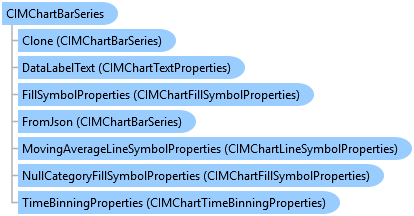
public class CIMChartBarSeries : CIMChartSeries, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMChartBarSeries Inherits CIMChartSeries Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
// For more information on the chart CIM specification: // https://github.com/Esri/cim-spec/blob/main/docs/v3/CIMCharts.md // Define fields names used in chart parameters. const string categoryField = "neighbourhood_group"; const string splitByField = "room_type"; var lyrsBar = MapView.Active.Map.GetLayersAsFlattenedList().OfType<FeatureLayer>(); var lyrBar = lyrsBar.First(); var lyrDefBar = lyrBar.GetDefinition(); // Get unique values for `splitByField` var values = new List<string>(); using (RowCursor cursor = lyrBar.Search()) { while (cursor.MoveNext()) { var value = Convert.ToString(cursor.Current[splitByField]); values.Add(value); } }; var uniqueValues = values.Distinct().ToList(); // Define bar chart CIM properties var barChart = new CIMChart { Name = "barChart", GeneralProperties = new CIMChartGeneralProperties { Title = $"{categoryField} grouped by {splitByField}", }, }; // Create list to store the info for each chart series var allSeries = new List<CIMChartSeries>(); // Create a series for each unique category foreach (var value in uniqueValues) { var series = new CIMChartBarSeries { UniqueName = value, Name = value, // Specify the category field Fields = new string[] { categoryField, string.Empty }, // Specify the WhereClause to filter a series by unique value WhereClause = $"{splitByField} = '{value}'", GroupFields = new[] { categoryField }, // Specify aggregation type FieldAggregation = new string[] { string.Empty, "COUNT" } }; allSeries.Add(series); } barChart.Series = allSeries.ToArray(); // Add new chart to layer's existing list of charts (if any exist) var newChartsBar = new CIMChart[] { barChart }; var allChartsBar = (lyrDefBar == null) ? newChartsBar : lyrDefBar.Charts.Concat(newChartsBar); // Add CIM chart to layer defintion lyrDefBar.Charts = allChartsBar.ToArray(); lyrBar.SetDefinition(lyrDefBar);
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMChartSeries
ArcGIS.Core.CIM.CIMChartBarSeries
Target Platforms: Windows 11, Windows 10