Summary
Combines multiple input datasets into a single, new output dataset. This tool can combine point, line, or polygon feature classes or tables.
Use the Append tool to combine input datasets with an existing dataset.
Illustration
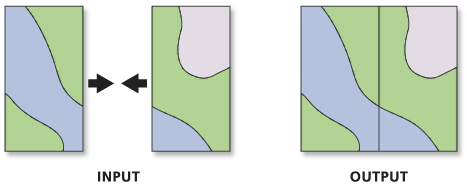
Usage
Use this tool to combine datasets from multiple sources into a new, single output dataset. All input feature classes must be of the same geometry type. For example, several point feature classes can be merged, but a line feature class cannot be merged with a polygon feature class.
Tables and feature classes can be combined in a single output dataset. The output type is determined by the first input. If the first input is a feature class, the output will be a feature class,; if the first input is a table, the output will be a table. If a table is merged into a feature class, the rows from the input table will have null geometry.
All fields in the output dataset and the contents of those fields can be controlled using the Field map.
- To change the field order, select a field name and drag it to the preferred position.
- The default data type of an output field is the same as the data type of the first input field (of that name) it encounters. You can manually change the data type at any time to any other valid data type.
- The following merge rules are available: first, last, join, sum, mean, median, mode, minimum, maximum, standard deviation, and count.
- When using the Join merge rule, you can specify a delimiter such as a space, comma, period, dash, and so on. To use a space, make sure the pointer is at the start of the input box and press the Spacebar once.
- You can specify the start and end positions of text fields using the format option.
- Do not perform standard deviation on a single input because values cannot be divided by zero, so standard deviation is not a valid option for single inputs.
This tool will not split or alter the geometries from the input datasets. All features from the input datasets will remain intact in the output dataset, even if the features overlap. To combine, or planarize, feature geometries, use the Union tool.
If feature classes are being merged, the output dataset will be in the coordinate system of the first feature class in the Input Datasets list, unless the Output Coordinate System geoprocessing environment is set.
This tool does not support annotation feature classes. Use the Append Annotation Feature Classes tool to combine annotation feature classes.
This tool does not support raster datasets. Use the Mosaic To New Raster tool to combine multiple rasters into a new output raster.
Syntax
arcpy.management.Merge(inputs, output, {field_mappings}, {add_source})
Parameter | Explanation | Data Type |
inputs [inputs,...] |
The input datasets that will be merged into a new output dataset. Input datasets can be point, line, or polygon feature classes or tables. Input feature classes must all be of the same geometry type. Tables and feature classes can be combined in a single output dataset. The output type is determined by the first input. If the first input is a feature class, the output will be a feature class; if the first input is a table, the output will be a table. If a table is merged into a feature class, the rows from the input table will have null geometry. | Table View |
output | The output dataset that will contain all combined input datasets. | Feature Class;Table |
field_mappings (Optional) | Controls which attribute fields will be in the output. By default, all fields from the inputs will be included. Fields can be added, deleted, renamed, and reordered, and you can change their properties. Merge rules allow you to specify how values from two or more input fields are merged or combined into a single output value. There are several merge rules you can use to determine how the output field will be populated with values.
In Python, you can use the FieldMappings class to define this parameter. | Field Mappings |
add_source (Optional) | Specifies whether source information will be added to the output dataset in a new text field, MERGE_SRC. The values in the MERGE_SRC field will indicate the input dataset path or layer name that is the source of each record in the output.
| Boolean |
Code sample
The following Python window script demonstrates how to use the Merge function.
import arcpy
arcpy.env.workspace = "C:/data"
arcpy.Merge_management(["majorrds.shp", "Habitat_Analysis.gdb/futrds"],
"C:/output/Output.gdb/allroads", "", "ADD_SOURCE_INFO")
Use the Merge function to move features from two street feature classes into a single dataset.
# Name: Merge.py
# Description: Use Merge to move features from two street
# feature classes into a single dataset with field mapping
# import system modules
import arcpy
# Set environment settings
arcpy.env.workspace = "C:/data"
# Street feature classes to be merged
oldStreets = "majorrds.shp"
newStreets = "Habitat_Analysis.gdb/futrds"
addSourceInfo = "ADD_SOURCE_INFO"
# Create FieldMappings object to manage merge output fields
fieldMappings = arcpy.FieldMappings()
# Add all fields from both oldStreets and newStreets
fieldMappings.addTable(oldStreets)
fieldMappings.addTable(newStreets)
# Add input fields "STREET_NAM" & "NM" into new output field
fldMap_streetName = arcpy.FieldMap()
fldMap_streetName.addInputField(oldStreets, "STREET_NAM")
fldMap_streetName.addInputField(newStreets, "NM")
# Set name of new output field "Street_Name"
streetName = fldMap_streetName.outputField
streetName.name = "Street_Name"
fldMap_streetName.outputField = streetName
# Add output field to field mappings object
fieldMappings.addFieldMap(fldMap_streetName)
# Add input fields "CLASS" & "IFC" into new output field
fldMap_streetClass = arcpy.FieldMap()
fldMap_streetClass.addInputField(oldStreets, "CLASS")
fldMap_streetClass.addInputField(newStreets, "IFC")
# Set name of new output field "Street_Class"
streetClass = fldMap_streetClass.outputField
streetClass.name = "Street_Class"
fldMap_streetClass.outputField = streetClass
# Add output field to field mappings object
fieldMappings.addFieldMap(fldMap_streetClass)
# Remove all output fields from the field mappings, except fields
# "Street_Class", "Street_Name", & "Distance"
for field in fieldMappings.fields:
if field.name not in ["Street_Class", "Street_Name", "Distance"]:
fieldMappings.removeFieldMap(fieldMappings.findFieldMapIndex(field.name))
# Since both oldStreets and newStreets have field "Distance", no field mapping
# is required
# Use Merge tool to move features into single dataset
uptodateStreets = "C:/output/Output.gdb/allroads"
arcpy.Merge_management([oldStreets, newStreets], uptodateStreets, fieldMappings,
addSourceInfo)
Environments
Licensing information
- Basic: Yes
- Standard: Yes
- Advanced: Yes