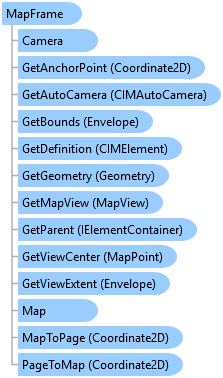
public sealed class MapFrame : Element, ArcGIS.Desktop.Mapping.IElement, System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IDisposable, System.IEquatable<Element>
Public NotInheritable Class MapFrame Inherits Element Implements ArcGIS.Desktop.Mapping.IElement, System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IDisposable, System.IEquatable(Of Element)
The MapFrame class primarily manages the placement of maps and scenes on a page layout. You can use Map and SetMap members to get and set the Map associated with a MapFrame.
The Export method allows you to export only the contents of a map frame instead of, for example, exporting an entire page layout.
If you want to change the geographic extent within a map frame, you can use the Camera and SetCamera members to get and set the Camera associated with a MapFrame. SetCamera is an overloaded method that allows you to change the map frame extent to a camera position, a bookmark location, and so on.
//Create a scale bar for a specific map frame and assign a scale bar style item. //Construct on the worker thread await QueuedTask.Run(() => { //Reference a North Arrow in a style StyleProjectItem stylePrjItm = Project.Current.GetItems<StyleProjectItem>().FirstOrDefault(item => item.Name == "ArcGIS 2D"); ScaleBarStyleItem sbStyleItm = stylePrjItm.SearchScaleBars("Double Alternating Scale Bar 1")[0]; //Build geometry Coordinate2D center = new Coordinate2D(7, 8); //Reference MF, create north arrow and add to layout MapFrame mf = layout.FindElement("New Map Frame") as MapFrame; if (mf == null) { ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show("Map frame not found", "WARNING"); return; } //At 2.x - //ScaleBar sbElm = LayoutElementFactory.Instance.CreateScaleBar(layout, center, mf, sbStyleItm); //sbElm.SetName("New Scale Bar"); //sbElm.SetWidth(2); //sbElm.SetX(6); //sbElm.SetY(7.5); var sbInfo = new ScaleBarInfo() { MapFrameName = mf.Name, ScaleBarStyleItem = sbStyleItm }; var sbElm = ElementFactory.Instance.CreateMapSurroundElement( layout, center.ToMapPoint(), sbInfo, "New Scale Bar") as ScaleBar; sbElm.SetWidth(2); sbElm.SetX(6); sbElm.SetY(7.5); });
//Create a north arrow for a specific map frame and assign a north arrow style item. //Construct on the worker thread await QueuedTask.Run(() => { //Reference a North Arrow in a style StyleProjectItem stylePrjItm = Project.Current.GetItems<StyleProjectItem>().FirstOrDefault(item => item.Name == "ArcGIS 2D"); NorthArrowStyleItem naStyleItm = stylePrjItm.SearchNorthArrows("ArcGIS North 10")[0]; //Build geometry Coordinate2D center = new Coordinate2D(7, 5.5); //Reference MF, create north arrow and add to layout MapFrame mf = layout.FindElement("New Map Frame") as MapFrame; if (mf == null) { ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show("Map frame not found", "WARNING"); return; } //At 2.x - //NorthArrow arrowElm = LayoutElementFactory.Instance.CreateNorthArrow(layout, center, mf, naStyleItm); //arrowElm.SetName("New North Arrow"); //arrowElm.SetHeight(1.75); //arrowElm.SetX(7); //arrowElm.SetY(6); var naInfo = new NorthArrowInfo() { MapFrameName = mf.Name, NorthArrowStyleItem = naStyleItm }; var arrowElm = ElementFactory.Instance.CreateMapSurroundElement( layout, center.ToMapPoint(), naInfo, "New North Arrow") as NorthArrow; arrowElm.SetHeight(1.75); arrowElm.SetX(7); arrowElm.SetY(6); });
//Set the extent of a map frame to a bookmark. //Perform on the worker thread await QueuedTask.Run(() => { //Reference MapFrame MapFrame mf_bk = layout.FindElement("Map Frame") as MapFrame; //Reference a bookmark that belongs to a map associated with the map frame Map m = mf_bk.Map; Bookmark bk = m.GetBookmarks().FirstOrDefault(item => item.Name.Equals("Lakes")); //Set the map frame extent using the bookmark mf_bk.SetCamera(bk); });
//Set the extent of a map frame to the envelope of a feature. //Perform on the worker thread await QueuedTask.Run(() => { //Reference MapFrame MapFrame mf_env = layout.FindElement("Map Frame") as MapFrame; //Get map and a layer of interest Map m = mf_env.Map; //Get the specific layer you want from the map and its extent FeatureLayer lyr = m.FindLayers("GreatLakes").First() as FeatureLayer; Envelope lyrEnv = lyr.QueryExtent(); //Set the map frame extent to the feature layer's extent / envelope mf_env.SetCamera(lyrEnv); //Note - you could have also used the lyr as an overload option });
//Added references using ArcGIS.Desktop.Core; //Project using ArcGIS.Desktop.Layouts; //Layout class using ArcGIS.Desktop.Framework.Threading.Tasks; //QueuedTask public class MapSurroundExample { public static Task<bool> UpdateMapSurroundAsync(string LayoutName, string SBName, string MFName) { //Reference a layoutitem in a project by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals(LayoutName)); if (layoutItem == null) return Task.FromResult(false); return QueuedTask.Run<bool>(() => { //Reference and load the layout associated with the layout item Layout lyt = layoutItem.GetLayout(); //Reference a scale bar element by name MapSurround scaleBar = lyt.FindElement(SBName) as MapSurround; if (scaleBar == null) return false; //Reference a map frame element by name MapFrame mf = lyt.FindElement(MFName) as MapFrame; if (mf == null) return false; //Set the scale bar to the newly referenced map frame scaleBar.SetMapFrame(mf); return true; }); } }
//Create a new table frame on the active layout. Layout layout = LayoutView.Active.Layout; //Perform on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D rec_ll = new Coordinate2D(1.0, 3.5); Coordinate2D rec_ur = new Coordinate2D(7.5, 4.5); //At 2.x - Envelope rec_env = EnvelopeBuilder.CreateEnvelope(rec_ll, rec_ur); Envelope rec_env = EnvelopeBuilderEx.CreateEnvelope(rec_ll, rec_ur); //Reference map frame MapFrame mf = layout.FindElement("Map Frame") as MapFrame; //Reference layer Map m = mf.Map; FeatureLayer lyr = m.FindLayers("GreatLakes").First() as FeatureLayer; //Build fields list var fields = new[] { "NAME", "Shape_Area", "Shape_Length" }; //Construct the table frame //At 2.x - TableFrame tabFrame = LayoutElementFactory.Instance.CreateTableFrame(layout, rec_env, mf, lyr, fields); var surroundInfo = new TableFrameInfo() { FieldNames = fields, MapFrameName = mf.Name, MapMemberUri = lyr.URI }; var tabFrame = ElementFactory.Instance.CreateMapSurroundElement(layout, rec_env, surroundInfo) as TableFrame; });
await QueuedTask.Run(() => { LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>() .FirstOrDefault(item => item.Name.Equals("Layout Name")); Layout lyt = layoutItem.GetLayout(); MapFrame mf = lyt.FindElement("Map1 Map Frame") as MapFrame; MapSurround ms = lyt.FindElement("Scale Bar") as MapSurround; ms.SetMapFrame(mf); });
//Create a map frame and set its camera by zooming to the extent of an existing bookmark. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D mf_ll = new Coordinate2D(6.0, 8.5); Coordinate2D mf_ur = new Coordinate2D(8.0, 10.5); //At 2.x - Envelope mf_env = EnvelopeBuilder.CreateEnvelope(mf_ll, mf_ur); Envelope mf_env = EnvelopeBuilderEx.CreateEnvelope(mf_ll, mf_ur); //Reference map, create MF and add to layout MapProjectItem mapPrjItem = Project.Current.GetItems<MapProjectItem>() .FirstOrDefault(item => item.Name.Equals("Map")); Map mfMap = mapPrjItem.GetMap(); Bookmark bookmark = mfMap.GetBookmarks().FirstOrDefault( b => b.Name == "Great Lakes"); //At 2.x - MapFrame mfElm = // LayoutElementFactory.Instance.CreateMapFrame( // layout, mf_env, mfMap); // mfElm.SetName("New Map Frame"); // MapFrame mfElm = ElementFactory.Instance.CreateMapFrameElement( layout, mf_env, mfMap, "New Map Frame"); //Zoom to bookmark mfElm.SetCamera(bookmark); });
//Must be on QueuedTask.Run(() => { ... //Build geometry Coordinate2D ll = new Coordinate2D(2.0, 4.5); Coordinate2D ur = new Coordinate2D(4.0, 6.5); Envelope env = EnvelopeBuilderEx.CreateEnvelope(ll, ur); //Reference map, create MF and add to layout //var map = MapView.Active.Map; //var map = mapProjectItem.GetMap(); //... MapFrame mfElm = ElementFactory.Instance.CreateMapFrameElement( layout, env, map);
//Must be on QueuedTask.Run(() => { ... //Build geometry Coordinate2D ll = new Coordinate2D(4.0, 2.5); Coordinate2D ur = new Coordinate2D(7.0, 5.5); Envelope env = EnvelopeBuilderEx.CreateEnvelope(ll, ur); //Reference map, create MF and add to layout //var map = MapView.Active.Map; //var map = mapProjectItem.GetMap(); //... MapFrame mfElm = ElementFactory.Instance.CreateMapFrameElement( layout, env.Center, map);
// This example create a new spatial map series and then applies it to the active layout. This will automatically // overwrite an existing map series if one is already present. //Reference map frame and index layer MapFrame mf = layout.FindElement("Map Frame") as MapFrame; Map m = mf.Map; BasicFeatureLayer indexLyr = m.FindLayers("Countries").FirstOrDefault() as BasicFeatureLayer; //Construct map series on worker thread await QueuedTask.Run(() => { //SpatialMapSeries constructor - required parameters SpatialMapSeries SMS = MapSeries.CreateSpatialMapSeries(layout, mf, indexLyr, "Name"); //Set optional, non-default values SMS.CategoryField = "Continent"; SMS.SortField = "Population"; SMS.ExtentOptions = ExtentFitType.BestFit; SMS.MarginType = ArcGIS.Core.CIM.UnitType.PageUnits; SMS.MarginUnits = ArcGIS.Core.Geometry.LinearUnit.Centimeters; SMS.Margin = 1; SMS.ScaleRounding = 1000; layout.SetMapSeries(SMS); //Overwrite existing map series. });
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Layouts.Element
ArcGIS.Desktop.Layouts.MapFrame
Target Platforms: Windows 11, Windows 10