Available with Image Analyst license.
Available with Spatial Analyst license.
Map Algebra allows you access to operators, functions, and classes through algebra. In its most basic form, an output raster is specified to the left of an equal sign (=), and the tools, operators, and their parameters are on the right. For example:
from arcpy.ia import *
elevationPlus100 = Plus("inelevation", 100)
The above statement adds 100 units to an elevation dataset, and creates a Raster object called elevationPlus100 to store the results.
Map Algebra can run simple statements, but the power of the language is realized when creating complex statements and models. As Map Algebra has been integrated in Python, all the functionality of Python and ArcPy and its extensions (modules, classes, functions, and properties) is available to you, the modeler.
As your needs grow, you can explore many of the facets of Map Algebra. The following quick tour will give you the essentials to get started.
The basics of running Map Algebra
There are three ways to use Map Algebra:
- The Raster Calculator tool
- The Python window
- Your favorite Python integrated development environment (IDE)
Raster Calculator
The Raster Calculator tool executes Map Algebra expressions. The tool has an easy-to-use calculator interface from which most Map Algebra statements can be created by simply clicking buttons. Raster Calculator can be used as a stand-alone tool, but it can also be used in ModelBuilder. As a result, the tool allows the power of Map Algebra to be integrated into ModelBuilder.
In the above expression, three rasters are combined by multiplying the second and third rasters together, and adding that result to the first. Note that operators follow a defined order of precedence.
The Raster Calculator tool is not intended to replace other Image Analyst or Spatial Analyst tools. Continue to use the other tools for the appropriate calculations. For example, use the Weighted Sum tool to overlay several weighted rasters. The Raster Calculator tool is designed to execute single-line algebraic statements.
Since Raster Calculator is a geoprocessing tool, like all tools, it can be integrated into ModelBuilder. See the following topics for more information:
Python window
The Python window is an efficient and convenient location to use geoprocessing tools and Python functionality from within ArcGIS. The Python commands run from this window can range from single lines of code to complex blocks with logic. The Python window also provides a place to access additional functionality using custom or third-party Python modules and libraries.
To launch the Python window, click the Python button in the Geoprocessing group on the Analysis tab, or from the Windows group on the View tab.
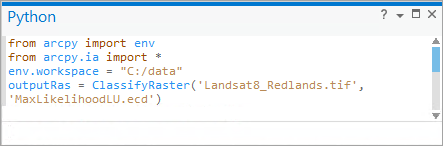
In the above sequence of statements, the ArcPy site package, geoprocessing environments, and Image Analyst modules are imported, the workspace is set, and the Classify Raster tool is run. Once a carriage return is entered at the end of a statement, that statement is immediately run.
Some features of the Python window include built-in line autocompletion, use of variables, and access to Python and ArcPy functionality.
Python Integrated Development Environment
Even though there is no limit to the number of statements that can be entered within the Python window in ArcGIS Pro, it may be cumbersome to create more complex models. The Image Analyst modules' tools, operators, functions, and classes can also be accessed from your favorite integrated development environment such as PythonWin. Start your preferred IDE and enter the desired statements.
In the following script, ArcPy, the geoprocessing environments, and the Image Analyst module are imported; variables are set; the extension is checked out; the Classify Raster tool is run; and the output is saved.
# Name: Image Classification
# Description:
# Requirements: Image Analyst Extension
# Import system modules
import arcpy
from arcpy import env
from arcpy.ia import *
# Check out the ArcGIS Image Analyst extension license
arcpy.CheckOutExtension("ImageAnalyst")
# Set environment settings
env.workspace = "C:/data"
# Set local variables
inRaster = "Landsat8_Redlands.tif"
classification_file = "LandCover.ecd"
# Execute Classify Raster
outLandCover = ClassifyRaster(inRaster, classification_file)
# Save the output
outLandCover.save("C:/data/Landcover.tif")
As is the case with the Python window, an IDE will provide access to all available Python and ArcPy functionality.
Working with operators
Map Algebra supports a series of operators (for example, +, -, and *). These same operators also exist in Python but are modified for Map Algebra to handle Raster objects differently. For example, the following adds two numbers together into a variable:
# set outVar to 14 using the Python + operator
outVar = 5 + 9
To distinguish that the statement should work on rasters (that is, to use the Map Algebra operator), you must cast the dataset as a Raster. The following example uses the Map Algebra + operator to add two rasters together:
outRas = Raster("inras1") + Raster("inras2")
Operators can accept a mixture of rasters and numbers. For example, the following adds a constant value of 8 to all the cells in the input raster:
outRas = Raster("inras1") + 8
Creating complex expressions
Tools and operators can be strung together in a single statement. The following example runs several tools and operators in each expression:
outRas = Slope("indem" * 2) / 57
outdist = EucDistance(ExtractByAttributes("inras", "Value > 105"))
Parentheses can be used to control the order of processing. Consider the following two examples, which use the same operators but yield different results due to the use of parentheses:
outRas1 = (Raster("inras1") + Raster("inras2")) / Raster("inras3")
and
outRas2 = Raster("inras1") + Raster("inras2") / Raster("inras3")
In the first statement, inras1 is added to inras2, and the result is divided by inras3. Without the parentheses, as in the second statement, inras2 would be divided by inras3, and the result would be added to inras1.
Suggestions for executing Map Algebra statements
In all the Map Algebra examples shown below, the output is a Raster object. The Raster object points to a temporary raster dataset that, unless it is explicitly saved, will be removed when the ArcGIS session ends. To permanently save the temporary dataset, the save method is called on the Raster object (see the two examples below).
An example demonstrating the workspace environment is:
import arcpy
from arcpy import env
from arcpy.ia import *
env.workspace = "C:/data"
outLandCover = ClassifyRaster("Landsat8_Redlands", "LandCover.ecd")
outLandCover.save("RedlandsLandcover")
In the above statement, the workspace is set, therefore, RedlandsLandcover will be saved in C:/data.
Further reading
To gain a deeper understanding of ArcPy, explore these topics:
To obtain more information on geoprocessing in Python, the following may be helpful: