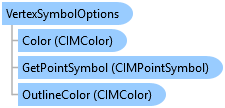
//Custom tools have the ability to change the symbology used when sketching a new feature. //Both the Sketch Segment Symbol and the Vertex Symbol can be modified using the correct set method. //This is set in the activate method for the tool. protected override Task OnToolActivateAsync(bool active) { QueuedTask.Run(() => { //Getting the current symbology options of the segment var segmentOptions = GetSketchSegmentSymbolOptions(); //Modifying the primary and secondary color and the width of the segment symbology options var deepPurple = new CIMRGBColor() { R = 75, G = 0, B = 110 }; segmentOptions.PrimaryColor = deepPurple; segmentOptions.Width = 4; segmentOptions.HasSecondaryColor = true; var pink = new CIMRGBColor() { R = 219, G = 48, B = 130 }; segmentOptions.SecondaryColor = pink; //Creating a new vertex symbol options instance with the values you want var vertexOptions = new VertexSymbolOptions(VertexSymbolType.RegularUnselected); var yellow = new CIMRGBColor() { R = 255, G = 215, B = 0 }; var purple = new CIMRGBColor() { R = 148, G = 0, B = 211 }; vertexOptions.AngleRotation = 45; vertexOptions.Color = yellow; vertexOptions.MarkerType = VertexMarkerType.Star; vertexOptions.OutlineColor = purple; vertexOptions.OutlineWidth = 3; vertexOptions.Size = 5; //Setting the value of the segment symbol options SetSketchSegmentSymbolOptions(segmentOptions); //Setting the value of the vertex symbol options of the regular unselected vertices using the vertexOptions instance created above. SetSketchVertexSymbolOptions(VertexSymbolType.RegularUnselected, vertexOptions); }); return base.OnToolActivateAsync(active); }
var options = ApplicationOptions.EditingOptions; //Must use QueuedTask QueuedTask.Run(() => { //There are 4 vertex symbol settings - selected, unselected and the //current vertex selected and unselected. var reg_select = options.GetVertexSymbolOptions(VertexSymbolType.RegularSelected); var reg_unsel = options.GetVertexSymbolOptions(VertexSymbolType.RegularUnselected); var curr_sel = options.GetVertexSymbolOptions(VertexSymbolType.CurrentSelected); var curr_unsel = options.GetVertexSymbolOptions(VertexSymbolType.CurrentUnselected); //to convert the options to a symbol use //GetPointSymbol var reg_sel_pt_symbol = reg_select.GetPointSymbol(); //ditto for reg_unsel, curr_sel, curr_unsel });
//var options = ApplicationOptions.EditingOptions; QueuedTask.Run(() => { //change the regular unselected vertex symbol //default is a green, hollow, square, 5pts. Change to //Blue outline diamond, 10 pts var vertexSymbol = new VertexSymbolOptions(VertexSymbolType.RegularUnselected); vertexSymbol.OutlineColor = ColorFactory.Instance.BlueRGB; vertexSymbol.MarkerType = VertexMarkerType.Diamond; vertexSymbol.Size = 10; //Are these valid? if (options.CanSetVertexSymbolOptions( VertexSymbolType.RegularUnselected, vertexSymbol)) { //apply them options.SetVertexSymbolOptions(VertexSymbolType.RegularUnselected, vertexSymbol); } });
//var options = ApplicationOptions.EditingOptions; QueuedTask.Run(() => { //ditto for reg selected and current selected, unselected var def_reg_unsel = options.GetDefaultVertexSymbolOptions(VertexSymbolType.RegularUnselected); //apply default options.SetVertexSymbolOptions( VertexSymbolType.RegularUnselected, def_reg_unsel); });
System.Object
ArcGIS.Desktop.Core.VertexSymbolOptions
Target Platforms: Windows 11, Windows 10