Label | Explanation | Data Type |
Input Features | The line features to be smoothed. | Feature Layer |
Output Feature Class | The output feature class to be created. | Feature Class |
Smoothing Algorithm | Specifies the smoothing algorithm.
| String |
Smoothing Tolerance | A tolerance used by the Polynomial Approximation with Exponential Kernal (PAEK) algorithm. A tolerance must be specified, and it must be greater than zero. You can choose a preferred unit; the default is the feature unit. This parameter is unavailable when the Bezier interpolation algorithm is used. | Linear Unit |
Preserve endpoint for closed lines (Optional) | This is a legacy parameter that is no longer used. It was formerly used to specify whether endpoints of closed lines would be preserved. This parameter is still included in the tool's syntax for compatibility in scripts and models but is hidden from the tool's dialog box. Specifies whether the endpoints of closed lines will be preserved. This option works with the PAEK algorithm only.
| Boolean |
Handling Topological Errors (Optional) | Specifies how topological errors (possibly introduced in the process, such as line crossing or overlapping) will be handled.
| String |
Input Barrier Layers
(Optional) | Inputs containing features that will act as barriers for smoothing. The resulting smoothed lines will not touch or cross barrier features. For example, when smoothing contour lines, spot height features input as barriers ensure that the smoothed contour lines will not be smooth across these points. The output will not violate the elevation as described by measured spot heights. | Feature Layer |
Summary
Smooths sharp angles in lines to improve aesthetic or cartographic quality.
Learn more about how the Smooth Line and Smooth Polygon tools work.
Illustration
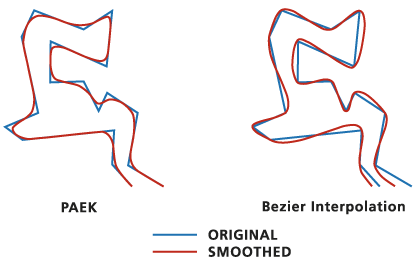
Usage
There are two smoothing methods available:
- The Polynomial Approximation with Exponential Kernel (PAEK) method (PAEK in Python) smooths lines based on a smoothing tolerance. Each smoothed line may have more vertices than its source line. The Smoothing Tolerance parameter controls the length of a moving path used in calculating the new vertices. The shorter the length, the more detail that will be preserved and the longer the processing time.
- The Bezier interpolation method (BEZIER_INTERPOLATION in Python) smooths lines without using a tolerance by creating approximated Bezier curves to match the input lines.
Use the Input barrier layers parameter to identify features that must not be crossed by smoothed lines. Barrier features can be points, lines, or polygons.
Processing large datasets may exceed memory limitations. In such cases, consider processing input data by partition by identifying a relevant polygon feature class in the Cartographic Partitions environment setting. Portions of the data, defined by partition boundaries, will be processed sequentially. The resulting feature class will be seamless and consistent at partition edges. See Generalizing large datasets using partitions for more information.
Caution:
The Cartographic Partitions environment setting is ignored when the Handling Topological Errors parameter is set to Do not check for topological errors (error_option = "NO_CHECK" in Python) or Flag topological errors (error_option = "FLAG_ERRORS" in Python).
Domains and subtypes are copied to the output even if the Transfer field domain, subtypes, and attributes rules environment is unchecked.
The output line feature class is topologically correct. Any topological errors in the input data are flagged in the output line feature class. The output feature class includes two additional fields, InLine_FID and SmoLnFlag, that contain the input feature IDs and topological errors of the input, respectively. A SmoLnFlag value of 1 indicates that a topological error is present; a value of 0 (zero) indicates that no errors are present.
Legacy:
Prior to the ArcGIS Pro 2.2 version of this tool, the Preserve endpoint for rings (endpoint_option in Python) parameter was used to specify whether the endpoint of a resulting isolated polygon ring would be preserved. This parameter is still included in the tool's syntax for compatibility in scripts and models but is now ignored and hidden on the tool's dialog box.
Parameters
arcpy.cartography.SmoothLine(in_features, out_feature_class, algorithm, tolerance, {endpoint_option}, {error_option}, {in_barriers})
Name | Explanation | Data Type |
in_features | The line features to be smoothed. | Feature Layer |
out_feature_class | The output feature class to be created. | Feature Class |
algorithm | Specifies the smoothing algorithm.
| String |
tolerance | A tolerance used by the PAEK algorithm. A tolerance must be specified, and it must be greater than zero. You can choose a preferred unit; the default is the feature unit. You must enter a 0 as a placeholder when using the BEZIER_INTERPOLATION smoothing algorithm. | Linear Unit |
endpoint_option (Optional) | This is a legacy parameter that is no longer used. It was formerly used to specify whether endpoints of closed lines would be preserved. This parameter is still included in the tool's syntax for compatibility in scripts and models but is hidden from the tool's dialog box. Specifies whether the endpoints of closed lines will be preserved. This option works with the PAEK algorithm only.
| Boolean |
error_option (Optional) | Specifies how topological errors (possibly introduced in the process, such as line crossing or overlapping) will be handled.
| String |
in_barriers [in_barriers,...] (Optional) | Inputs containing features that will act as barriers for smoothing. The resulting smoothed lines will not touch or cross barrier features. For example, when smoothing contour lines, spot height features input as barriers ensure that the smoothed contour lines will not be smooth across these points. The output will not violate the elevation as described by measured spot heights. | Feature Layer |
Code sample
The following Python window script demonstrates how to use the SmoothLine function in immediate mode.
import arcpy
import arcpy.cartography as CA
arcpy.env.workspace = "C:/data"
CA.SmoothLine("contours.shp", "C:/output/output.gdb/smoothed_contours", "PAEK", 100)
The following stand-alone script demonstrates how to use the SmoothLine function.
# Name: SmoothLine_Example2.py
# Description: Simplify and then Smooth coastlines
# Import system modules
import arcpy
import arcpy.cartography as CA
# Set environment settings
arcpy.env.workspace = "C:/data/Portland.gdb/Hydrography"
# Set local variables
inCoastlineFeatures = "coastlines"
barriers = "C:/data/Portland.gdb/Structures/buildings"
simplifiedFeatures = "C:/data/PortlandOutput.gdb/coastlines_simplified"
smoothedFeatures = "C:/data/PortlandOutput.gdb/coastlines_smoothed"
# Simplify coastlines.
CA.SimplifyLine(inCoastlineFeatures, simplifiedFeatures, "POINT_REMOVE", 50,
"RESOLVE_ERRORS", "KEEP_COLLAPSED_POINTS", "CHECK", barriers)
# Smooth coastlines.
CA.SmoothLine(simplifiedFeatures, smoothedFeatures, "PAEK", 100, "",
"FLAG_ERRORS", barriers)
Environments
Licensing information
- Basic: No
- Standard: Yes
- Advanced: Yes