Label | Explanation | Data Type |
Input Features | The input point, line, or polygon features to be buffered. | Feature Layer |
Output Feature class | The output feature class that will contain multiple buffers. | Feature Class |
Distances | The list of buffer distances. | Double |
Distance Unit (Optional) | Specifies the linear unit that will be used with the distance values.
| String |
Buffer Distance Field Name (Optional) | The name of the field in the output feature class that will store the buffer distance used to create each buffer feature. The default is distance. The field will be of type Double. | String |
Dissolve Option (Optional) | Specifies whether buffers will be dissolved to resemble rings around the input features.
| String |
Outside Polygons Only (Optional) | Specifies whether the buffers will cover the input features. This parameter is valid only for polygon input features.
| Boolean |
Method
(Optional) | Specifies the method used to create the buffer.
| String |
Summary
Creates multiple buffers at specified distances around the input features. These buffers can be merged and dissolved using the buffer distance values to create nonoverlapping buffers.
Illustration
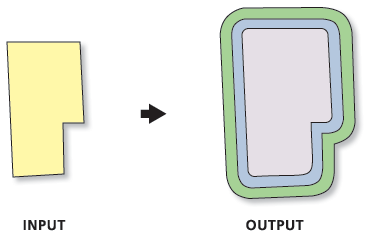
Usage
If the Input Features parameter value has a projected coordinate system, planar buffers will be produced in the output by default. If the Input Features parameter value has a geographic coordinate system, geodesic buffers will be produced in the output by default. The Method parameter can be used to change the default behavior.
If the Dissolve Option parameter's Non-overlapping (rings) option (Dissolve_Option = "ALL" in Python) is specified, the output feature class will contain one feature for each distance specified in the Distances parameter; all buffers that are the same distance from the input features will be dissolved together.
Parameters
arcpy.analysis.MultipleRingBuffer(Input_Features, Output_Feature_class, Distances, {Buffer_Unit}, {Field_Name}, {Dissolve_Option}, {Outside_Polygons_Only}, {Method})
Name | Explanation | Data Type |
Input_Features | The input point, line, or polygon features to be buffered. | Feature Layer |
Output_Feature_class | The output feature class that will contain multiple buffers. | Feature Class |
Distances [distance,...] | The list of buffer distances. | Double |
Buffer_Unit (Optional) | Specifies the linear unit that will be used with the distance values.
| String |
Field_Name (Optional) | The name of the field in the output feature class that will store the buffer distance used to create each buffer feature. The default is distance. The field will be of type Double. | String |
Dissolve_Option (Optional) | Specifies whether buffers will be dissolved to resemble rings around the input features.
| String |
Outside_Polygons_Only (Optional) | Specifies whether the buffers will cover the input features. This parameter is valid only for polygon input features.
| Boolean |
Method (Optional) | Specifies the method used to create the buffer.
| String |
Code sample
The following Python window script demonstrates how to use the MultipleRingBuffer function in immediate mode.
import arcpy
arcpy.env.workspace = "C:/data/airport.gdb"
arcpy.MultipleRingBuffer_analysis("schools", "c:/output/output.gdb/multibuffer1",
[10, 20, 30], "meters", "", "ALL")
The following stand-alone script demonstrates how to use the MultipleRingBuffer function.
# Name: MultipleRingBuffer_Example2.py
# Description: Create multiple buffers for the input features
# Import system modules
import arcpy
# Set environment settings
arcpy.env.workspace = "C:/data/airport.gdb"
# Set local variables
inFeatures = "schools"
outFeatureClass = "c:/output/output.gdb/multibuffer1"
distances = [10, 20, 30]
bufferUnit = "meters"
# Execute MultipleRingBuffer
arcpy.MultipleRingBuffer_analysis(inFeatures, outFeatureClass, distances,
bufferUnit, "", "ALL")
Environments
Licensing information
- Basic: Yes
- Standard: Yes
- Advanced: Yes