Label | Explanation | Data Type |
Input Surface | The surface to use for interpolating z-values. | LAS Dataset Layer; Mosaic Layer; Raster Layer; Terrain Layer; TIN Layer; Image Service |
Input Features | The input features to process. | Feature Layer |
Output Feature Class | The feature class that will be produced. | Feature Class |
Sampling Distance (Optional) | The spacing at which z-values will be interpolated. By default, this is a raster dataset's cell size or a triangulated surface's natural densification. | Double |
Z Factor (Optional) | The factor by which z-values will be multiplied. This is typically used to convert z linear units to match x,y linear units. The default is 1, which leaves elevation values unchanged. This parameter is not available if the spatial reference of the input surface has a z datum with a specified linear unit. | Double |
Method (Optional) | Specifies the interpolation method that will be used to determine elevation values for the output features. The available options depend on the surface type being used.
| String |
Interpolate Vertices Only (Optional) | Specifies whether the interpolation will only occur along the vertices of an input feature, thereby ignoring the sample distance option. When the input surface is a raster and nearest neighbor interpolation method is selected, the z-values can only be interpolated at the feature vertices.
| Boolean |
Pyramid Level Resolution (Optional) | The z-tolerance or window-size resolution of the terrain pyramid level that will be used. The default is 0, or full resolution. | Double |
Preserve features partially outside surface
(Optional) | Specifies whether features with one or more vertices that fall outside the raster's data area will be retained in the output. This parameter is only available when the input surface is a raster and the nearest neighbor interpolation method is used.
| Boolean |
Available with 3D Analyst license.
Available with Spatial Analyst license.
Summary
Creates 3D features by interpolating z-values from a surface.
Illustration
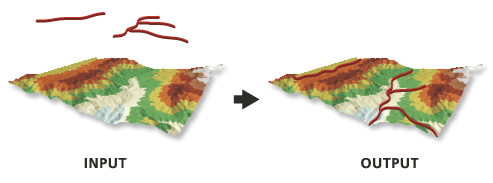
Usage
When using natural neighbors interpolation, consider specifying a sampling distance that's equal to or above half of the average point spacing of the data points in the surface.
When using the Interpolate Vertices Only option, features with vertices that fall outside the data area of the surface will not be part of the output unless the input surface is a raster and the nearest neighbor interpolation method is being used.
Parameters
InterpolateShape(in_surface, in_feature_class, out_feature_class, {sample_distance}, {z_factor}, {method}, {vertices_only}, {pyramid_level_resolution}, {preserve_features})
Name | Explanation | Data Type |
in_surface | The surface to use for interpolating z-values. | LAS Dataset Layer; Mosaic Layer; Raster Layer; Terrain Layer; TIN Layer; Image Service |
in_feature_class | The input features to process. | Feature Layer |
out_feature_class | The feature class that will be produced. | Feature Class |
sample_distance (Optional) | The spacing at which z-values will be interpolated. By default, this is a raster dataset's cell size or a triangulated surface's natural densification. | Double |
z_factor (Optional) | The factor by which z-values will be multiplied. This is typically used to convert z linear units to match x,y linear units. The default is 1, which leaves elevation values unchanged. This parameter is not available if the spatial reference of the input surface has a z datum with a specified linear unit. | Double |
method (Optional) | Specifies the interpolation method that will be used to determine elevation values for the output features. The available options depend on the surface type being used.
| String |
vertices_only (Optional) | Specifies whether the interpolation will only occur along the vertices of an input feature, thereby ignoring the sample distance option.
| Boolean |
pyramid_level_resolution (Optional) | The z-tolerance or window-size resolution of the terrain pyramid level that will be used. The default is 0, or full resolution. | Double |
preserve_features (Optional) | Specifies whether features with one or more vertices that fall outside the raster's data area will be retained in the output. This parameter is only available when the input surface is a raster and the nearest neighbor interpolation method is used.
| Boolean |
Code sample
The following sample demonstrates the use of this tool in the Python window.
from arcpy.sa import *
InterpolateShape("my_tin", "roads.shp", "roads_interp.shp")
The following sample demonstrates the use of this tool in a stand-alone Python script.
# Name: InterpolateShape_Ex_02.py
# Description: This script demonstrates how to use InterpolateShape on the 2D
# features in a target workspace.
# Requirements: Spatial Analyst Extension
# Import system modules
import arcpy
from arcpy.sa import *
# Check out the ArcGIS Spatial Analyst extension license
arcpy.CheckOutExtension("Spatial")
# Set the analysis environments
arcpy.env.workspace = "C:/arcpyExamples/data"
# Set the local variables
inFeatureClass = "point.shp"
inSurface = "dtm_tin"
OutFeatureClass = "point_interp.shp"
method = "NEAREST"
# Execute the tool
InterpolateShape(inSurface, inFeatureClass, OutFeatureClass, 15, 1, method, True)
Environments
Licensing information
- Basic: Requires Spatial Analyst or 3D Analyst
- Standard: Requires Spatial Analyst or 3D Analyst
- Advanced: Requires Spatial Analyst or 3D Analyst