Summary
Generates connecting lines from origin features to destination features. This is often referred to as a spider diagram.
Illustration
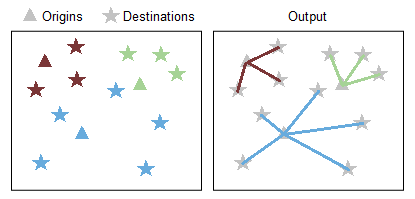
Usage
When the origin or destination features are lines or polygons, the feature centroids are used for generating links.
The output features will include the following attribute fields:
- ORIG_FID—The ObjectID field of the origin feature.
- ORIG_X—The x-coordinate of the origin feature (or centroid).
- ORIG_Y—The y-coordinate of the origin feature (or centroid).
- DEST_FID—The ObjectID field of the destination feature.
- DEST_X—The x-coordinate of the destination feature (or centroid).
- DEST_Y—The y-coordinate of the destination feature (or centroid).
- LINK_DIST—The length of the output link, measured in the specified distance unit.
- GROUP_ID—The group field values that are shared between linked pairs of origin and destination features. This field will only be added if both origin and destination group fields are specified.
- COLOR_ID—A numeric value that is used to symbolize origins or groups into at most eight unique colored links. The values are random numbers between 1 and 8.
- LINK_COUNT—The number of overlapping links. This is added when you specify to aggregate overlapping links.
- Any statistics fields specified when aggregating overlapping links will also be added to the output feature class.
The origin features and destination features can be specified as the same layer or dataset. If the same data is used for origins and destinations, links will not be generated from an origin to the destination feature with the same Object ID, as this zero-length line is a null geometry error.
This tool can be used for both one-to-one and one-to-many relationships. For example, you can link a motor vehicle theft to its recovery location (one-to-one), or perform a proximity analysis to understand the distances between a central headquarters location to several regional office locations (one-to-many).
The output links layer will include the following charts to help you visualize the result of the analysis:
- A bar chart of the count of each group ID, if you specified group fields.
- A bar chart of the sum of link lengths for each origin ID or group ID (if you specified group fields). This is useful to see if some origins or groups have large or small total distances to destinations.
- A bar chart of the mean link lengths for each origin ID or group ID (if you specified group fields). This is useful to see the average distance between origin features or groups and their linked destinations.
- A box plot of the distribution of link lengths for each origin ID or group ID (if you specified group fields). This is useful to see if most of the links for an origin or group have small or large lengths to their destinations, and the range and distribution summary of those link lengths. If an origin ID or group ID has a vertically short box, it means the distances from that origin or group to all linked destinations were similar. If an origin ID or group ID has a box that is located high on the y-axis, most of the links have a long length.
Syntax
arcpy.analysis.GenerateOriginDestinationLinks(origin_features, destination_features, out_feature_class, {origin_group_field}, {destination_group_field}, {line_type}, {num_nearest}, {search_distance}, distance_unit, {aggregate_links}, {sum_fields})
Parameter | Explanation | Data Type |
origin_features |
The input features from which links will be generated. | Feature Layer |
destination_features |
The destination features to which links will be generated. | Feature Layer |
out_feature_class |
The output polyline feature class that will contain the output links. | Feature Class |
origin_group_field (Optional) |
The attribute field from the input origin features that will be used for grouping. Features that have the same group field value between origins and destinations will be connected with a link. | Field |
destination_group_field (Optional) | The attribute field from the input destination features that will be used for grouping. Features that have the same group field value between origins and destinations will be connected with a link. | Field |
line_type (Optional) | Specifies whether a shortest path on a spheroid (geodesic) or a Cartesian projected earth (planar) will be used when generating the output links. Geodesic lines will have a slight curve when their length exceeds approximately 50 kilometers, as the curvature of the Earth makes the shortest distance between two points appear curved when viewed on a 2D map. It is recommended that you use the Geodesic line type with data stored in a coordinate system that is not appropriate for distance measurements (for example, Web Mercator and any geographic coordinate system) or any dataset that spans a large geographic area.
| String |
num_nearest (Optional) | The maximum number of links that will be generated per origin feature to the nearest destination features. If no number is specified, the tool will generate links between all origin and destination features. For example, using a value of 1 will generate links between each origin feature and its closest destination feature. | Double |
search_distance (Optional) | The maximum distance between an origin and destination feature that will produce a link feature in the output. The unit of the search distance is specified in the distance unit parameter. If no search distance is specified, the tool will generate links between all origin and destination features regardless of their distance apart. | Double |
distance_unit | Specifies the units used to measure the length of the links. Distances for each link will appear in the LINK_DIST field. If a distance unit is not specified, the distance unit of the origin features' coordinate system will be used.
| String |
aggregate_links (Optional) | Specifies whether overlapping links will be aggregated.
| Boolean |
sum_fields [sum_fields,...] (Optional) | Specifies numeric fields from the destination features containing attribute values that will be summarized when multiple links overlap. Multiple statistic and field combinations can be specified. Null values are excluded from all statistical calculations. The available statistics types are as follows:
| Value Table |
Code sample
The following demonstrates how to use the GenerateOriginDestinationLinks function in the Python window:
import arcpy
arcpy.env.workspace = "C:/data/input/genODLinks.gdb"
arcpy.GenerateOriginDestinationLinks_analysis(
"Station_100", "City_FireResponses", "Station_100_OD_Links")
The following Python script demonstrates how to use the GenerateOriginDestinationLinks function in a stand-alone script:
# Name: GenerateODLinks.py
# Description: Finds 10 nearest links within 25 miles from the origin fire
# stations to the destination response points.
# import system modules
import arcpy
# set workspace environment
arcpy.env.workspace = "C:/data/input/genODLinks.gdb"
# set required parameters
origin_features = "Station_100"
destination_features = "City_FireResponses"
out_feature_class = "Station_100_OD_Links"
# optional parameters
origin_group_field = 'STA_NUM'
destination_group_field = 'District'
line_type = 'PLANAR'
num_nearest = 10
search_distance = 25
distance_unit = 'MILES'
aggregate_links='AGGREGATE_OVERLAPPING'
sum_fields = 'TimeSpentOnCall SUM'
# make links between fire stations and call response points
arcpy.GenerateOriginDestinationLinks_analysis(
origin_features, destination_features, out_feature_class,
origin_group_field, destination_group_field, line_type, num_nearest,
search_distance, distance_unit, aggregate_links, sum_fields)
Environments
Licensing information
- Basic: Yes
- Standard: Yes
- Advanced: Yes