Summary
The Chart class defines an ArcGIS Pro chart. The class allows you to create various types of charts, including bar charts, line charts, scatterplots, scatterplot matrices, QQ plots, histograms, box plots, and data clocks. The class can also be used to define the chart title, axes, and other properties.
Learn more about charts in ArcGIS Pro
Legacy:
The Chart class is a parent for additional subclasses in the arcpy.charts module. The subclasses were designed as a more convenient and intuitive alternative to the Chart class. As of ArcGIS Pro 2.9, the Chart class is deprecated and will not be updated for new functionality. It is recommended that you use the subclasses in the arcpy.charts module instead.
Discussion
Charts assist in presenting information about map features and the relationship between them in a visual manner. Charts can also be created for nonspatial tables and can show additional information about the features on the map or the same information in a different way. Charts are complementary to maps in that they visually convey information that would generally be summarized numerically or explored from tables. With a chart, you can quickly compare features to gain insight into the functional relationship between the features and thereby visualize the distribution, trends, and patterns in the data that would otherwise be difficult to see.
Charts can be created using a Chart object and added to a layer or table in an ArcGIS Pro project. To add a chart to a layer in a project, create a Chart object, configure its properties, and associate the Chart object with an arcpy.mp.Layer from an arcpy.mp.ArcGISProject. A chart made in this way can be opened from the Charts section under the layer in the Contents pane when that project is opened in ArcGIS Pro.
The Chart object has a dataSource property that you can use to create a chart for data sources, including various feature class and table formats and web layers (feature services). A chart can be exported to an .svg file for visualization. Chart objects support rich representation in Notebooks and can be visualized graphically.
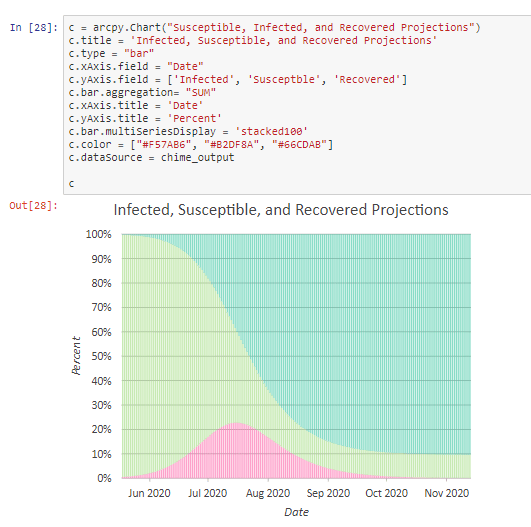
Syntax
Chart (name)
Parameter | Explanation | Data Type |
name | The name of the chart. A chart must have a unique name per layer. This name is only used for identification; it is not displayed. | String |
Properties
Property | Explanation | Data Type |
bar (Read and Write) | Sets additional properties that apply to bar charts.
| Object |
boxPlot (Read and Write) | Sets additional properties that apply to box plots.
| Object |
calendarHeatChart (Read and Write) | Sets additional properties that apply to calendar heat charts.
| Object |
dataClock (Read and Write) | Sets additional properties that apply to data clocks.
| Object |
dataSource (Read and Write) | Sets the data source of the chart. When a chart is exported using the exportToSVG method or displayed in an ArcGIS Notebook, the data source is read and rendered on the chart. Valid data sources include paths to datasets, including local datasets, UNC paths, and service URLs, and arcpy.mp Layer objects. | String |
description (Read and Write) | Sets the description of the chart. The description text appears at the bottom of the chart view. | String |
displaySize (Read and Write) | Sets the size of the chart when exported using the exportToSVG method or displayed in an ArcGIS Notebook. The value must be specified as a two-item list, where the first item is the width of the chart and the second item is the height of the chart. | List |
histogram (Read and Write) | Sets additional properties that apply to histograms.
| Object |
legend (Read and Write) | Sets properties of the chart legend.
| Object |
line (Read and Write) | Sets additional properties that apply to line charts.
| Object |
matrixHeatChart (Read and Write) | Sets additional properties that apply to matrix heat charts.
| Object |
qqPlot (Read and Write) | Sets additional properties that apply to QQ plots.
| Object |
scatter (Read and Write) | Sets additional properties that apply to scatter plots.
| Object |
scatterMatrix (Read and Write) | Sets additional properties that apply to scatter plot matrices.
| Object |
title (Read and Write) | Sets the title of the chart. The title text appears at the top of the chart view and is used as the label in the Contents pane on the List By Drawing Order tab | String |
type (Read and Write) | Sets the type of chart to create. Valid options include the following:
| String |
xAxis (Read and Write) | Sets properties of the x-axis.
| Object |
yAxis (Read and Write) | Sets properties of the y-axis.
| Object |
Method Overview
Method | Explanation |
addToLayer (layer_or_layerfile) | Adds the chart object to a layer or stand-alone table. |
exportToSVG (path, width, height) | Exports the chart to SVG format. |
updateChart () | Updates chart properties to sync changes between the object and the chart previously added to a layer. |
Methods
addToLayer (layer_or_layerfile)
Parameter | Explanation | Data Type |
layer_or_layerfile | The chart will be added to the target object. The layer_or_layerfile argument can be a Layer or a Table object. | Object |
Often the final step after defining chart properties is to add the chart object to a layer or table using the addToLayer method.
Add a chart to an existing layer.
import arcpy
aprx = arcpy.mp.ArcGISProject("current")
map = aprx.listMaps()[0]
censusLayer = map.listLayers('Census Block Groups')[0]
# Add chart object to a layer
chart.addToLayer(censusLayer)
exportToSVG (path, width, height)
Parameter | Explanation | Data Type |
path | The path where the chart will be exported in SVG format. | String |
width | The width of the output graphic. | Integer |
height | The height of the output graphic. | Integer |
In some cases, you may want to save the chart as a graphic that can be shared and viewed outside of ArcGIS Pro. Exporting to the SVG graphic format is beneficial, as the chart elements and text are stored as vector elements that can be independently modified in a vector graphics software. An SVG graphic can also be resized to any scale without pixelation or loss in quality.
Export a chart that has a project layer data source to an .svg file.
import arcpy
aprx = arcpy.mp.ArcGISProject('current')
censusLayer = aprx.listMaps()[0].listLayers('Census Block Groups')[0]
# Set data source of chart object to a layer within current project
chart.dataSource = censusLayer
# Save the chart to file with dimensions width=500, height=500
chart.exportToSVG('populationByState.svg', 500, 500)
Export a chart that has a feature service data source to an .svg file.
featureServiceURL = r'https://services1.arcgis.com/hLJbHVT9ZrDIzK0I/arcgis/rest/services/CrimesChiTheft/FeatureServer/0'
# Set data source of chart object to a feature service URL
chart.dataSource = featureServiceURL
# Save the chart to file with dimensions width=800, height=600
chart.exportToSVG('theftsPerBeat.svg', 800, 600)
updateChart ()
Often the final step after defining chart properties is to add the chart object to a layer using the addToLayer method.
To further modify the chart properties, you can modify the properties of the original chart instead of starting from scratch with a new chart. You can then use the updateChart method to synchronize any changes into the chart that was added to the layer. This will allow the changes you make to be presented in the Chart properties pane and chart view.
Use the updateChart method to synchronize chart property changes into a layer.
chart.addToLayer(myLayer)
# Further modification is necessary
chart.description = "Data from the U.S. Census Bureau"
chart.updateChart()
Code sample
Add a Chart object to an arcpy.mp Layer object to add the chart to the layer.
import arcpy
aprx = arcpy.mp.ArcGISProject("current")
map = aprx.listMaps()[0]
censusLayer = map.listLayers('Census Block Groups')[0]
chart = arcpy.Chart('MyChart')
chart.type = 'scatter'
chart.title = 'Relationship between Percent Vacant (Housing) and Population Density'
chart.description = 'This chart examines the relationship between housing vacancy and population density.'
chart.xAxis.field = 'Per_Vacant'
chart.yAxis.field = 'Pop_Density'
chart.xAxis.title = 'Vacant Housing %'
chart.yAxis.title = 'Population Density (per Sq. Mile)'
chart.addToLayer(censusLayer)
Create and export a chart based on values in a list. The example demonstrates how the list can be written to disk as a CSV file, and this file can then be used to create the chart by setting the dataSource property.
import arcpy
import csv
temp_csv_file = r'c:\temp\data.csv'
out_svg_file = r'c:\temp\chart.svg'
# Data for automobile miles per gallon (MPG) and horsepower
columns = ['mpg', 'horsepower']
data = [
[18, 130],
[15, 165],
[26, 113],
[18, 150],
[28, 90],
[32, 61],
[16, 150],
[17, 140]
]
# Write this list to a .csv file
with open(temp_csv_file, 'w', newline='') as csvfile:
writer = csv.writer(csvfile, delimiter=',')
# Write column headers
writer.writerow(columns)
# Write data rows
writer.writerows(data)
chart = arcpy.Chart('MyChart')
chart.type = 'scatter'
chart.title = 'Relationship between MPG and Horsepower'
chart.xAxis.field = 'mpg'
chart.yAxis.field = 'horsepower'
chart.dataSource = temp_csv_file
chart.exportToSVG(out_svg_file, width=750, height=400)