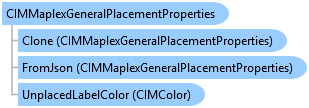
public class CIMMaplexGeneralPlacementProperties : CIMGeneralPlacementProperties, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMMaplexGeneralPlacementProperties Inherits CIMGeneralPlacementProperties Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
public void CreateFeatureLinkedAnnotationFeatureClass(Geodatabase geodatabase, SpatialReference spatialReference) { // Creating a feature-linked annotation feature class between water pipe and valve in water distribution network // with following user defined fields // PipeName // GlobalID // Annotation feature class name string annotationFeatureClassName = "WaterPipeAnnotation"; // Create user defined attribute fields for annotation feature class FieldDescription pipeGlobalID = FieldDescription.CreateGlobalIDField(); FieldDescription nameFieldDescription = FieldDescription.CreateStringField("Name", 255); // Create a list of all field descriptions List<FieldDescription> fieldDescriptions = new List<FieldDescription> { pipeGlobalID, nameFieldDescription }; // Create a ShapeDescription object ShapeDescription shapeDescription = new ShapeDescription(GeometryType.Polygon, spatialReference); // Create general placement properties for Maplex engine CIMMaplexGeneralPlacementProperties generalPlacementProperties = new CIMMaplexGeneralPlacementProperties { AllowBorderOverlap = true, PlacementQuality = MaplexQualityType.High, DrawUnplacedLabels = true, InvertedLabelTolerance = 1.0, RotateLabelWithDisplay = true, UnplacedLabelColor = new CIMRGBColor { R = 255, G = 0, B = 0, Alpha = 0.5f } }; // Create annotation label classes // Green label CIMLabelClass greenLabelClass = new CIMLabelClass { Name = "Green", ExpressionTitle = "Expression-Green", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 1, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Tahoma", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 255, 0) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Green" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Blue label CIMLabelClass blueLabelClass = new CIMLabelClass { Name = "Blue", ExpressionTitle = "Expression-Blue", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 2, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Consolas", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 0, 255) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Blue" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Create a list of labels List<CIMLabelClass> labelClasses = new List<CIMLabelClass> { greenLabelClass, blueLabelClass }; // Create linked feature description // Linked feature class name string linkedFeatureClassName = "WaterPipe"; // Create fields for water pipe FieldDescription waterPipeGlobalID = FieldDescription.CreateGlobalIDField(); FieldDescription pipeName = FieldDescription.CreateStringField("PipeName", 255); // Create a list of water pipe field descriptions List<FieldDescription> pipeFieldDescriptions = new List<FieldDescription> { waterPipeGlobalID, pipeName }; // Create a linked feature class description FeatureClassDescription linkedFeatureClassDescription = new FeatureClassDescription(linkedFeatureClassName, pipeFieldDescriptions, new ShapeDescription(GeometryType.Polyline, spatialReference)); // Create a SchemaBuilder object SchemaBuilder schemaBuilder = new SchemaBuilder(geodatabase); // Add the creation of the linked feature class to the list of DDL tasks FeatureClassToken linkedFeatureClassToken = schemaBuilder.Create(linkedFeatureClassDescription); // Create an annotation feature class description object to describe the feature class to create AnnotationFeatureClassDescription annotationFeatureClassDescription = new AnnotationFeatureClassDescription(annotationFeatureClassName, fieldDescriptions, shapeDescription, generalPlacementProperties, labelClasses, new FeatureClassDescription(linkedFeatureClassToken)) { IsAutoCreate = true, IsSymbolIDRequired = false, IsUpdatedOnShapeChange = true }; // Add the creation of the annotation feature class to the list of DDL tasks schemaBuilder.Create(annotationFeatureClassDescription); // Execute the DDL bool success = schemaBuilder.Build(); // Inspect error messages if (!success) { IReadOnlyList<string> errorMessages = schemaBuilder.ErrorMessages; //etc. } }
public static void CreateDictionary() { //Get the map's defintion var mapDefn = MapView.Active.Map.GetDefinition(); //Get the Map's Maplex labelling engine properties var mapDefnPlacementProps = mapDefn.GeneralPlacementProperties as CIMMaplexGeneralPlacementProperties; //Define the abbreaviations we need in an array List<CIMMaplexDictionaryEntry> abbreviationDictionary = new List<CIMMaplexDictionaryEntry> { new CIMMaplexDictionaryEntry { Abbreviation = "Hts", Text = "Heights", MaplexAbbreviationType = MaplexAbbreviationType.Ending }, new CIMMaplexDictionaryEntry { Abbreviation = "Ct", Text = "Text", MaplexAbbreviationType = MaplexAbbreviationType.Ending } //etc }; //The Maplex Dictionary - can hold multiple Abbreviation collections var maplexDictionary = new List<CIMMaplexDictionary> { new CIMMaplexDictionary { Name = "NameEndingsAbbreviations", MaplexDictionary = abbreviationDictionary.ToArray() } }; //Set the Maplex Label Engine Dictionary property to the Maplex Dictionary collection created above. mapDefnPlacementProps.Dictionaries = maplexDictionary.ToArray(); //Set the Map defintion MapView.Active.Map.SetDefinition(mapDefn); } private static void ApplyDictionary() { var featureLayer = MapView.Active.Map.GetLayersAsFlattenedList().OfType<FeatureLayer>().First(); QueuedTask.Run(() => { //Creates Abbreviation dictionary and adds to Map Defintion CreateDictionary(); //Get the layer's definition var lyrDefn = featureLayer.GetDefinition() as CIMFeatureLayer; //Get the label classes - we need the first one var listLabelClasses = lyrDefn.LabelClasses.ToList(); var theLabelClass = listLabelClasses.FirstOrDefault(); //Modify label Placement props to use abbreviation dictionary CIMGeneralPlacementProperties labelEngine = MapView.Active.Map.GetDefinition().GeneralPlacementProperties; theLabelClass.MaplexLabelPlacementProperties.DictionaryName = "NameEndingsAbbreviations"; theLabelClass.MaplexLabelPlacementProperties.CanAbbreviateLabel = true; theLabelClass.MaplexLabelPlacementProperties.CanStackLabel = false; //Set the labelClasses back lyrDefn.LabelClasses = listLabelClasses.ToArray(); //set the layer's definition featureLayer.SetDefinition(lyrDefn); }); }
//Note: call within QueuedTask.Run() //Get the active map's definition - CIMMap. var cimMap = MapView.Active.Map.GetDefinition(); //Get the labeling engine from the map definition var cimGeneralPlacement = cimMap.GeneralPlacementProperties; if (cimGeneralPlacement is CIMMaplexGeneralPlacementProperties) { //Current labeling engine is Maplex labeling engine //Create a new standard label engine properties var cimStandardPlacementProperties = new CIMStandardGeneralPlacementProperties(); //Set the CIMMap's GeneralPlacementProperties to the new label engine cimMap.GeneralPlacementProperties = cimStandardPlacementProperties; } else { //Current labeling engine is Standard labeling engine //Create a new Maplex label engine properties var cimMaplexGeneralPlacementProperties = new CIMMaplexGeneralPlacementProperties(); //Set the CIMMap's GeneralPlacementProperties to the new label engine cimMap.GeneralPlacementProperties = cimMaplexGeneralPlacementProperties; } //Set the map's definition MapView.Active.Map.SetDefinition(cimMap);
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMGeneralPlacementProperties
ArcGIS.Core.CIM.CIMMaplexGeneralPlacementProperties
Target Platforms: Windows 11, Windows 10