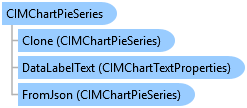
public class CIMChartPieSeries : CIMChartSeries, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMChartPieSeries Inherits CIMChartSeries Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMChartSeries
ArcGIS.Core.CIM.CIMChartPieSeries
Target Platforms: Windows 11, Windows 10