Label | Explanation | Data Type |
Input Features | The input feature classes or layers. When the distance between features is less than the cluster tolerance, the features with the lower rank will snap to the feature with the higher rank. The highest rank is one. All of the input features must be polygons. | Value Table |
Output Feature Class | The feature class that will contain the results. | Feature Class |
Attributes To Join (Optional) | Specifies which attributes from the input features will be transferred to the output feature class.
| String |
XY Tolerance (Optional) | The minimum distance separating all feature coordinates (nodes and vertices) as well as the distance a coordinate can move in x or y (or both). Caution:Changing this parameter's value may cause failure or unexpected results. It is recommended that you do not modify this parameter. It has been removed from view on the tool dialog box. By default, the input feature class's spatial reference x,y tolerance property is used. | Linear Unit |
Gaps Allowed (Optional) | Specifies whether a feature will be created for areas in the output that are completely enclosed by polygons. Gaps are areas in the output feature class that are completely enclosed by other polygons (created from the intersection of features or from existing holes in the input polygons). These areas are not invalid, but you can identify them for analysis. To identify the gaps in the output, uncheck this parameter; a feature will be created in these areas. To select these features, query the output feature class based on all the input feature's FID values being equal to -1.
| Boolean |
Summary
Computes a geometric union of the input features. All features and their attributes will be written to the output feature class.
Illustration
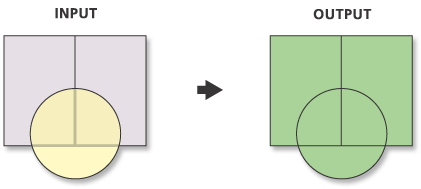
Usage
All input feature classes and feature layers must have polygon geometry.
The Allow Gaps parameter can be used with the All attributes or Only feature IDs settings in the Attributes To Join parameter. This allows identification of resulting areas that are completely enclosed by the resulting polygons. The FID attributes for these gap features will all be -1.
The output feature class will contain a FID_<name> attribute for each of the input feature classes. For example, if one of the input feature classes is named Soils, there will be a FID_Soils attribute in the output feature class. The FID_<name> values will be -1 for any input feature (or any part of an input feature) that does not intersect another input feature. Attribute values for the other feature classes in the union where no intersection is detected will not be transferred to the output feature in this case.
Attribute values from the input feature classes will be copied to the output feature class. However, if the input is a layer or layers created by the Make Feature Layer tool and a field's Use Ratio Policy is checked, then a ratio of the input attribute value is calculated for the output attribute value. When Use Ratio Policy is enabled, whenever a feature in an overlay operation is split, the attributes of the resulting features are a ratio of the attribute value of the input feature. The output value is based on the ratio in which the input feature geometry was divided. For example, if the input geometry was divided equally, each new feature's attribute value is assigned one-half of the value of the input feature's attribute value. Use Ratio Policy only applies to numeric field types.
Caution:
Geoprocessing tools do not honor geodatabase feature class or table field split policies.
This tool may generate multipart features in the output even if all inputs are single part. If you don't want multipart features, use the Multipart To Singlepart tool on the output feature class.
License:
The ArcGIS Desktop Basic and Desktop Standard licenses limit the number of input feature classes or layers to two.
Parameters
arcpy.analysis.Union(in_features, out_feature_class, {join_attributes}, {cluster_tolerance}, {gaps})
Name | Explanation | Data Type |
in_features [[in_features, {rank}],...] | The input feature classes or layers. When the distance between features is less than the cluster tolerance, the features with the lower rank will snap to the feature with the higher rank. The highest rank is one. All of the input features must be polygons. | Value Table |
out_feature_class | The feature class that will contain the results. | Feature Class |
join_attributes (Optional) | Specifies which attributes from the input features will be transferred to the output feature class.
| String |
cluster_tolerance (Optional) | The minimum distance separating all feature coordinates (nodes and vertices) as well as the distance a coordinate can move in x or y (or both). Caution:Changing this parameter's value may cause failure or unexpected results. It is recommended that you do not modify this parameter. It has been removed from view on the tool dialog box. By default, the input feature class's spatial reference x,y tolerance property is used. | Linear Unit |
gaps (Optional) | Specifies whether a feature will be created for areas in the output that are completely enclosed by polygons. Gaps are areas in the output feature class that are completely enclosed by other polygons (created from the intersection of features or from existing holes in the input polygons). These areas are not invalid, but you can identify them for analysis. To identify the gaps in the output, set this parameter to NO_GAPS, and a feature will be created in these areas. To select these features, query the output feature class based on all the input feature's FID values being equal to -1.
| Boolean |
Code sample
The following Python window script demonstrates how to use the Union function in immediate mode.
import arcpy
arcpy.env.workspace = "C:/data/data/gdb"
arcpy.analysis.Union(["well_buff50", "stream_buff200", "waterbody_buff500"],
"water_buffers", "NO_FID", 0.0003)
arcpy.analysis.Union([["counties", 2], ["parcels", 1], ["state", 2]],
"state_landinfo")
The following stand-alone script shows two ways to apply the Union function in scripting.
# unions.py
# Purpose: union 3 feature classes
# Import the system modules
import arcpy
# Set the current workspace (to avoid having to specify the full path to the
# feature classes each time)
arcpy.env.workspace = "c:/data/data.gdb"
# Union 3 feature classes but only carry the FID attributes to the output
inFeatures = ["well_buff50", "stream_buff200", "waterbody_buff500"]
outFeatures = "water_buffers"
arcpy.analysis.Union(inFeatures, outFeatures, "ONLY_FID")
# Union 3 other feature classes, but specify some ranks for each
# since parcels has better spatial accuracy
inFeatures = [["counties", 2], ["parcels", 1], ["state", 2]]
outFeatures = "state_landinfo"
arcpy.analysis.Union(inFeatures, outFeatures)
Environments
Special cases
- Parallel Processing Factor
This tool honors the Parallel Processing Factor environment. If the environment is not set (the default) or is set to 0, parallel processing will be disabled; parallel processing will not be used and processing will be done sequentially. Setting the environment to 100 will enable parallel processing; parallel processing will be used and processing will be done in parallel. Up to 10 cores will be used when parallel processing is enabled.
Parallel processing is currently supported for polygon-on-polygon, line-on-polygon, and point-on-polygon overlay operations.
Licensing information
- Basic: Limited
- Standard: Limited
- Advanced: Yes