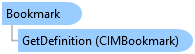
public sealed class Bookmark : ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase, System.ComponentModel.INotifyPropertyChanged
Public NotInheritable Class Bookmark Inherits ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase Implements System.ComponentModel.INotifyPropertyChanged
Bookmarks can be spatial (2D or 3D) and temporal. If your map is time-enabled, bookmarks can be created for a specific point in time. Bookmarks in an ArcGIS Pro project are associated and managed with the map they were created in. Bookmarks are transferable, so you can re-use bookmarks between multiple maps and scenes in your project, as well as with or without temporal information.
You can call the Map.GetBookmarks method on the Map to return a collection of bookmarks for the map. An similar extension method, ArcGIS.Desktop.Core.ProjectExtender.GetBookmarks, is available off of Project which will return all the bookmarks in the Project. You can use the bookmark to navigate the view by calling the MapView.ZoomTo or MapView.PanTo methods on the MapView.
<!-- Copyright 2018 Esri Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License. --> <UserControl x:Class="Examples.BookmarkDockPane" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mapping="clr-namespace:ArcGIS.Desktop.Mapping;assembly=ArcGIS.Desktop.Mapping" mc:Ignorable="d" d:DesignHeight="300" d:DesignWidth="300"> <UserControl.Resources> <ResourceDictionary> <ResourceDictionary.MergedDictionaries> <ResourceDictionary Source="pack://application:,,,/ArcGIS.Desktop.Framework;component\Themes\Default.xaml"/> </ResourceDictionary.MergedDictionaries> <DataTemplate x:Key="BookmarkListItem" DataType="{x:Type mapping:Bookmark}"> <StackPanel Orientation="Vertical" Margin="0" Background="Transparent" > <Grid> <Image Source="{Binding Thumbnail}" MaxHeight="96" MaxWidth="96" Margin="5"> <Image.Effect> <DropShadowEffect Color="#FF565454" Opacity="0.4" /> </Image.Effect> </Image> <Image Source="pack://application:,,,/ArcGIS.Desktop.Resources;component/Images/GenericTime16.png" Margin="5" MaxHeight="16" MaxWidth="16" VerticalAlignment="Bottom" HorizontalAlignment="Left"> <Image.Style> <Style TargetType="Image"> <Style.Triggers> <DataTrigger Binding="{Binding HasTimeExtent}" Value="False"> <Setter Property="Visibility" Value="Collapsed"/> </DataTrigger> </Style.Triggers> </Style> </Image.Style> </Image> </Grid> <TextBlock Text="{Binding Name}" ToolTip="{Binding Name}" HorizontalAlignment="Center" TextAlignment="Center" TextWrapping="NoWrap" TextTrimming="CharacterEllipsis" MaxWidth="96" Margin="0,1"/> </StackPanel> </DataTemplate> </ResourceDictionary> </UserControl.Resources> <Grid> <ListBox Margin="0" ScrollViewer.VerticalScrollBarVisibility="Auto" ScrollViewer.HorizontalScrollBarVisibility="Disabled" ItemsSource="{Binding Bookmarks}" SelectedItem="{Binding SelectedBookmark}" ItemTemplate="{StaticResource BookmarkListItem}"> <ListBox.ItemsPanel> <ItemsPanelTemplate> <WrapPanel Orientation="Horizontal" /> </ItemsPanelTemplate> </ListBox.ItemsPanel> <ListBox.ItemContainerStyle> <Style TargetType="ListBoxItem"> <Setter Property="HorizontalAlignment" Value="Center"/> <Setter Property="VerticalAlignment" Value="Center" /> <EventSetter Event="MouseDoubleClick" Handler="ListBoxItem_MouseDoubleClick" /> </Style> </ListBox.ItemContainerStyle> </ListBox> </Grid> </UserControl>
/* Copyright 2018 Esri Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License. */ using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Navigation; using System.Windows.Shapes; namespace Examples { /// <summary> /// Interaction logic for BookmarksView.xaml. /// </summary> public partial class BookmarkDockPane : UserControl { public BookmarkDockPane() { InitializeComponent(); } /// <summary> /// Event handler for list box item double-click. /// </summary> public void ListBoxItem_MouseDoubleClick(object sender, RoutedEventArgs e) { var vm = this.DataContext as BookmarkDockPaneViewModel; if (vm == null) return; vm.ZoomToSelectedBookmarkAsync(); } } }
/* Copyright 2018 Esri Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License. */ using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using ArcGIS.Desktop.Framework; using ArcGIS.Desktop.Framework.Contracts; using System.Collections.ObjectModel; using ArcGIS.Desktop.Mapping; using ArcGIS.Desktop.Core.Events; using ArcGIS.Desktop.Core; using ArcGIS.Desktop.Framework.Threading.Tasks; namespace Examples { /// <summary> /// ViewModel for the Bookmarks DockPane. /// </summary> internal class BookmarkDockPaneViewModel : DockPane { /// <summary> /// Subscribe to the ProjectOpenedEvent when the DockPane is created. /// </summary> protected BookmarkDockPaneViewModel() { ProjectOpenedEvent.Subscribe(OnProjectOpened); } /// <summary> /// Unsubscribe from the ProjectOpenedEvent when the DockPane is destroyed. /// </summary> ~BookmarkDockPaneViewModel() { ProjectOpenedEvent.Unsubscribe(OnProjectOpened); } /// <summary> /// Called by the framework when the DockPane initializes. /// </summary> protected override Task InitializeAsync() { return UpdateBookmarksAsync(); } /// <summary> /// Bindable property for the collection of bookmarks for the project. /// </summary> private ReadOnlyObservableCollection<Bookmark> _bookmarks; public ReadOnlyObservableCollection<Bookmark> Bookmarks { get { return _bookmarks; } } /// <summary> /// Bindable property for the currently selected bookmark. /// </summary> private Bookmark _selectedBookmark; public Bookmark SelectedBookmark { get { return _selectedBookmark; } set { SetProperty(ref _selectedBookmark, value, () => SelectedBookmark); } } /// <summary> /// Zoom the active map view to the selected bookmark. /// </summary> /// <returns>True if the navigation completes successfully.</returns> internal Task<bool> ZoomToSelectedBookmarkAsync() { if (MapView.Active == null) return Task.FromResult(false); return MapView.Active.ZoomToAsync(SelectedBookmark, TimeSpan.FromSeconds(1.5)); } /// <summary> /// Updates the collection of bookmarks which is called when the DockPane is initialized or a project is opened. /// </summary> private async Task UpdateBookmarksAsync() { _bookmarks = await QueuedTask.Run(() => Project.Current.GetBookmarks()); NotifyPropertyChanged(() => Bookmarks); } /// <summary> /// Event delegate for the ProjectOpenedEvent /// </summary> private void OnProjectOpened(ProjectEventArgs obj) { Task t = UpdateBookmarksAsync(); } } }
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Mapping.Bookmark
Target Platforms: Windows 10, Windows 8.1, Windows 7