Available with Spatial Analyst license.
Available with Image Analyst license.
Summary
Performs an integer divide on the values of two rasters on a cell-by-cell basis.
Illustration
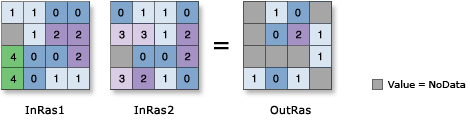
Discussion
When using an operator with a raster input the result will be a raster. However, if all inputs are numbers, then the result is a number.
When multiple operators are used in an expression, they are not necessarily executed in left-to-right order. The operator with the highest precedence value will be executed first. For more information on operator precedence, see operator precedence table. You can use parentheses to control the execution order.
The order of input is relevant for this operator.
When a number is divided by zero, the output result is NoData.
If both inputs are integers, the output contains integer values. For example, if 5 is divided by 2, the output will be 2 (the remainder is discarded).
If either input is of floating-point type, the output quotient will be floating-point. For example, if 5.3 is divided by 2, the output is 2.0 (the remainder of 0.65 is discarded).
Another way to perform the integer divide operation is a //= b, which is an alternative way to write a = a // b.
If both inputs are single band rasters, or one of the inputs is a constant, the output will be a single band raster.
If both inputs are multiband rasters, or one of the inputs is a constant, the output will be a multiband raster. The number of bands in each multiband input must be the same.
The operator will perform the operation on each band from one input against the corresponding band from the other input. If one of the inputs is a multiband raster and the other input is a constant, the operator will perform the operation against the constant value for each band in the multiband input.
Syntax
in_raster_or_constant1 // in_raster_or_constant2
Operand | Explanation | Data Type |
in_raster_or_constant1 | The input whose values will be divided by the second input. If the first input is a raster and the second is a scalar, an output raster is created with each input raster value being divided by the scalar value. | Raster Layer | Constant |
in_raster_or_constant2 | The input whose values the first input are to be divided by. If the first input is a scalar and the second is a raster, an output raster is created with each input raster value being divided into the scalar value. | Raster Layer | Constant |
Return Value
Name | Explanation | Data Type |
out_raster | The output raster object. The cell values are the quotient of the first input raster (dividend) divided by the second input (divisor). | Raster |
Code sample
This sample divides the values of the first input raster by the second.
import arcpy
from arcpy import env
from arcpy.ia import *
env.workspace = "C:/iapyexamples/data"
outDivide = Raster("degs") / Raster("negs")
outDivide.save("C:/iapyexamples/output/outdivide")
This sample divides the values of the first input raster by the second
# Name: Op_Divide_Ex_02.py
# Description: Divides the values of two rasters on a cell-by-cell basis
# Requirements: Image Analyst Extension
# Import system modules
import arcpy
from arcpy import env
from arcpy.ia import *
# Set environment settings
env.workspace = "C:/iapyexamples/data"
# Set local variables
inRaster01 = Raster("elevation")
inRaster02 = Raster("landuse")
# Execute Divide
outDivide = inRaster01 / inRaster02
# Save the output
outDivide.save("C:/iapyexamples/output/outdivide2")