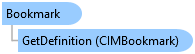
public sealed class Bookmark : ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase, System.ComponentModel.INotifyPropertyChanged
Public NotInheritable Class Bookmark Inherits ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase Implements System.ComponentModel.INotifyPropertyChanged
Bookmarks can be spatial (2D or 3D) and temporal. If your map is time-enabled, bookmarks can be created for a specific point in time. Bookmarks in an ArcGIS Pro project are associated and managed with the map they were created in. Bookmarks are transferable, so you can re-use bookmarks between multiple maps and scenes in your project, as well as with or without temporal information.
You can call the Map.GetBookmarks method on the Map to return a collection of bookmarks for the map. An similar extension method, ArcGIS.Desktop.Core.ProjectExtender.GetBookmarks, is available off of Project which will return all the bookmarks in the Project. You can use the bookmark to navigate the view by calling the MapView.ZoomTo or MapView.PanTo methods on the MapView.
public Task<bool> ZoomToBookmark(string bookmarkName) { return QueuedTask.Run(() => { //Get the active map view. var mapView = MapView.Active; if (mapView == null) return false; //Get the first bookmark with the given name. var bookmark = mapView.Map.GetBookmarks().FirstOrDefault(b => b.Name == bookmarkName); if (bookmark == null) return false; //Zoom the view to the bookmark. return mapView.ZoomTo(bookmark); }); } public async Task<bool> ZoomToBookmarkAsync(string bookmarkName) { //Get the active map view. var mapView = MapView.Active; if (mapView == null) return false; //Get the first bookmark with the given name. var bookmark = await QueuedTask.Run(() => mapView.Map.GetBookmarks().FirstOrDefault(b => b.Name == bookmarkName)); if (bookmark == null) return false; //Zoom the view to the bookmark. return await mapView.ZoomToAsync(bookmark, TimeSpan.FromSeconds(2)); }
public Task<Bookmark> AddBookmarkAsync(string name) { return QueuedTask.Run(() => { //Get the active map view. var mapView = MapView.Active; if (mapView == null) return null; //Adding a new bookmark using the active view. return mapView.Map.AddBookmark(mapView, name); }); }
public Task<ReadOnlyObservableCollection<Bookmark>> GetProjectBookmarksAsync() { //Get the collection of bookmarks for the project. return QueuedTask.Run(() => Project.Current.GetBookmarks()); }
public Task<ReadOnlyObservableCollection<Bookmark>> GetActiveMapBookmarksAsync() { return QueuedTask.Run(() => { //Get the active map view. var mapView = MapView.Active; if (mapView == null) return null; //Return the collection of bookmarks for the map. return mapView.Map.GetBookmarks(); }); }
public Task RemoveBookmarkAsync(Map map, string name) { return QueuedTask.Run(() => { //Find the first bookmark with the name var bookmark = map.GetBookmarks().FirstOrDefault(b => b.Name == name); if (bookmark == null) return; //Remove the bookmark map.RemoveBookmark(bookmark); }); }
public Task SetThumbnailAsync(Bookmark bookmark, string imagePath) { //Set the thumbnail to an image on disk, ie. C:\Pictures\MyPicture.png. BitmapImage image = new BitmapImage(new Uri(imagePath, UriKind.RelativeOrAbsolute)); return QueuedTask.Run(() => bookmark.SetThumbnail(image)); }
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Mapping.Bookmark
Target Platforms: Windows 11, Windows 10, Windows 8.1