Label | Explanation | Data Type |
Input Features or Dataset | The features that will be clipped. | Feature Layer; Scene Layer; File; Building Scene Layer |
Clip Features | The features that will be used to clip the input features. | Feature Layer |
Output Features or Dataset | The dataset that will be created. | Feature Class; File |
XY Tolerance (Optional) | The minimum distance separating all feature coordinates as well as the distance a coordinate can move in x or y (or both). Set the value higher for data with less coordinate accuracy and lower for data with extremely high accuracy. Caution:Changing this parameter's value may cause failure or unexpected results. It is recommended that you do not modify this parameter. It has been removed from view on the tool dialog box. By default, the input feature class's spatial reference x,y tolerance property is used. | Linear Unit |
Summary
Extracts input features that overlay the clip features.
Use this tool to cut out a piece of one dataset using one or more of the features in another dataset as a cookie cutter. This is particularly useful for creating a new dataset—also referred to as a study area or area of interest (AOI)—that contains a geographic subset of the features in another, larger dataset.
Clip operations can also be performed using the Pairwise Clip tool.
Illustration
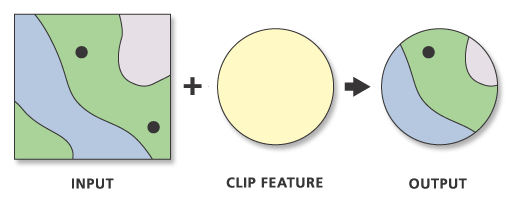
Usage
The Clip Features parameter values can be points, lines, and polygons, depending on the Input Features or Dataset parameter type.
- When the Input Features or Dataset values are polygons, the Clip Features values must also be polygons.
- When the Input Features or Dataset values are lines, the Clip Features values can be lines or polygons. When clipping line features with line features, only the coincident lines or line segments will be written to the output, as shown in the image below.
- When the Input Features or Dataset values are points, the Clip Features values can be points, lines, or polygons. When clipping point features with point features, only the coincident points will be written to the output, as shown in the image below. When clipping point features with line features, only the points that are coincident with the line features will be written to the output.
- When the Input Features or Dataset values are an integrated mesh, point, building, 3D object, or point cloud scene layer or scene layer package, the Clip Features values must be polygons.
The Output Features or Dataset parameter will contain all the attributes of the Input Features or Dataset parameter when the output is a feature class.
Integrated mesh, Point, Building and 3D object scene layer packages and scene services must use I3S version 1.6 and later to be used as input. Use the Upgrade Scene Layer tool to upgrade version 1.5 and earlier scene layer packages. The output scene layer package will be in the latest I3S version.
Scene services must have the extract capability in order to be clipped. Services without this capability will be blocked as input.
If the input is a building scene layer, all category and discipline layers will be clipped. If the input is a category layer, the output scene layer package will be a 3D object scene layer package with only the selected input layer.
For better performance and scalability, this tool uses a tiling process to handle very large datasets. For details, see Tiled processing of large datasets.
Line features clipped by polygon features:
Point features clipped by polygon features:
Line features clipped with line features:
Point features clipped with point features:
Attribute values from the input feature classes will be copied to the output feature class. However, if the input is a layer or layers created by the Make Feature Layer tool and a field's Use Ratio Policy is checked, then a ratio of the input attribute value is calculated for the output attribute value. When Use Ratio Policy is enabled, whenever a feature in an overlay operation is split, the attributes of the resulting features are a ratio of the attribute value of the input feature. The output value is based on the ratio in which the input feature geometry was divided. For example, if the input geometry was divided equally, each new feature's attribute value is assigned one-half of the value of the input feature's attribute value. Use Ratio Policy only applies to numeric field types.
Caution:
Geoprocessing tools do not honor geodatabase feature class or table field split policies.
Parameters
arcpy.analysis.Clip(in_features, clip_features, out_feature_class, {cluster_tolerance})
Name | Explanation | Data Type |
in_features | The features that will be clipped. | Feature Layer; Scene Layer; File; Building Scene Layer |
clip_features | The features that will be used to clip the input features. | Feature Layer |
out_feature_class | The dataset that will be created. | Feature Class; File |
cluster_tolerance (Optional) | The minimum distance separating all feature coordinates as well as the distance a coordinate can move in x or y (or both). Set the value higher for data with less coordinate accuracy and lower for data with extremely high accuracy. Caution:Changing this parameter's value may cause failure or unexpected results. It is recommended that you do not modify this parameter. It has been removed from view on the tool dialog box. By default, the input feature class's spatial reference x,y tolerance property is used. | Linear Unit |
Code sample
The following Python window script demonstrates how to use the Clip function in immediate mode.
import arcpy
arcpy.env.workspace = "C:/data"
arcpy.analysis.Clip("majorrds.shp", "study_quads.shp",
"C:/output/studyarea.shp")
The following Python window script demonstrates how to use the Clip function with a scene layer.
import arcpy
arcpy.env.workspace = "C:/data"
arcpy.analysis.Clip("campus.slpk", "building_footprint.shp",
"C:/output/AreaOfInterest.slpk")
The following Python script demonstrates how to use the Clip function in a stand-alone script.
# Description: Clip major roads that fall within the study area.
# Import system modules
import arcpy
# Set workspace
arcpy.env.workspace = "C:/data"
# Set local variables
in_features = "majorrds.shp"
clip_features = "study_quads.shp"
out_feature_class = "C:/output/studyarea.shp"
# Run Clip
arcpy.analysis.Clip(in_features, clip_features, out_feature_class)
The following Python script demonstrates how to use the Clip function in a stand-alone script with a scene service.
# Description: Clip a scene service.
# Import system modules
import arcpy
# Set workspace
arcpy.env.workspace = "C:/data"
# Set local variables
scene_service = "https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/2021_02_04_Frankfurt/SceneServer"
mesh_layer_name = "mesh_layer"
clip_features = "AOI.shp"
out_feature_class = "C:/output/studyarea.shp"
# Create a layer of a scene service
mesh_layer = arcpy.management.MakeSceneLayer(scene_service,
mesh_layer_name)
# Run Clip
arcpy.analysis.Clip(mesh_layer, clip_features, out_feature_class)
Environments
Special cases
- Parallel Processing Factor
This tool honors the Parallel Processing Factor environment. If the environment is not set (the default) or is set to 0, parallel processing will be disabled; parallel processing will not be used and processing will be done sequentially. Setting the environment to 100 will enable parallel processing; parallel processing will be used and processing will be done in parallel. Up to 10 cores will be used when parallel processing is enabled.
Parallel processing is currently supported for polygon-on-polygon, line-on-polygon, and point-on-polygon overlay operations.
Licensing information
- Basic: Yes
- Standard: Yes
- Advanced: Yes