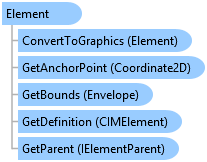
public abstract class Element : ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase, System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable<Element>
Public MustInherit Class Element Inherits ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase Implements System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable(Of Element)
//Find an element on a layout. // Reference a layout project item by name LayoutProjectItem layoutItem = Project.Current.GetItems<LayoutProjectItem>().FirstOrDefault(item => item.Name.Equals("MyLayout")); if (layoutItem != null) { QueuedTask.Run(() => { // Reference and load the layout associated with the layout item Layout mylayout = layoutItem.GetLayout(); if (layout != null) { //Find a single specific element Element rect = mylayout.FindElement("Rectangle") as Element; //Or use the Elements collection Element rect2 = mylayout.Elements.FirstOrDefault(item => item.Name.Equals("Rectangle")); } }); }
//Update an element's transparency using the CIM. //Perform on the worker thread QueuedTask.Run(() => { // Reference and load the layout associated with the layout item Layout layout = layoutItem.GetLayout(); if (layout != null) { // Reference a element by name GraphicElement graphicElement = layout.FindElement("MyElement") as GraphicElement; if (graphicElement != null) { // Modify the Transparency property that exists only in the CIMGraphic class. CIMGraphic CIMGraphic = graphicElement.GetGraphic() as CIMGraphic; CIMGraphic.Transparency = 50; // mark it 50% transparent graphicElement.SetGraphic(CIMGraphic); } } });
//Clone a layout graphic element and apply an offset. //Perform on the worker thread QueuedTask.Run(() => { // Reference and load the layout associated with the layout item Layout layout = layoutItem.GetLayout(); if (layout != null) { // Reference a graphic element by name GraphicElement graphicElement = layout.FindElement("MyElement") as GraphicElement; if (graphicElement != null) { //Clone and set the new x,y GraphicElement cloneElement = graphicElement.Clone("Clone"); cloneElement.SetX(cloneElement.GetX() + xOffset); cloneElement.SetY(cloneElement.GetY() + yOffset); } } });
//Get element's selection count. //Count the number of selected elements on the active layout view LayoutView activeLayoutView = LayoutView.Active; if (activeLayoutView != null) { var selectedElements = activeLayoutView.GetSelectedElements(); ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show($@"Selected elements: {selectedElements.Count}"); }
//Set the active layout view's selection to include 2 rectangle elements. //Reference the active view LayoutView activeLayoutView = LayoutView.Active; if (activeLayoutView != null) { //Perform on the worker thread QueuedTask.Run(() => { //Reference the layout Layout lyt = activeLayoutView.Layout; //Reference the two rectangle elements Element rec = lyt.FindElement("Rectangle"); Element rec2 = lyt.FindElement("Rectangle 2"); //Construct a list and add the elements List<Element> elmList = new List<Element> { rec, rec2 }; //Set the selection activeLayoutView.SelectElements(elmList); }); }
//Clear the layout selection.
layout.ClearElementSelection();
//Delete an element or elements on a layout. //Perform on the worker thread QueuedTask.Run(() => { //Delete a specific element on a layout aLayout.DeleteElement(elm); //Or delete a group of elements using a filter aLayout.DeleteElements(item => item.Name.Contains("Clone")); //Or delete all elements on a layout aLayout.DeleteElements(item => true); });
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Layouts.Element
ArcGIS.Desktop.Layouts.AttachmentFrame
ArcGIS.Desktop.Layouts.GraphicElement
ArcGIS.Desktop.Layouts.GroupElement
ArcGIS.Desktop.Layouts.MapFrame
ArcGIS.Desktop.Layouts.MapSurround
Target Platforms: Windows 10, Windows 8.1