neighbor connection features. | Feature Class |
Code sample
This example calculates the optimum connections between regions.
import arcpy
arcpy.OptimalRegionConnections_ra(
"https://myserver/rest/services/regions/ImageServer", "outOptimalConnections",
"https://myserver/rest/services/barriers/ImageServer",
"https://myserver/rest/services/cost/ImageServer",
"outNeighborConnections")
This example calculates the optimum connections between regions.
#-------------------------------------------------------------------------------
# Name: OptimalRegionConnections_Ex_02.py
# Description: Calculates the optimal connections between regions.
# Requirements: ArcGIS Image Server
# Import system modules
import arcpy
# Set local variables
inputRegionsLayer =
'https://MyPortal.esri.com/server/rest/services/Hosted/regions/ImageServer'
outputName = 'outOptimalConnections'
inputBarriersLayer =
'https://MyPortal.esri.com/server/rest/services/Hosted/barriers/ImageServer'
inputCostLayer =
'https://MyPortal.esri.com/server/rest/services/Hosted/cost/ImageServer'
outputName02 = 'outNeighborConnections'
distanceMethod = 'GEODESIC'
connectionsWithinRegions = 'GENERATE_CONNECTIONS'
arcpy.OptimalRegionConnections_ra(inputRegionsLayer, outputName, inputBarriersLayer,
inputCostLayer, outputName02, distanceMethod,connectionsWithinRegions)
Environments
Licensing information
- Basic: Requires ArcGIS Image Server
- Standard: Requires ArcGIS Image Server
- Advanced: Requires ArcGIS Image Server