Summary
Filters, clips, and reprojects the collection of lidar data referenced by a LAS dataset.
Illustration
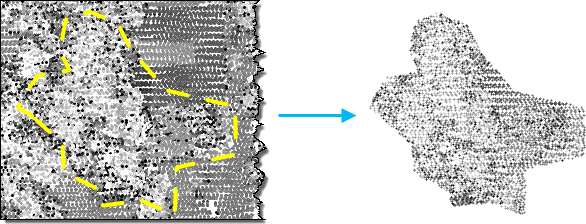
Usage
-
You can have the LAS dataset layer limit the LAS points that are displayed and processed by selecting any combination of classification codes, classification flags, and return values in the layer's filter settings. The filters can be defined through the Layer Properties dialog box or the Make LAS Dataset Layer tool.
To reproject LAS files into a different coordinate system, specify the spatial reference in the Output Coordinate System environment setting. The LAS files must have a spatial reference defined for the files to be reprojected. If the spatial reference contains a vertical coordinate system, the LAS files can also be reprojected to another height reference system if the vertical datum transformation grids are installed and a transformation is available from the source reference and the target coordinate system. A LAS file's spatial reference can be defined in either its header or through an auxiliary PRJ file that defines the spatial reference using the well-known text (WKT) convention. The PRJ file must reside in the same location as the LAS file and have the same name as the LAS file. When present, the PRJ file will override the spatial reference information in the LAS file's header. You can create PRJ files for LAS files with missing or incorrectly defined spatial reference using the Define Projection tool for an individual LAS or ZLAS file or the Create LAS Dataset tool with the PRJ option enabled.
It is not uncommon for LAS point records to be stored in the LAS file in a binary sequence that does not correspond with the spatial clustering of the points. When data of such distribution is queried, it can result in less efficient access to the binary records that represent the LAS points. Rearranging the points in the resulting LAS file will optimize the data for visualization and other spatial operations. Statistics will automatically be calculated when the rearrange option is enabled. If you choose not to rearrange the LAS points, then you can elect to enable or disable the calculation of statistics. Calculating statistics will optimize spatial queries and provide a summary of the class codes and return values that are present in the LAS file. However, it will also add time to the processing of this tool. If the resulting LAS files will not be used in ArcGIS, you may elect to disable the calculation of statistics so that the tool can execute faster.
When clipping LAS files, if an extraction extent is defined along with an extraction boundary, the intersection of both will define the coverage of the extracted LAS files.
The output LAS files will match the LAS version and point record format of the input.
Syntax
arcpy.3d.ExtractLas(in_las_dataset, target_folder, {extent}, {boundary}, {process_entire_files}, {name_suffix}, {remove_vlr}, {rearrange_points}, {compute_stats}, {out_las_dataset}, {compression})
Parameter | Explanation | Data Type |
in_las_dataset | The LAS dataset to process. | LAS Dataset Layer |
target_folder | The existing folder that the output LAS files will be written to. | Folder |
extent (Optional) | Specifies the extent of the data that will be evaluated by this tool.
| Extent |
boundary (Optional) | A polygon boundary that defines the area of the LAS files that will be clipped. | Feature Layer |
process_entire_files (Optional) | Specifies how the processing extent is applied.
| Boolean |
name_suffix (Optional) | The text that will be appended to the name of each output LAS file. Each file will inherit its base name from its source file, followed by the suffix specified in this parameter. | String |
remove_vlr (Optional) | Each LAS file may potentially contain a set of variable length records (VLRs) added by the software that produced it. The meaning of these records is typically only known by the originating software. Unless the output LAS data will be processed by an application that understands this information, retaining the VLRs may not provide any value-added functionality. Removing the VLRs could potentially save significant disk space depending on their total size and the number of files containing them.
| Boolean |
rearrange_points (Optional) | Determines whether to rearrange points in the LAS files.
| Boolean |
compute_stats (Optional) | Specifies whether statistics should be computed for the LAS files referenced by the LAS dataset. Computing statistics provides a spatial index for each LAS file, which improves analysis and display performance. Statistics also enhance the filtering and symbology experience by limiting the display of LAS attributes, like classification codes and return information, to values that are present in the LAS file.
| Boolean |
out_las_dataset (Optional) | The output LAS dataset referencing the newly created LAS files. | LAS Dataset |
compression (Optional) | Specifies whether the output LAS file will in a compressed format or the standard LAS format.
| String |
Derived Output
Name | Explanation | Data Type |
out_folder | The folder to which the LAS files will be written. | Folder |
Code sample
The following sample demonstrates the use of this tool in the Python window.
import arcpy
from arcpy import env
env.workspace = 'C:/data'
arcpy.ddd.ExtractLas('test.lasd', 'c:/lidar/subset', boundary='study_area.shp',
name_suffix='subset', remove_vlr=True,
rearrange_points='REARRANGE_POINTS',
out_las_dataset='extracted_lidar.lasd')
The following sample demonstrates the use of this tool in a stand-alone Python script.
'''****************************************************************************
Name: Split Large LAS File
Description: Divides a large LAS file whose point distribution covers the full
XY extent of the data into smaller files to optimize performance
when reading lidar data.
****************************************************************************'''
# Import system modules
import arcpy
import tempfile
import math
in_las_file = arcpy.GetParameterAsText(0)
tile_width = arcpy.GetParameter(1) # double in LAS file's XY linear unit
tile_height = arcpy.GetParameter(2) # double in LAS file's XY linear unit
out_folder = arcpy.GetParameterAsText(3) # folder for LAS files
out_name_suffix = arcpy.GetParameterAsText(4) # basename for output files
out_lasd = arcpy.GetParameterAsText(5) # output LAS dataset
try:
temp_lasd = arcpy.CreateUniqueName('temp.lasd', tempfile.gettempdir())
arcpy.management.CreateLasDataset(in_las_file, temp_lasd,
compute_stats='COMPUTE_STATS')
desc = arcpy.Describe(temp_lasd)
total_columns = int(math.ceil(desc.extent.width/tile_width))
total_rows = int(math.ceil(desc.extent.height/tile_height))
digits = int(math.log10(max(cols, rows))) + 1
for row in range(1, total_rows+1):
yMin = desc.extent.YMin + tile_height*(row-1)
yMax = desc.extent.YMin + tile_height*(row)
for col in range (1, total_columns+1):
xMin = desc.extent.XMin + tile_width*(col-1)
xMax = desc.extent.XMax + tile_width*(col)
name_suffix = '_{0}_{1}x{2}'.format(out_name_suffix,
str(row).zfill(digits),
str(col).zfill(digits))
arcpy.ddd.ExtractLas(temp_lasd, out_folder,
arcpy.Extent(xMin, yMin, xMax, yMax),
name_suffix=name_suffix,
rearrange_points='REARRANGE_POINTS',
compute_stats='COMPUTE_STATS')
arcpy.env.workspace = out_folder
arcpy.management.CreateLasDataset(arcpy.ListFiles('*{0}*.las'.format(out_name_suffix)),
out_lasd, compute_stats='COMPUTE_STATS',
relative_paths='RELATIVE_PATHS')
except arcpy.ExecuteError:
print(arcpy.GetMessages())
Licensing information
- Basic: Requires 3D Analyst
- Standard: Requires 3D Analyst
- Advanced: Requires 3D Analyst