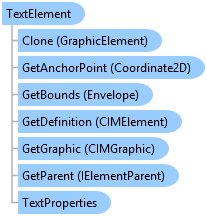
public sealed class TextElement : GraphicElement, System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable<Element>
Public NotInheritable Class TextElement Inherits GraphicElement Implements System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable(Of Element)
//Create a simple point text element and assign basic symbology and text settings. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D point geometry Coordinate2D coord2D = new Coordinate2D(3.5, 10); //Set symbolology, create and add element to layout CIMTextSymbol sym = SymbolFactory.Instance.ConstructTextSymbol(ColorFactory.Instance.RedRGB, 32, "Arial", "Regular"); string textString = "Point text"; GraphicElement ptTxtElm = LayoutElementFactory.Instance.CreatePointTextGraphicElement(layout, coord2D, textString, sym); ptTxtElm.SetName("New Point Text"); //Change additional text properties ptTxtElm.SetAnchor(Anchor.CenterPoint); ptTxtElm.SetX(4.5); ptTxtElm.SetY(9.5); ptTxtElm.SetRotation(45); });
//Create rectangle text with background and border symbology. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D polygon geometry List<Coordinate2D> plyCoords = new List<Coordinate2D>(); plyCoords.Add(new Coordinate2D(3.5, 7)); plyCoords.Add(new Coordinate2D(4.5, 7)); plyCoords.Add(new Coordinate2D(4.5, 6.7)); plyCoords.Add(new Coordinate2D(5.5, 6.7)); plyCoords.Add(new Coordinate2D(5.5, 6.1)); plyCoords.Add(new Coordinate2D(3.5, 6.1)); Polygon poly = PolygonBuilder.CreatePolygon(plyCoords); //Set symbolology, create and add element to layout //Also notice how formatting tags are using within the text string. CIMTextSymbol sym = SymbolFactory.Instance.ConstructTextSymbol(ColorFactory.Instance.GreyRGB, 10, "Arial", "Regular"); string text = "Some Text String that is really long and is <BOL>forced to wrap to other lines</BOL> so that we can see the effects." as String; GraphicElement polyTxtElm = LayoutElementFactory.Instance.CreatePolygonParagraphGraphicElement(layout, poly, text, sym); polyTxtElm.SetName("New Polygon Text"); //(Optionally) Modify paragraph border CIMGraphic polyTxtGra = polyTxtElm.GetGraphic(); CIMParagraphTextGraphic cimPolyTxtGra = polyTxtGra as CIMParagraphTextGraphic; cimPolyTxtGra.Frame.BorderSymbol = new CIMSymbolReference(); cimPolyTxtGra.Frame.BorderSymbol.Symbol = SymbolFactory.Instance.ConstructLineSymbol(ColorFactory.Instance.GreyRGB, 1.0, SimpleLineStyle.Solid); polyTxtElm.SetGraphic(polyTxtGra); });
//Modify the text properties for an existing text element. //Reference a layoutitem in a project by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("Layout")); //Perform on the worker thread await QueuedTask.Run(() => { //Reference and load the layout associated with the layout item Layout lyt = layoutItem.GetLayout(); //Reference a text element by name TextElement txtElm = lyt.FindElement("Title") as TextElement; //Change placement txtElm.SetX(4.25); txtElm.SetY(8); //Change TextProperties TextProperties txt_prop = txtElm.TextProperties; txt_prop.FontSize = 48; txt_prop.Font = "Times New Roman"; txt_prop.Text = "Some new text"; txtElm.SetTextProperties(txt_prop); });
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Layouts.Element
ArcGIS.Desktop.Layouts.GraphicElement
ArcGIS.Desktop.Layouts.TextElement
Target Platforms: Windows 10, Windows 8.1