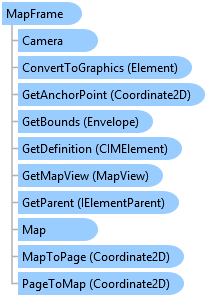
public sealed class MapFrame : Element, System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable<Element>
Public NotInheritable Class MapFrame Inherits Element Implements System.ComponentModel.INotifyPropertyChanged, System.IComparable, System.IEquatable(Of Element)
The MapFrame class primarily manages the placement of maps and scenes on a page layout. You can use Map and SetMap members to get and set the Map associated with a MapFrame.
The Export method allows you to export only the contents of a map frame instead of, for example, exporting an entire page layout.
If you want to change the geographic extent within a map frame, you can use the Camera and SetCamera members to get and set the Camera associated with a MapFrame. SetCamera is an overloaded method that allows you to change the map frame extent to a camera position, a bookmark location, and so on.
//Create a map frame and set its camera by zooming to the extent of an existing bookmark. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D mf_ll = new Coordinate2D(6.0, 8.5); Coordinate2D mf_ur = new Coordinate2D(8.0, 10.5); Envelope mf_env = EnvelopeBuilder.CreateEnvelope(mf_ll, mf_ur); //Reference map, create MF and add to layout MapProjectItem mapPrjItem = Project.Current.GetItems<MapProjectItem>().FirstOrDefault(item => item.Name.Equals("Map")); Map mfMap = mapPrjItem.GetMap(); MapFrame mfElm = LayoutElementFactory.Instance.CreateMapFrame(layout, mf_env, mfMap); mfElm.SetName("New Map Frame"); //Zoom to bookmark Bookmark bookmark = mfElm.Map.GetBookmarks().FirstOrDefault(b => b.Name == "Great Lakes"); mfElm.SetCamera(bookmark); });
//Apply a background color to the map frame element using the CIM. //Perform on the worker thread await QueuedTask.Run(() => { //Get the layout var myLayout = Project.Current.GetItems<LayoutProjectItem>()?.First().GetLayout(); if (myLayout == null) return; //Get the map frame in the layout MapFrame mapFrame = myLayout.FindElement("New Map Frame") as MapFrame; if (mapFrame == null) { ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show("Map frame not found", "WARNING"); return; } //Get the map frame's definition in order to modify the background. var mapFrameDefn = mapFrame.GetDefinition() as CIMMapFrame; //Construct the polygon symbol to use to create a background var polySymbol = SymbolFactory.Instance.ConstructPolygonSymbol(ColorFactory.Instance.BlueRGB, SimpleFillStyle.Solid); //Set the background mapFrameDefn.GraphicFrame.BackgroundSymbol = polySymbol.MakeSymbolReference(); //Set the map frame definition mapFrame.SetDefinition(mapFrameDefn); });
//Create a legend for an associated map frame. //Construct on the worker thread await QueuedTask.Run(() => { //Build 2D envelope geometry Coordinate2D leg_ll = new Coordinate2D(6, 2.5); Coordinate2D leg_ur = new Coordinate2D(8, 4.5); Envelope leg_env = EnvelopeBuilder.CreateEnvelope(leg_ll, leg_ur); //Reference MF, create legend and add to layout MapFrame mapFrame = layout.FindElement("New Map Frame") as MapFrame; if (mapFrame == null) { ArcGIS.Desktop.Framework.Dialogs.MessageBox.Show("Map frame not found", "WARNING"); return; } Legend legendElm = LayoutElementFactory.Instance.CreateLegend(layout, leg_env, mapFrame); legendElm.SetName("New Legend"); });
//Change a map frame's camera settings. //Perform on the worker thread await QueuedTask.Run(() => { //Reference MapFrame MapFrame mf = layout.FindElement("Map Frame") as MapFrame; //Reference the camera associated with the map frame and change the scale Camera cam = mf.Camera; cam.Scale = 100000; //Set the map frame extent based on the new camera info mf.SetCamera(cam); });
//Zoom map frame to the extent of a single layer. //Perform on the worker thread await QueuedTask.Run(() => { //Reference MapFrame MapFrame mf = layout.FindElement("Map Frame") as MapFrame; //Reference map and layer Map m = mf.Map; FeatureLayer lyr = m.FindLayers("GreatLakes").First() as FeatureLayer; //Set the map frame extent to all features in the layer mf.SetCamera(lyr, false); });
//Change the extent of a map frame to the selected features multiple layers. //Perform on the worker thread await QueuedTask.Run(() => { //Reference MapFrame MapFrame mf = layout.FindElement("Map Frame") as MapFrame; //Reference map, layers and create layer list Map m = mf.Map; FeatureLayer fl_1 = m.FindLayers("GreatLakes").First() as FeatureLayer; FeatureLayer fl_2 = m.FindLayers("States_WithRegions").First() as FeatureLayer; var layers = new[] { fl_1, fl_2 }; //IEnumerable<Layer> layers = m.Layers; //This creates a list of ALL layers in map. //Set the map frame extent to the selected features in the list of layers mf.SetCamera(layers, true); });
//Export a map frame to JPG. //Create JPEG format with appropriate settings //BMP, EMF, EPS, GIF, PDF, PNG, SVG, TGA, and TFF formats are also available for export JPEGFormat JPG = new JPEGFormat() { HasWorldFile = true, Resolution = 300, OutputFileName = filePath, JPEGColorMode = JPEGColorMode.TwentyFourBitTrueColor, Height = 800, Width = 1200 }; //Reference the map frame MapFrame mf = layout.FindElement("MyMapFrame") as MapFrame; //Export on the worker thread await QueuedTask.Run(() => { //Check to see if the path is valid and export if (JPG.ValidateOutputFilePath()) { mf.Export(JPG); //Export the map frame to JPG } });
System.Object
ArcGIS.Desktop.Framework.Contracts.PropertyChangedBase
ArcGIS.Desktop.Layouts.Element
ArcGIS.Desktop.Layouts.MapFrame
Target Platforms: Windows 10, Windows 8.1