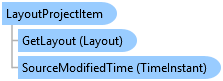
public sealed class LayoutProjectItem : ArcGIS.Desktop.Internal.Core.ProjectItem, ArcGIS.Desktop.Core.IMetadata, ArcGIS.Desktop.Core.IProjectItem, System.ComponentModel.INotifyPropertyChanged
Public NotInheritable Class LayoutProjectItem Inherits ArcGIS.Desktop.Internal.Core.ProjectItem Implements ArcGIS.Desktop.Core.IMetadata, ArcGIS.Desktop.Core.IProjectItem, System.ComponentModel.INotifyPropertyChanged
Each Layout in a project is associated with a LayoutProjectItem. This item contains numerous read-only metadata properties about the layout. Although a layout may exist in the project, it may not be loaded (an open layout view). To reference the actual layout and ensure it is loaded into memory, you must use the GetLayout method.
LayoutProjectItem layoutProjItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("myLayout"));
//Gets all the layouts in the current project var projectLayouts = Project.Current.GetItems<LayoutProjectItem>(); foreach (var layoutItem in projectLayouts) { //Do Something with the layout }
//GetItems searches project content var map = Project.Current.GetItems<MapProjectItem>().FirstOrDefault(m => m.Name == "Map1"); var layout = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(m => m.Name == "Layout1"); var folders = Project.Current.GetItems<FolderConnectionProjectItem>(); var style = Project.Current.GetItems<StyleProjectItem>().FirstOrDefault(s => s.Name == "ArcGIS 3D"); //Find item uses a catalog path. The path can be to a file or dataset var fcPath = @"C:\Pro\CommunitySampleData\Interacting with Maps\Interacting with Maps.gdb\Crimes"; var pdfPath = @"C:\Temp\Layout1.pdf"; var imgPath = @"C:\Temp\AddinDesktop16.png"; var fc = Project.Current.FindItem(fcPath); var pdf = Project.Current.FindItem(pdfPath); var img = Project.Current.FindItem(imgPath);
//This example references a graphic element on a layout and sets its Transparency property (which is not available in the managed API) //by accessing the element's CIMGraphic. //Added references using ArcGIS.Core.CIM; //CIM using ArcGIS.Desktop.Core; //Project using ArcGIS.Desktop.Layouts; //Layout class using ArcGIS.Desktop.Framework.Threading.Tasks; //QueuedTask public class GraphicElementExample1 { public static Task<bool> UpdateElementTransparencyAsync(string LayoutName, string ElementName, int TransValue) { //Reference a layoutitem in a project by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals(LayoutName)); if (layoutItem == null) return Task.FromResult(false); return QueuedTask.Run<bool>(() => { //Reference and load the layout associated with the layout item Layout lyt = layoutItem.GetLayout(); //Reference a element by name GraphicElement graElm = lyt.FindElement(ElementName) as GraphicElement; if (graElm == null) return false; //Modify the Transparency property that exists only in the CIMGraphic class. CIMGraphic CIMGra = graElm.GetGraphic() as CIMGraphic; CIMGra.Transparency = TransValue; //e.g., TransValue = 50 graElm.SetGraphic(CIMGra); return true; }); } }
//Reference the layout associated with a layout project item. LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("Layout Name")); Layout layout = await QueuedTask.Run(() => layoutItem.GetLayout()); //Perform on the worker thread
//Open the layout properties dialog. //Get the layout associated with a layout project item LayoutProjectItem lytItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("Layout Name")); Layout lyt = await QueuedTask.Run(() => lytItem.GetLayout()); //Worker thread //Open the properties dialog lyt.ShowProperties(); //GUI thread
//Report the event args when a layout is added. //At 2.x - ArcGIS.Desktop.Layouts.Events.LayoutAddedEvent.Subscribe((args) => //{ // System.Windows.MessageBox.Show("LayoutAddedEvent:" + // Environment.NewLine + // " arg Layout: " + args.Layout.Name); //}); //Use ProjectItemsChangedEvent at 3.x ArcGIS.Desktop.Core.Events.ProjectItemsChangedEvent.Subscribe((args) => { //Layout added. Layout removed would be NotifyCollectionChangedAction.Remove if (args.Action == System.Collections.Specialized.NotifyCollectionChangedAction.Add && args.ProjectItem is LayoutProjectItem layoutProjectItem) { var layout_name = layoutProjectItem.Name; var layout = layoutProjectItem.GetLayout(); System.Diagnostics.Debug.WriteLine($"Layout Added: {layout_name}"); } });
//Report the event args when a layout is removed. //At 2.x - ArcGIS.Desktop.Layouts.Events.LayoutRemovedEvent.Subscribe((args) => //{ // System.Windows.MessageBox.Show("LayoutViewEvent:" + // Environment.NewLine + // " arg Layout: " + args.Layout.Name); //}); //Use ProjectItemsChangedEvent at 3.x ArcGIS.Desktop.Core.Events.ProjectItemsChangedEvent.Subscribe((args) => { //Layout added. Layout removed would be NotifyCollectionChangedAction.Remove if (args.Action == System.Collections.Specialized.NotifyCollectionChangedAction.Remove && args.ProjectItem is LayoutProjectItem layoutProjectItem) { var layout_name = layoutProjectItem.Name; System.Diagnostics.Debug.WriteLine($"Layout Removed: {layout_name}"); } });
//Report the event args when a layout is about to be removed. //At 2.x - ArcGIS.Desktop.Layouts.Events.LayoutRemovingEvent.Subscribe((args) => //{ // if (args.LayoutPath == "CIMPATH=layout.xml") // { // args.Cancel = true; // } // return Task.FromResult(0); //}); //At 3.x use ProjectItemRemovingEvent ArcGIS.Desktop.Core.Events.ProjectItemRemovingEvent.Subscribe((args) => { var layoutItems = args.ProjectItems.ToList().OfType<LayoutProjectItem>() ?? new List<LayoutProjectItem>(); var layoutName = "DontDeleteThisOne"; foreach(var layoutItem in layoutItems) { if (layoutItem.Name == layoutName) { args.Cancel = true;//Cancel the remove break; } } return Task.FromResult(0); });
//This example deletes a layout in a project after finding it by name. //Added references using ArcGIS.Desktop.Core; using ArcGIS.Desktop.Layouts; public class DeleteLayoutExample { public static Task<bool> DeleteLayoutAsync(string LayoutName) { //Reference a layoutitem in a project by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals(LayoutName)); //Check for layoutItem if (layoutItem == null) return Task.FromResult<bool>(false); //Delete the layout from the project return Task.FromResult<bool>(Project.Current.RemoveItem(layoutItem)); } }
//Find and close all layout panes associated with a specific layout. LayoutProjectItem findLytItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("Layout")); Layout findLyt = await QueuedTask.Run(() => findLytItem.GetLayout()); //Perform on the worker thread var panes = ProApp.Panes.FindLayoutPanes(findLyt); foreach (Pane pane in panes) { ProApp.Panes.CloseLayoutPanes(findLyt.URI); //Close the pane }
//Reference layout project items and their associated layout. //A layout project item is an item that appears in the Layouts //folder in the Catalog pane. //Reference all the layout project items IEnumerable<LayoutProjectItem> layouts = Project.Current.GetItems<LayoutProjectItem>(); //Or reference a specific layout project item by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>() .FirstOrDefault(item => item.Name.Equals("MyLayout"));
//Open a layout project item in a new view. //A layout project item may exist but it may not be open in a view. //Reference a layout project item by name LayoutProjectItem someLytItem = Project.Current.GetItems<LayoutProjectItem>() .FirstOrDefault(item => item.Name.Equals("MyLayout")); //Get the layout associated with the layout project item Layout layout = await QueuedTask.Run(() => someLytItem.GetLayout()); //Worker thread //Create the new pane - call on UI ILayoutPane iNewLayoutPane = await ProApp.Panes.CreateLayoutPaneAsync(layout); //GUI thread
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Core.Item
ArcGIS.Desktop.Layouts.LayoutProjectItem
Target Platforms: Windows 11, Windows 10