The Calculate Value tool is a versatile ModelBuilder tool that returns a value from a Python expression. The tool supports simple calculations, Python built-in functions and modules, geoprocessing functions and objects, and your own Python code.
Expression
Perform a variety of calculations using only the Expression parameter.
Math calculations
The Calculate Value tool can evaluate simple mathematical expressions in Python. For example, see the following expressions:
Operator | Explanation | Example | Result |
---|---|---|---|
x + y | x plus y | 3 + 5 | 8 |
x - y | x minus y | 4.3 - 2.1 | 2.2 |
x * y | x times y | 8 * 9 | 72 |
x / y | x divided by y | 4 / 1.25 | 3.2 |
x // y | x divided by y (floor division) | 4 // 1.25 | 3 |
x % y | remainder of x divided y | 4 % 1.25 | 0.25 |
x**y | x raised to the power of y | 2 ** 3 | 8 |
x < y | if x is less than y | 2 < 3 | 1 |
x <= y | if x is less than or equal to y | 2 <=3 | 1 |
x > y | if x is greater than y | 2 > 3 | 0 |
x >= y | if x is greater than or equal to y | 2 >= 3 | 0 |
x == y | if x is equal to y | 2 == 3 | 0 |
x != y | if x is not equal to y | 2 != 3 | 1 |
The Calculate Value tool allows the use of the Python math module to perform more complex mathematical operations.
Return the square root of a value.
Expression:
math.sqrt(25)
Return the cosine of a value in radians.
Expression:
math.cos(0.5)
Constants are also supported through the math module.
Return the constant value of π.
Expression:
math.pi
The Calculate Value tool allows the use of the random module to generate random numbers. Examples of using the random module are as follows:
Return a random integer between 0 and 10.
Expression:
random.randint(0, 10)
Return a random value derived from a normal distribution with a mean of 10 and a standard deviation of 3.
Expression:
random.normalvariate(10, 3)
String examples
Python operators and index can be used on string values.
Example | Explanation | Result |
---|---|---|
"Input" + " " + "Name" | String concatenation. | Input Name |
"Input_Name"[6:] | The seventh character to the last character. | Name |
"STREET".lower() | Convert a string value to lowercase. | street |
"Street Name".split()[1] | Split a string into multiple strings by space. And get the second returned string. | Name |
The Calculate Value tool can replace or remove characters from a string. For example, if you have an input value with a decimal (field value of the input table in this case) and want to use the value in the output name of another tool through inline variable substitution, the decimal can be replaced using the replace method.
Expression:
"%Value%".replace(".", "")
In a string, Python treats a backslash character (\) as an escape character. For example, in the string "C:\temp\newProjectFolder", \n represents a line feed, and \t represents a tab. To ensure that the string is interpreted as you expect, do one of the following:
- Use a forward slash (/) in place of a backslash.
- Use two backslashes in place of one.
- Convert the string to a string literal by placing the letter r before the string.
Data Type
The Data Type parameter specifies the data type of the Calculate Value tool output. It is essential to make sure that the Calculate Value tool output data type matches with the required input data type of the tool that follows.
You can use the output of the Calculate Value tool in any Spatial Analyst tools that accept a raster or a constant value such as Plus, Greater Than, and Less Than. To use the output of the Calculate Value tool, change the Data Type value to Formulated Raster. This data type is a raster surface whose cell values are represented by a formula or constant.
Return the calculated value in the Formulated Raster data type to be used as the input of the Greater Than tool.
Expression:
%A% + 120
The Expression parameter value uses inline variable substitution. When the tool runs, the %A% will be replaced by the value of the variable A. The value of variable A plus 120 will be used as the Input raster or constant value 1 in the Greater Than tool.
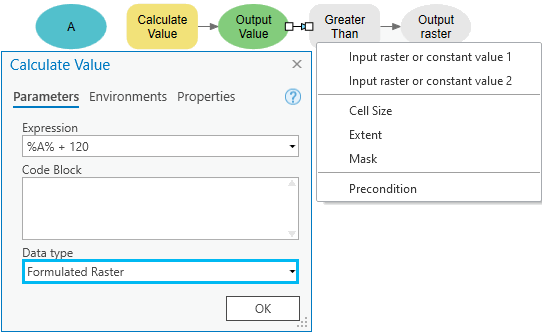
Code Block
For simple calculation, you will often only need to use the Expression parameter. For more complicated expressions, such as multiline calculations, or logical operations (if-else), you will also need the Code Block parameter. The Code Block parameter must be used in conjunction with the Expression parameter.
Variables defined in the Code Block parameter can be referenced from the Expression parameter.
For example, size is defined in the Code Block counting how many files in the folder path. It will be referenced from the Expression parameter during execution. The Calculate Value tool output value is actual file count plus five.
Expression:
5 + size
Code Block:
import os
size = 0
folderpath = r"C:\temp\csvFiles"
for ele in os.scandir(folderpath):
size += 1
You can also use the Code Block parameter to define a function and call the function from the Expression parameter. In Python, a function is defined with the def statement followed by the name of the function. A function can include required and optional arguments, or no arguments at all. Return the output of a function using the return statement.
Time
You can use the Code Block parameter to call Python modules and methods. The example below calls the time module's ctime method.
In cases in which you need to back up data regularly, adding time to the folder name helps distinguish the data. The example below demonstrates how to add a time stamp to a folder name. The time.ctime function returns the current date and time in a format such as Tue Jun 22 16:24:08 2021. This returned value cannot be used as a folder name of the Create Folder tool directly since spaces and punctuation marks are not allowed. To remove them, the Python replace method is used by stacking the method for each element that needs to be replaced. The resulting name of the folder in this example is TueJun221622522021.
Expression:
gettime()
Code Block:
import time
def gettime():
# First replace removes punctuation marks. Second replace removes spaces.
return time.ctime().replace(":", "").replace(" ", "")
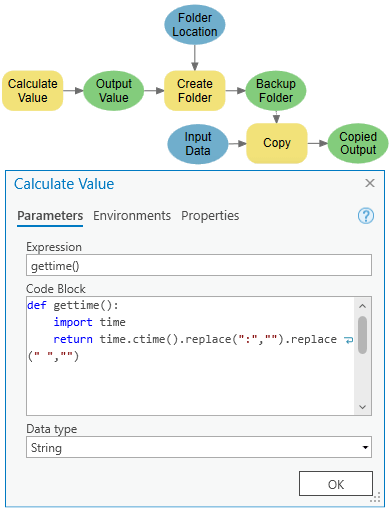
If-then-else and inline substitution
The Code Block parameter can also take in values through function input parameters. The number of parameters in the Code Block must match the number of parameters in the Expression parameter. When the tool is executed, the parameter value is passed from the Expression to the Code Block. You can pass a model variable value through using an inline variable as the Expression parameter, as shown below.
In the example below, the function getAspectDir has one parameter inValue. The Expression parameter passes the value of the Input Degree variable to the Code Block.
The following example calculates the slope aspect direction based on the Input Degree value. With the Input Degree variable value of 223, the output aspect direction returns South.
Expression:
getAspectDir("%Input Degree%")
Code Block:
def getAspectDir(inValue):
inValue = int(inValue)
if inValue >= 45 and inValue < 135:
return "East"
elif inValue >= 135 and inValue < 225:
return "South"
elif inValue >= 225 and inValue < 315:
return "West"
else:
return "North"
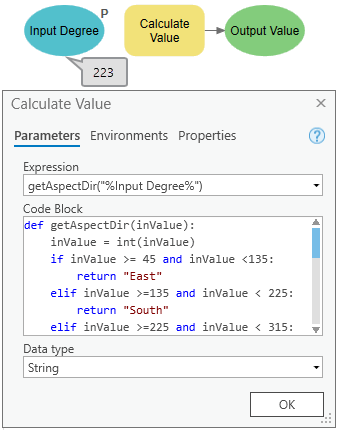
Multiple inline substitutions
The Code Block parameter can also take in multiple inline variable values.
The code block checks to see whether the value of the User Input Value variable is greater than the value of the Default Value. If so, the Calculate Value tool output value is the User Input Value. Otherwise, the output value will be the value of the Default Value. In this case, the Output Value will be 10.
Expression:
fn("%User Input Value%","%Default Value%")
Code Block:
def fn(userInputValue, defaultValue):
if float(userInputValue) > float(defaultValue):
return float(userInputValue)
else:
return float(defaultValue)
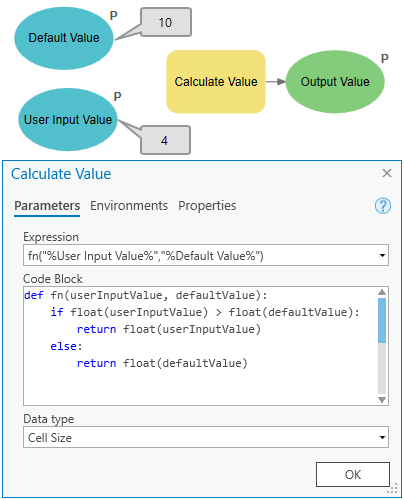
Caution:
Enclose an inline variable of type string within quotes ("%string variable%") in an expression. Inline variables of numeric types (double, long) do not require quotes (%double%).
Data path concatenation
The example below demonstrates the copying of features to a specified folder path and name. The Code Block parameter accepts two values: Folder Path and Folder Name. The code evaluates whether the combination of folder path and name exists. If the combined path does not exist, the makedirs function adds any missing folders. The letter r in the Expression parameter preceding the folder path is necessary to make sure the path is interpreted correctly.
Expression:
getPath(r"%Folder Path%", "%Folder Name%")
Code Block:
import os
def getPath(folderPath, folderName):
outPath = os.path.join(folderPath, folderName)
if not os.path.exists(outPath):
os.makedirs(outPath)
return outPath
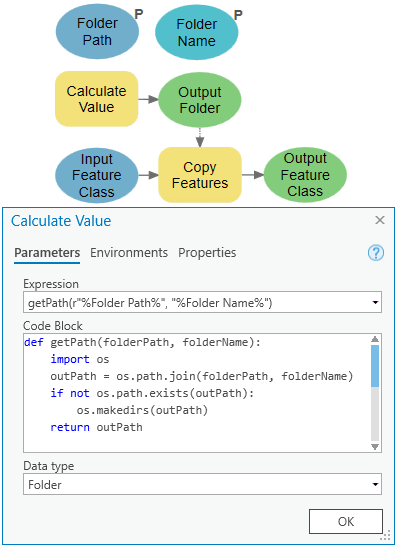
Buffer distance value and unit concatenation
To use the Calculate Value tool with a tool that accepts a linear distance, such as the Buffer tool, do the following:
- Return a distance value and a linear unit in the Code Block parameter.
- Set the Data Type parameter value to Linear Unit.
In this example, the Calculate Value tool returns a value of 12 Kilometers for use with the Buffer tool.
Expression:
fn("%A%", "%B%")
Code Block:
def fn(a, b):
distance = int(a) * int(b)
return f"{distance} Kilometers"
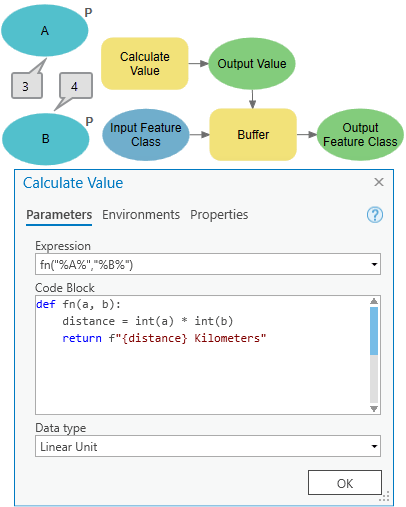
ArcPy
ArcPy is an Esri Python site package that provides a useful and productive way to perform geographic data analysis, data conversion, data management, and map automation with Python. ArcPy provides access to geoprocessing tools as well as additional functions, classes, and modules that allow you to create simple or complex workflows.
Cursor
You can use cursor for working with data. A cursor is a data access object that can be used to either iterate over the set of rows in a table or insert new rows into a table. Cursors have three forms: search, insert, and update, which are commonly used to read and write data.
To convert tree species code into its common name, you can use the UpdateCursor to iterate through each tree in the table. Based on the tree code value, assign the common name to it. For example, if a tree has code PIPO, assign its common name ponderosa pine to the CommonName field.
Expression:
fn("%trees%")
Code Block:
def fn(trees):
with arcpy.da.UpdateCursor(trees, ["Code", "CommonName"]) as cursor:
for row in cursor:
if row[0] == "PIPO":
row[1] = "ponderosa pine"
elif row[0] == "BEPA":
row[1] = "paper birch"
elif row[0] == "FAGR":
row[1] = "American beech"
cursor.updateRow(row)
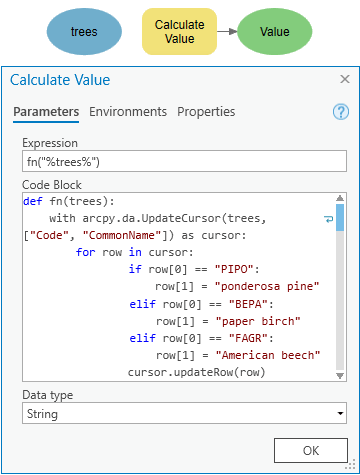
Geoprocessing tools
ArcPy provides access to geoprocessing tools as well. You can call geoprocessing tools within the Code Block parameter.
In the following example, the Calculate Value tool uses the Select Layer By Attribute, Copy Features and Buffer tools. The tools select all the roads with a LABEL field containing the word HIGHWAY, make a copy of them, and then buffer the roads.
Expression:
fn("%Input Feature Class%")
Code Block:
def fn(InputFC):
# To allow overwriting outputs change overwriteOutput option to True.
arcpy.env.overwriteOutput = True
# Process: Select Layer By Attribute (Select Layer By Attribute) (management)
InputFC_Layer, Count = arcpy.management.SelectLayerByAttribute(InputFC, "NEW_SELECTION", "LABEL LIKE '%HIGHWAY%'")
# Process: Copy Features (Copy Features) (management)
copyFeaturesOutput = "C:\\temp\\Output.gdb\\copyFeaturesOutput"
arcpy.management.CopyFeatures(InputFC_Layer, copyFeaturesOutput)
# Process: Buffer (Buffer) (analysis)
bufferOutput = "C:\\temp\\Output.gdb\\bufferOutput"
arcpy.analysis.Buffer(copyFeaturesOutput, bufferOutput, "1500 Feet")
return bufferOutput
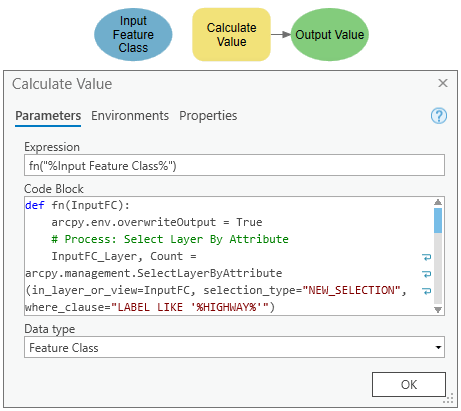