Each feature in a feature class contains a set of points defining the vertices of a polygon or line, or a single coordinate defining a point feature. These points can be accessed with geometry objects (Polygon, Polyline, PointGeometry, or MultiPoint), which returns them in an array of Point objects.
Features can have multiple parts. The geometry object's partCount property returns the number of parts for a feature. The getPart method returns an array of point objects for a particular part of the geometry if an index is specified. If an index is not specified, an array containing an array of point objects for each geometry part is returned.
PointGeometry features return a single Point object instead of an array of point objects. All other feature types—polygon, polyline, and multipoint—return an array of point objects, or if the feature has multiple parts, an array containing multiple arrays of point objects.
If a polygon contains holes, it consists of a number of rings. The array of point objects returned for a polygon contains the points for the exterior ring and all inner rings. The exterior ring is always returned first, followed by inner rings, with null point objects as the separator between rings. Whenever a script is reading coordinates for polygons in a geodatabase or shapefile, it should contain logic for handling inner rings if this information is required by the script; otherwise, only the exterior ring is read.
A multipart feature is composed of more than one physical part but only references one set of attributes in the database. For example, in a layer of states, the state of Hawaii could be considered a multipart feature. Although composed of many islands, it would be recorded in the database as one feature.
A ring is a closed path that defines a two-dimensional area. A valid ring consists of a valid path, such that the from and to points of the ring have the same x,y coordinates. A clockwise ring is an exterior ring, and a counterclockwise ring defines an interior ring.
Learn more about writing geometries
Using geometry tokens
Geometry tokens can also be used as shortcuts in place of accessing full geometry objects. Additional geometry tokens can be used to access specific geometry information. Accessing the full geometry is more time-consuming. If you only need specific properties of the geometry, use tokens to provide shortcuts to access geometry properties. For instance, SHAPE@XY returns a tuple of x,y coordinates that represent the feature's centroid.
Token | Explanation |
---|---|
SHAPE@ | A geometry object for the feature. |
SHAPE@XY | A tuple of the feature's centroid x,y coordinates. |
SHAPE@TRUECENTROID | A tuple of the feature's centroid x,y coordinates. This returns the same value as SHAPE@XY. |
SHAPE@X | A double of the feature's x-coordinate. |
SHAPE@Y | A double of the feature's y-coordinate. |
SHAPE@Z | A double of the feature's z-coordinate. |
SHAPE@M | A double of the feature's m-value. |
SHAPE@JSON | The Esri JSON string representing the geometry. |
SHAPE@WKB | The well-known binary (WKB) representation for OGC geometry. It provides a portable representation of a geometry value as a contiguous stream of bytes. |
SHAPE@WKT | The well-known text (WKT) representation for OGC geometry. It provides a portable representation of a geometry value as a text string. |
SHAPE@AREA | A double of the feature's area. |
SHAPE@LENGTH | A double of the feature's length. |
Reading point geometries
The examples below use SearchCursor to print the coordinates for all features.
import arcpy
infc = arcpy.GetParameterAsText(0)
# Enter for loop for each feature
for row in arcpy.da.SearchCursor(infc, ["SHAPE@XY"]):
# Print x,y coordinates of each point feature
x, y = row[0]
print("{}, {}".format(x, y))
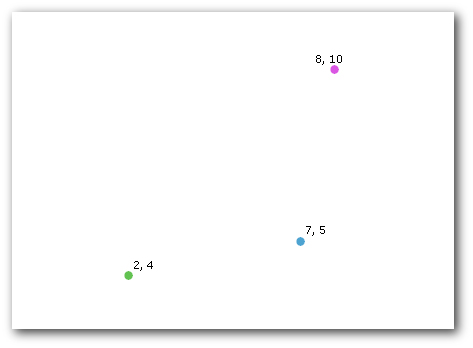
With the above feature class, the script returns the following information:
2.0 4.0
8.0 10.0
7.0 5.0
Reading multipoint geometries
import arcpy
infc = arcpy.GetParameterAsText(0)
# Enter for loop for each feature
for row in arcpy.da.SearchCursor(infc, ["OID@", "SHAPE@"]):
# Print the current multipoint's ID
print("Feature {}:".format(row[0]))
# For each point in the multipoint feature,
# print the x,y coordinates
for pnt in row[1]:
print("{}, {}".format(pnt.X, pnt.Y))
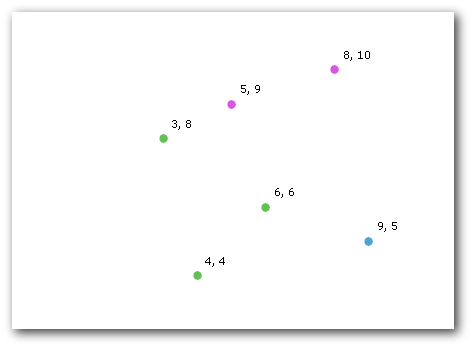
With the feature class above, the script returns the following information:
Feature 0:
3.0 8.0
4.0 4.0
6.0 6.0
Feature 1:
5.0 9.0
8.0 10.0
Feature 2:
9.0 5.0
Reading polyline or polygon geometries
import arcpy
infc = arcpy.GetParameterAsText(0)
# Enter for loop for each feature
for row in arcpy.da.SearchCursor(infc, ["OID@", "SHAPE@"]):
# Print the current polygon or polyline's ID
print("Feature {}:".format(row[0]))
partnum = 0
# Step through each part of the feature
for part in row[1]:
# Print the part number
print("Part {}:".format(partnum))
# Step through each vertex in the feature
for pnt in part:
if pnt:
# Print x,y coordinates of current point
print("{}, {}".format(pnt.X, pnt.Y))
else:
# If pnt is None, this represents an interior ring
print("Interior Ring:")
partnum += 1
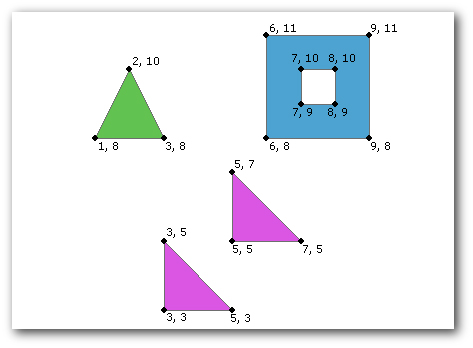
With the feature class above, the script returns the information below. Feature 0 is a single-part polygon, feature 1 is a two-part polygon, and feature 2 is a single-part polygon with an interior ring.
Feature 0:
Part 0:
3.0 8.0
1.0 8.0
2.0 10.0
3.0 8.0
Feature 1:
Part 0:
5.0 3.0
3.0 3.0
3.0 5.0
5.0 3.0
Part 1:
7.0 5.0
5.0 5.0
5.0 7.0
7.0 5.0
Feature 2:
Part 0:
9.0 11.0
9.0 8.0
6.0 8.0
6.0 11.0
9.0 11.0
Interior Ring:
7.0 10.0
7.0 9.0
8.0 9.0
8.0 10.0
7.0 10.0