Label | Explanation | Data Type |
Output Feature Class | The path and name of the output feature class containing the tessellated grid. | Feature Class |
Extent
| The extent that the tessellation will cover. This can be the currently visible area, the extent of a dataset, or manually entered values.
| Extent |
Shape Type
(Optional) | The type of shape to tessellate.
| String |
Size
(Optional) | The area of each individual shape that comprises the tessellation. | Areal Unit |
Spatial Reference
(Optional) | The spatial reference to which the output dataset will be projected. If a spatial reference is not provided, the output will be projected to the spatial reference of the input extent. If neither has a spatial reference, the output is projected in GCS_WGS_1984. | Spatial Reference |
Summary
Generates a tessellated grid of regular polygon features to cover a given extent. The tessellation can be of triangles, squares, diamonds, hexagons, or transverse hexagons.
Illustration
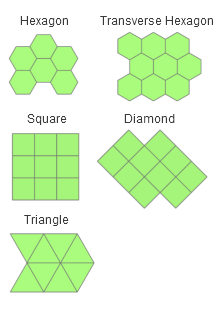
Usage
To ensure the entire input extent is covered by the tessellated grid, the output features purposely extend beyond the input extent. This occurs because the edges of the tessellated grid will not always be straight lines, and gaps would be present if the grid was limited by the input extent.
The output features contain a GRID_ID field. The GRID_ID field provides a unique ID for each feature in the output feature class. The format for the IDs is A-1, A-2, B-1, B-2, and so on. This allows for easy selection of rows and columns using queries in the Select Layer By Attribute tool. For example, select all features in column A with GRID_ID like 'A-%', or select all features in row 1 with GRID_ID like '%-1'.
- To create a grid that excludes tessellation features that do not intersect features in another dataset, use the Select Layer By Location tool to select output polygons that contain the source features, and use the Copy Features tool to make a permanent copy of the selected output features to a new feature class.
Parameters
arcpy.management.GenerateTessellation(Output_Feature_Class, Extent, {Shape_Type}, {Size}, {Spatial_Reference})
Name | Explanation | Data Type |
Output_Feature_Class | The path and name of the output feature class containing the tessellated grid. | Feature Class |
Extent | The extent that the tessellation will cover. This can be the currently visible area, the extent of a dataset, or manually entered values.
| Extent |
Shape_Type (Optional) | The type of shape to tessellate.
| String |
Size (Optional) | The area of each individual shape that comprises the tessellation. | Areal Unit |
Spatial_Reference (Optional) | The spatial reference to which the output dataset will be projected. If a spatial reference is not provided, the output will be projected to the spatial reference of the input extent. If neither has a spatial reference, the output is projected in GCS_WGS_1984. | Spatial Reference |
Code sample
The following Python window script demonstrates how to use the GenerateTesselation tool in immediate mode.
import arcpy
tessellation_extent = arcpy.Extent(0.0, 0.0, 10.0, 10.0)
spatial_ref = arcpy.SpatialReference(4326)
arcpy.GenerateTessellation_management(r"C:\data\project.gdb\hex_tessellation",
tessellation_extent, "HEXAGON",
"100 SquareMiles", spatial_ref)
The following stand-alone Python script demonstrates how to programmatically extract an extent from a feature class and use the extent to fill the parameters of the GenerateTessellation tool.
# Name: GenerateDynamicTessellation.py
# Purpose: Generate a grid of squares over the envelope of a provided feature
# class.
# Import modules
import arcpy
# Set paths of features
my_feature = r"C:\data\project.gdb\myfeature"
output_feature = r"C:\data\project.gdb\sqtessellation"
# Describe the input feature and extract the extent
description = arcpy.Describe(my_feature)
extent = description.extent
# Find the width, height, and linear unit used by the input feature class' extent
# Divide the width and height value by three.
# Multiply the divided values together and specify an area unit from the linear
# unit.
# Should result in a 4x4 grid covering the extent. (Not 3x3 since the squares
# hang over the extent.)
w = extent.width
h = extent.height
u = extent.spatialReference.linearUnitName
area = "{size} Square{unit}s".format(size=w/3 * h/3, unit=u)
# Use the extent's spatial reference to project the output
spatial_ref = extent.spatialReference
arcpy.GenerateTessellation_management(output_feature, extent, "SQUARE", area,
spatial_ref)
Environments
Licensing information
- Basic: Yes
- Standard: Yes
- Advanced: Yes