摘要
建立对栅格或栅格列表中所有像素块的只读访问。
说明
PixelBlockCollection 对象是栅格或栅格列表中所有 PixelBlock 对象的迭代器。如果处理后的栅格过大,无法加载到内存中,则可使用该对象逐块执行自定义栅格处理。
PixelBlockCollection 类支持将单个栅格或栅格列表作为输入。如果输入为单个栅格,则输出将具有两个维度(沿 y 轴的像素块数,沿 x 轴的像素块数)。例如,要访问输入栅格中的第一个像素块,请使用以下命令:
pixelblockcoll = PixelBlockCollection(in_raster)
numpy_array = pixelblockcoll[0][0].getData()
如果输入为栅格列表,则输出将具有三个维度(沿 y 轴的像素块数,沿 x 轴的像素块数,输入栅格数)。例如,要访问列表中第二个栅格中的第一个像素块,请使用以下命令:
pixelblockcoll = PixelBlockCollection(in_raster1, in_raster2) numpy_array = pixelblockcoll[0][0][1].getData()
PixelBlockCollection 类的 stride 参数指定从内存中的一行像素到内存中的下一行像素的字节数。这也称为增量、仰俯角或跨度大小。下图显示了当步幅设置为默认值(大小与像素块大小相同)时,PixelBlockCollection 对象将如何遍历像素:
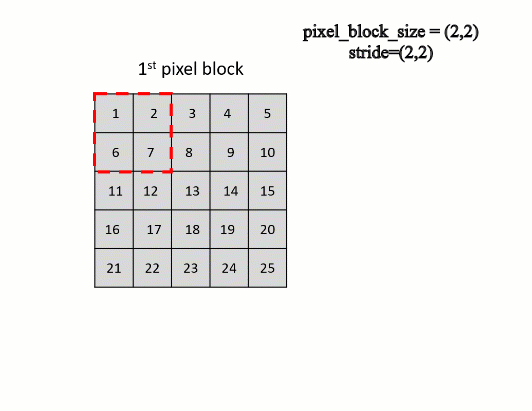
下图显示了当步幅设置为 (2, 1) 时,PixelBlockCollection 对象将如何遍历像素:
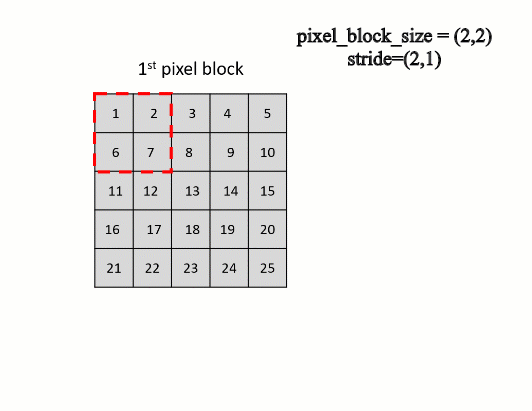
语法
PixelBlockCollection (in_rasters, pixel_block_size, stride, overlay_type, nodata_to_values)
参数 | 说明 | 数据类型 |
in_rasters [in_rasters,...] | 输入栅格对象或栅格对象列表。如果您使用的是栅格对象列表,则所有栅格必须具有相同的像元大小和空间参考。 | Raster |
pixel_block_size | 包含两个用于描述块大小(行数、列数)的值的元组。 (默认值为 (512, 512)) | tuple |
stride | 包含两个可沿要处理的行和列指定步幅或增量的整数的元组。 如果未指定任何值,则将使用像素块大小且相邻像素块将不发生重叠。 (默认值为 None) | tuple |
overlay_type | 指定输入栅格具有不同范围时的叠加类型。
(默认值为 INTERSECTION) | String |
nodata_to_values [nodata_to_values,...] | 从生成的 NumPy 数组中的输入栅格分配至 NoData 值的值。该值可以是与每个栅格相对应的单个值或一组值。 | Integer |
属性
属性 | 说明 | 数据类型 |
size (只读) | 像素块集合中沿 y 方向和 x 方向的像素块数。y 方向上的像素块数将位于第一个位置。 | tuple |
方法概述
方法 | 说明 |
reset () | 将像素块集合迭代器重置到集合的开头。 |
shuffle () | 打乱像素块集合中的像素块顺序。将图像样本输入到深度学习模型时,此选项能起到很大作用。 |
方法
代码示例
遍历像素块以计算土地覆被栅格中的总城区面积。
import arcpy
# Specify the input raster
in_raster1 = arcpy.Raster("landcover.tif")
# Create a PixelBlockCollection
blockCollection = arcpy.ia.PixelBlockCollection(
in_raster1, pixel_block_size = (512, 512), nodata_to_values = -1)
# Check the number of pixelblocks along x and y direction
number_blocks_y, number_blocks_x= blockCollection.size
urban_cell_count=0
# Iterate through each PixelBlock
for i in range(number_blocks_y):
for j in range(number_blocks_x):
pixelblock = blockCollection[i,j]
np_array = pixelblock.getData()
urban_cell_count+= np.count_nonzero(np_array == 3)
# value = 3 is urban class
urban_area = urban_cell_count * in_raster1.meanCellWidth*in_raster1.meanCellHeight
print("Total urban area : " + str(urban_area))
遍历像素块以计算在 2006 年到 2016 年间从森林转变为城市的总面积。
import arcpy
from arcpy.ia import *
# Specify the input rasters
in_raster1 = arcpy.Raster("C:/iapyexamples/data/landcover_2006.tif")
in_raster2 = arcpy.Raster("C:/iapyexamples/data/landcover_2016.tif")
# Create a PixelBlockCollection
blockCollection = arcpy.ia.PixelBlockCollection(
[in_raster1, in_raster2], pixel_block_size = (256, 256), nodata_to_values = -1)
# Check the number of pixelblocks along x and y direction
number_blocks_y, number_blocks_x= pixelblocks.size
forest_to_urban_cell_count = 0
# Iterate through each PixelBlock
for i in range(number_blocks_y):
for j in range(number_blocks_x):
pixelblocklist = blockCollection[i][j]
# get the array from pixelblock in the 1st raster
array_in_raster1 = pixelblocklist[0].getData()
# get the array from pixelblock in the 2nd raster
array_ in_raster2 = pixelblocklist[1].getData()
forest_in_raster1= array_in_raster1[array_in_raster1==1] # value = 1 is forest class
urban_in_raster2= array_in_raster2[array_in_raster2==3] # value = 3 is urban class
res= forest_in_raster1+ urban_in_raster2
forest_to_urban_cell_count+= np.count_nonzero(res == 4) # value = 4 is forest in in_raster1 and urban in in_raster2
forest_to_urban_area= forest_to_urban_cell_count * in_raster1.meanCellWidth*in_raster1.meanCellHeight
print("total area from forest in 2006 to urban in 2016 : " + str(forest_to_urban_area))