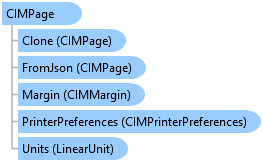
public class CIMPage : CIMObject, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMPage Inherits CIMObject Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
//Modify a layouts page settings. //Perform on the worker thread await QueuedTask.Run(() => { CIMPage page = layout.GetPage(); //Do something layout.SetPage(page); });
//This example creates a new layout using a CIM page definion with rulers and guides included. //Added references using ArcGIS.Desktop.Layouts; using ArcGIS.Desktop.Framework.Threading.Tasks; using ArcGIS.Desktop.Core; using ArcGIS.Core.CIM; public class CreateLayoutEx2 { async public static Task<Layout> CreateCIMLayout(double width, double height, LinearUnit units, string LayoutName) { Layout CIMlayout = null; await QueuedTask.Run(() => { //Set up a page CIMPage newPage = new CIMPage(); //required newPage.Width = width; newPage.Height = height; newPage.Units = units; //optional rulers newPage.ShowRulers = true; newPage.SmallestRulerDivision = 5; //optional guides newPage.ShowGuides = true; CIMGuide guide1 = new CIMGuide(); guide1.Position = 25; guide1.Orientation = Orientation.Vertical; CIMGuide guide2 = new CIMGuide(); guide2.Position = 185; guide2.Orientation = Orientation.Vertical; CIMGuide guide3 = new CIMGuide(); guide3.Position = 25; guide3.Orientation = Orientation.Horizontal; CIMGuide guide4 = new CIMGuide(); guide4.Position = 272; guide4.Orientation = Orientation.Horizontal; List<CIMGuide> guideList = new List<CIMGuide>(); guideList.Add(guide1); guideList.Add(guide2); guideList.Add(guide3); guideList.Add(guide4); newPage.Guides = guideList.ToArray(); Layout layout = LayoutFactory.Instance.CreateLayout(newPage); layout.SetName(LayoutName); }); //Open the layout in a pane on the UI! await ProApp.Panes.CreateLayoutPaneAsync(CIMlayout); return CIMlayout; } }
//Create a new layout using a modified CIM and open it. //The CIM exposes additional members that may not be //available through the managed API. //In this example, optional guides are added. //Create a new CIMLayout on the worker thread Layout newCIMLayout = await QueuedTask.Run(() => { //Set up a CIM page CIMPage newPage = new CIMPage { //required parameters Width = 8.5, Height = 11, Units = LinearUnit.Inches, //optional rulers ShowRulers = true, SmallestRulerDivision = 0.5, //optional guides ShowGuides = true }; CIMGuide guide1 = new CIMGuide { Position = 1, Orientation = Orientation.Vertical }; CIMGuide guide2 = new CIMGuide { Position = 6.5, Orientation = Orientation.Vertical }; CIMGuide guide3 = new CIMGuide { Position = 1, Orientation = Orientation.Horizontal }; CIMGuide guide4 = new CIMGuide { Position = 10, Orientation = Orientation.Horizontal }; List<CIMGuide> guideList = new List<CIMGuide> { guide1, guide2, guide3, guide4 }; newPage.Guides = guideList.ToArray(); //Construct the new layout using the customized cim definitions var layout_local = LayoutFactory.Instance.CreateLayout(newPage); layout_local.SetName("New 8.5x11 Layout"); return layout_local; }); //Open new layout on the GUI thread await ProApp.Panes.CreateLayoutPaneAsync(newCIMLayout);
//Change the layout page size. //Reference the layout project item LayoutProjectItem lytItem = Project.Current.GetItems<LayoutProjectItem>() .FirstOrDefault(item => item.Name.Equals("MyLayout")); if (layoutItem != null) { await QueuedTask.Run(() => { //Get the layout Layout lyt = lytItem.GetLayout(); if (lyt != null) { //Change properties CIMPage page = lyt.GetPage(); page.Width = 8.5; page.Height = 11; //Apply the changes to the layout lyt.SetPage(page); } }); }
//Note: Call within QueuedTask.Run() //The fields in the datasource used for the report //This uses a US Cities dataset var listFields = new List<CIMReportField> { //Grouping should be the first field new CIMReportField{Name = "STATE_NAME", FieldOrder = 0, Group = true, SortInfo = FieldSortInfo.Desc}, //Group cities using STATES new CIMReportField{Name = "CITY_NAME", FieldOrder = 1}, new CIMReportField{Name = "POP1990", FieldOrder = 2, }, }; //Definition query to use for the data source var defQuery = "STATE_NAME LIKE 'C%'"; //Define the Datasource var reportDataSource = new ReportDataSource(featureLayer, defQuery, listFields); //The CIMPage defintion - page size, units, etc var cimReportPage = new CIMPage { Height = 11, StretchElements = false, Width = 6.5, ShowRulers = true, ShowGuides = true, Margin = new CIMMargin { Bottom = 1, Left = 1, Right = 1, Top = 1 }, Units = LinearUnit.Inches }; //Report template var reportTemplates = await ReportTemplateManager.GetTemplatesAsync(); var reportTemplate = reportTemplates.Where(r => r.Name == "Attribute List with Grouping").First(); //Report Styling var reportStyles = await ReportStylingManager.GetStylingsAsync(); var reportStyle = reportStyles.Where(s => s == "Cool Tones").First(); //Field Statistics var fieldStatisticsList = new List<ReportFieldStatistic> { new ReportFieldStatistic{ Field = "POP1990", Statistic = FieldStatisticsFlag.Sum} //Note: NoStatistics option for FieldStatisticsFlag is not supported. }; var report = ReportFactory.Instance.CreateReport("USAReport", reportDataSource, cimReportPage, fieldStatisticsList, reportTemplate, reportStyle);
//Note: Call within QueuedTask.Run() var cimReportPage = new CIMPage { Height = 12, StretchElements = false, Width = 6.5, ShowRulers = true, ShowGuides = true, Margin = new CIMMargin { Bottom = 1, Left = 1, Right = 1, Top = 1 }, Units = LinearUnit.Inches }; report.SetPage(cimReportPage); //Change only the report's page height report.SetPageHeight(12);
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMPage
Target Platforms: Windows 11, Windows 10