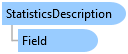
public sealed class StatisticsDescription
Public NotInheritable Class StatisticsDescription
// Calculate the Sum and Average of the Population_1990 and Population_2000 fields, grouped and ordered by Region public void CalculateStatistics(FeatureClass countryFeatureClass) { using (FeatureClassDefinition featureClassDefinition = countryFeatureClass.GetDefinition()) { // Get fields Field regionField = featureClassDefinition.GetFields() .First(x => x.Name.Equals("Region")); Field pop1990Field = featureClassDefinition.GetFields() .First(x => x.Name.Equals("Population_1990")); Field pop2000Field = featureClassDefinition.GetFields() .First(x => x.Name.Equals("Population_2000")); // Create StatisticsDescriptions StatisticsDescription pop1990StatisticsDescription = new StatisticsDescription(pop1990Field, new List<StatisticsFunction>() { StatisticsFunction.Sum, StatisticsFunction.Average }); StatisticsDescription pop2000StatisticsDescription = new StatisticsDescription(pop2000Field, new List<StatisticsFunction>() { StatisticsFunction.Sum, StatisticsFunction.Average }); // Create TableStatisticsDescription TableStatisticsDescription tableStatisticsDescription = new TableStatisticsDescription(new List<StatisticsDescription>() { pop1990StatisticsDescription, pop2000StatisticsDescription }); tableStatisticsDescription.GroupBy = new List<Field>() { regionField }; tableStatisticsDescription.OrderBy = new List<SortDescription>() { new SortDescription(regionField) }; // Calculate Statistics IReadOnlyList<TableStatisticsResult> tableStatisticsResults = countryFeatureClass.CalculateStatistics(tableStatisticsDescription); foreach (TableStatisticsResult tableStatisticsResult in tableStatisticsResults) { // Get the Region name // If multiple fields had been passed into TableStatisticsDescription.GroupBy, there would be multiple values in TableStatisticsResult.GroupBy string regionName = tableStatisticsResult.GroupBy.First().Value.ToString(); // Get the statistics results for the Population_1990 field StatisticsResult pop1990Statistics = tableStatisticsResult.StatisticsResults[0]; double population1990Sum = pop1990Statistics.Sum; double population1990Average = pop1990Statistics.Average; // Get the statistics results for the Population_2000 field StatisticsResult pop2000Statistics = tableStatisticsResult.StatisticsResults[1]; double population2000Sum = pop2000Statistics.Sum; double population2000Average = pop2000Statistics.Average; // Do something with the results here... } } }
System.Object
ArcGIS.Core.Data.StatisticsDescription
Target Platforms: Windows 11, Windows 10, Windows 8.1