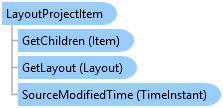
public sealed class LayoutProjectItem : ArcGIS.Desktop.Internal.Core.ProjectItem, ArcGIS.Desktop.Core.IMetadata, ArcGIS.Desktop.Core.IProjectItem, System.ComponentModel.INotifyPropertyChanged
Public NotInheritable Class LayoutProjectItem Inherits ArcGIS.Desktop.Internal.Core.ProjectItem Implements ArcGIS.Desktop.Core.IMetadata, ArcGIS.Desktop.Core.IProjectItem, System.ComponentModel.INotifyPropertyChanged
Each Layout in a project is associated with a LayoutProjectItem. This item contains numerous read-only metadata properties about the layout. Although a layout may exist in the project, it may not be loaded (an open layout view). To reference the actual layout and ensure it is loaded into memory, you must use the GetLayout method.
//Reference layout project items and their associated layout. //A layout project item is an item that appears in the Layouts folder in the Catalog pane. //Reference all the layout project items IEnumerable<LayoutProjectItem> layouts = Project.Current.GetItems<LayoutProjectItem>(); //Or reference a specific layout project item by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("MyLayout"));
//Open a layout project item in a new view. //A layout project item may exist but it may not be open in a view. //Reference a layout project item by name LayoutProjectItem someLytItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("MyLayout")); //Get the layout associated with the layout project item Layout layout = await QueuedTask.Run(() => someLytItem.GetLayout()); //Worker thread //Create the new pane ILayoutPane iNewLayoutPane = await ProApp.Panes.CreateLayoutPaneAsync(layout); //GUI thread
//Import a pagx into a project. //Create a layout project item from importing a pagx file IProjectItem pagx = ItemFactory.Instance.Create(@"C:\Temp\Layout.pagx") as IProjectItem; Project.Current.AddItem(pagx);
//This example deletes a layout in a project after finding it by name. //Added references using ArcGIS.Desktop.Core; using ArcGIS.Desktop.Layouts; public class DeleteLayoutExample { public static Task<bool> DeleteLayoutAsync(string LayoutName) { //Reference a layoutitem in a project by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals(LayoutName)); //Check for layoutItem if (layoutItem == null) return Task.FromResult<bool>(false); //Delete the layout from the project return Task.FromResult<bool>(Project.Current.RemoveItem(layoutItem)); } }
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Core.Item
ArcGIS.Desktop.Layouts.LayoutProjectItem
Target Platforms: Windows 10, Windows 8.1, Windows 7