Label | Explanation | Data Type |
Input Features | The input features that can be point, multipoint, line, polygon, or multipatch. | Feature Layer |
Output Feature Class | The output polygon feature class. | Feature Class |
Geometry Type (Optional) | Specifies what type of minimum bounding geometry the output polygons will represent.
| String |
Group Option (Optional) | Specifies how the input features will be grouped; each group will be enclosed with one output polygon.
| String |
Group Field(s) (Optional) | The field or fields in the input features that will be used to group features, when List is specified as Group Option. At least one group field is required for List option. All features that have the same value in the specified field or fields will be treated as a group. | Field |
Add geometry characteristics as attributes to output (Optional) | Specifies whether to add the geometric attributes in the output feature class or omit them in the output feature class.
| Boolean |
Summary
Creates a feature class containing polygons which represent a specified minimum bounding geometry enclosing each input feature or each group of input features.
Illustration
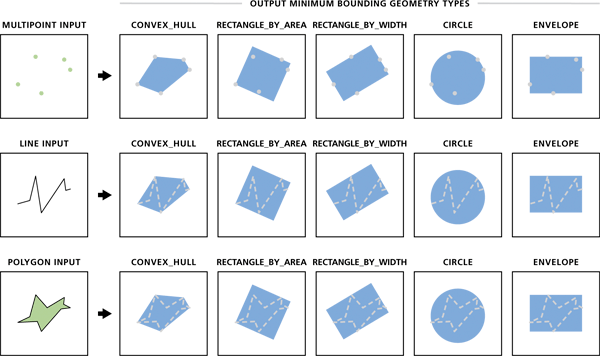
Usage
The output polygon features and their attributes will vary depending on the specified geometry type and grouping choices.
The Group Option parameter (group_option parameter in Python) will affect the output polygons and attributes in the following ways:
- Using None, none of the input features will be grouped. An output polygon feature will be created for each input feature; the resulting polygons may overlap. The attributes of the input features will be maintained in the output feature class. A new field, ORIG_FID, will be added to the output feature class and set to the input feature IDs.
- Using All, one output polygon feature will be created for all input features. The attributes of the input features will not be maintained in the output feature class.
- Using List, each set of input features with the same field values in the specified group field(s) will be treated as a group. An output polygon feature will be created for each group; the resulting polygons may overlap. The attributes of the input features used as the group field or fields will be maintained in the output feature class.
Each geometry type can be characterized by one or more unique measurements; these measurements can optionally be added to the output as new fields as described below. The width, length, and diameter values are in feature units; the orientation angles are in decimal degrees clockwise from north. The prefix, MBG_, indicates minimum bounding geometry field.
- For Rectangle by area and Rectangle by width, the new fields and measurements are:
- MBG_Width—The length of the shorter side of the resulting rectangle.
- MBG_Length—The length of the longer side of the resulting rectangle.
- MBG_Orientation—The orientation of the longer side of the resulting rectangle.
- For Envelope, the new fields and measurements are:
- MBG_Width—The length of the shorter side of the resulting rectangle.
- MBG_Length—The length of the longer side of the resulting rectangle.
- For Convex hull, the new fields and measurements are:
- MBG_Width—The shortest distance between any two vertices of the convex hull. (It may be found between more than one pair of vertices, but the first found will be used.)
- MBG_Length—The longest distance between any two vertices of the convex hull; these vertices are called antipodal pairs or antipodal points. (It may be found between more than one pair of vertices, but the first found will be used.)
- MBG_APodX1—The x coordinate of the first point of the antipodal pairs.
- MBG_APodY1—The y coordinate of the first point of the antipodal pairs.
- MBG_APodX2—The x coordinate of the second point of the antipodal pairs.
- MBG_APodY2—The y coordinate of the second point of the antipodal pairs.
- MBG_Orientation—The orientation of the imagined line connecting the antipodal pairs.
- For Circle, the new field and measurement are:
- MBG_Diameter—The diameter of the resulting circle.
- For Rectangle by area and Rectangle by width, the new fields and measurements are:
There are special cases of input features that would result in invalid (zero-area) output polygons. In these cases, a small value derived from the input feature XY Tolerance will be used as the width, length, or diameter to create output polygons. These polygons serve as 'place holders' for keeping track of features. If the resulting polygons appear 'invisible' in ArcMap using the default polygon outline width, change to a thicker outline line symbol to display them. The examples of these cases include:
- If a multipoint feature contains only one point or a group of such features are coincident, a very small square polygon will be created around the point for geometry types Rectangle by area, Rectangle by width, Convex hull, and Envelope; and a very small circle for geometry type Circle. The MBG_Width, MBG_Length, MBG_Orientation, and MBG_Diameter values will be set to zero to indicate these cases.
- If an input feature or a group of input features are perfectly aligned, for example, a horizontal or vertical line or a two-point multipoint feature, a rectangle polygon with a very small width will be created around the feature. This applies to geometry types Rectangle by area, Rectangle by width, Convex hull, and Envelope; the resulting MBG_Width value will be set to zero to indicate these cases.
Parameters
arcpy.management.MinimumBoundingGeometry(in_features, out_feature_class, {geometry_type}, {group_option}, {group_field}, {mbg_fields_option})
Name | Explanation | Data Type |
in_features | The input features that can be point, multipoint, line, polygon, or multipatch. | Feature Layer |
out_feature_class | The output polygon feature class. | Feature Class |
geometry_type (Optional) | Specifies what type of minimum bounding geometry the output polygons will represent.
| String |
group_option (Optional) | Specifies how the input features will be grouped; each group will be enclosed with one output polygon.
| String |
group_field [group_field,...] (Optional) | The field or fields in the input features that will be used to group features, when LIST is specified as group_option. At least one group field is required for LIST option. All features that have the same value in the specified field or fields will be treated as a group. | Field |
mbg_fields_option (Optional) | Specifies whether to add the geometric attributes in the output feature class or omit them in the output feature class.
| Boolean |
Code sample
The following Python window script demonstrates how to use the MinimumBoundingGeometry function in immediate mode.
import arcpy
from arcpy import env
env.workspace = "C:/data"
arcpy.MinimumBoundingGeometry_management("parks.shp",
"c:/output/output.gdb/parks_mbg",
"RECTANGLE_BY_AREA", "NONE")
The following stand-alone script is a simple example of how to apply the MinimumBoundingGeometry function in a scripting environment.
# Name: MinimumBoundingGeometry.py
# Description: Use MinimumBoundingGeometry function to find an area
# for each multipoint input feature.
# import system modules
import arcpy
from arcpy import env
# Set environment settings
env.workspace = "C:/data"
# Create variables for the input and output feature classes
inFeatures = "treeclusters.shp"
outFeatureClass = "forests.shp"
# Use MinimumBoundingGeometry function to get a convex hull area
# for each cluster of trees which are multipoint features
arcpy.MinimumBoundingGeometry_management(inFeatures, outFeatureClass,
"CONVEX_HULL", "NONE")
Environments
Licensing information
- Basic: Yes
- Standard: Yes
- Advanced: Yes