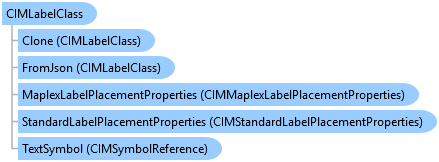
public class CIMLabelClass : CIMObject, System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
Public Class CIMLabelClass Inherits CIMObject Implements System.ComponentModel.INotifyPropertyChanged, System.Xml.Serialization.IXmlSerializable
public async Task CreatingAnAnnotationFeature(Geodatabase geodatabase) { await ArcGIS.Desktop.Framework.Threading.Tasks.QueuedTask.Run(() => { using (AnnotationFeatureClass annotationFeatureClass = geodatabase.OpenDataset<AnnotationFeatureClass>("Annotation // feature // class // name")) using (AnnotationFeatureClassDefinition annotationFeatureClassDefinition = annotationFeatureClass.GetDefinition()) using (RowBuffer rowBuffer = annotationFeatureClass.CreateRowBuffer()) using (AnnotationFeature annotationFeature = annotationFeatureClass.CreateRow(rowBuffer)) { annotationFeature.SetAnnotationClassID(0); annotationFeature.SetStatus(AnnotationStatus.Placed); // Get the annotation labels from the label collection IReadOnlyList<CIMLabelClass> labelClasses = annotationFeatureClassDefinition.GetLabelClassCollection(); // Setup the symbol reference with the symbol id and the text symbol CIMSymbolReference cimSymbolReference = new CIMSymbolReference(); cimSymbolReference.Symbol = labelClasses[0].TextSymbol.Symbol; cimSymbolReference.SymbolName = labelClasses[0].TextSymbol.SymbolName; // Setup the text graphic CIMTextGraphic cimTextGraphic = new CIMTextGraphic(); cimTextGraphic.Text = "Charlotte, North Carolina"; cimTextGraphic.Shape = new MapPointBuilderEx(new Coordinate2D(-80.843, 35.234), SpatialReferences.WGS84).ToGeometry(); cimTextGraphic.Symbol = cimSymbolReference; // Set the symbol reference on the graphic and store annotationFeature.SetGraphic(cimTextGraphic); annotationFeature.Store(); } }); }
// Creating a Cities annotation feature class // with following user defined fields // Name // GlobalID // Annotation feature class name string annotationFeatureClassName = "CitiesAnnotation"; // Create user defined attribute fields for annotation feature class FieldDescription globalIDFieldDescription = FieldDescription.CreateGlobalIDField(); FieldDescription nameFieldDescription = FieldDescription.CreateStringField("Name", 255); // Create a list of all field descriptions List<FieldDescription> fieldDescriptions = new List<FieldDescription> { globalIDFieldDescription, nameFieldDescription }; // Create a ShapeDescription object ShapeDescription shapeDescription = new ShapeDescription(GeometryType.Polygon, spatialReference); // Create general placement properties for Maplex engine CIMMaplexGeneralPlacementProperties generalPlacementProperties = new CIMMaplexGeneralPlacementProperties { AllowBorderOverlap = true, PlacementQuality = MaplexQualityType.High, DrawUnplacedLabels = true, InvertedLabelTolerance = 1.0, RotateLabelWithDisplay = true, UnplacedLabelColor = new CIMRGBColor { R = 0, G = 255, B = 0, Alpha = 0.5f // Green } }; // Create general placement properties for Standard engine //CIMStandardGeneralPlacementProperties generalPlacementProperties = // new CIMStandardGeneralPlacementProperties // { // DrawUnplacedLabels = true, // InvertedLabelTolerance = 3.0, // RotateLabelWithDisplay = true, // UnplacedLabelColor = new CIMRGBColor // { // R = 255, G = 0, B = 0, Alpha = 0.5f // Red // } // }; // Create annotation label classes // Green label CIMLabelClass greenLabelClass = new CIMLabelClass { Name = "Green", ExpressionTitle = "Expression-Green", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 1, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Tahoma", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 255, 0) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Green" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Blue label CIMLabelClass blueLabelClass = new CIMLabelClass { Name = "Blue", ExpressionTitle = "Expression-Blue", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 2, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Consolas", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 0, 255) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Blue" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Create a list of labels List<CIMLabelClass> labelClasses = new List<CIMLabelClass> { greenLabelClass, blueLabelClass }; // Create an annotation feature class description object to describe the feature class to create AnnotationFeatureClassDescription annotationFeatureClassDescription = new AnnotationFeatureClassDescription(annotationFeatureClassName, fieldDescriptions, shapeDescription, generalPlacementProperties, labelClasses) { IsAutoCreate = true, IsSymbolIDRequired = false, IsUpdatedOnShapeChange = true }; // Create a SchemaBuilder object SchemaBuilder schemaBuilder = new SchemaBuilder(geodatabase); // Add the creation of the Cities annotation feature class to the list of DDL tasks schemaBuilder.Create(annotationFeatureClassDescription); // Execute the DDL bool success = schemaBuilder.Build(); // Inspect error messages if (!success) { IReadOnlyList<string> errorMessages = schemaBuilder.ErrorMessages; //etc. }
// Creating a feature-linked annotation feature class between water pipe and valve in water distribution network // with following user defined fields // PipeName // GlobalID // Annotation feature class name string annotationFeatureClassName = "WaterPipeAnnotation"; // Create user defined attribute fields for annotation feature class FieldDescription pipeGlobalID = FieldDescription.CreateGlobalIDField(); FieldDescription nameFieldDescription = FieldDescription.CreateStringField("Name", 255); // Create a list of all field descriptions List<FieldDescription> fieldDescriptions = new List<FieldDescription> { pipeGlobalID, nameFieldDescription }; // Create a ShapeDescription object ShapeDescription shapeDescription = new ShapeDescription(GeometryType.Polygon, spatialReference); // Create general placement properties for Maplex engine CIMMaplexGeneralPlacementProperties generalPlacementProperties = new CIMMaplexGeneralPlacementProperties { AllowBorderOverlap = true, PlacementQuality = MaplexQualityType.High, DrawUnplacedLabels = true, InvertedLabelTolerance = 1.0, RotateLabelWithDisplay = true, UnplacedLabelColor = new CIMRGBColor { R = 255, G = 0, B = 0, Alpha = 0.5f } }; // Create annotation label classes // Green label CIMLabelClass greenLabelClass = new CIMLabelClass { Name = "Green", ExpressionTitle = "Expression-Green", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 1, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Tahoma", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 255, 0) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Green" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Blue label CIMLabelClass blueLabelClass = new CIMLabelClass { Name = "Blue", ExpressionTitle = "Expression-Blue", ExpressionEngine = LabelExpressionEngine.Arcade, Expression = "$feature.OBJECTID", ID = 2, Priority = 0, Visibility = true, TextSymbol = new CIMSymbolReference { Symbol = new CIMTextSymbol() { Angle = 45, FontType = FontType.Type1, FontFamilyName = "Consolas", FontEffects = FontEffects.Normal, HaloSize = 2.0, Symbol = new CIMPolygonSymbol { SymbolLayers = new CIMSymbolLayer[] { new CIMSolidFill { Color = CIMColor.CreateRGBColor(0, 0, 255) } }, UseRealWorldSymbolSizes = true } }, MaxScale = 0, MinScale = 0, SymbolName = "TextSymbol-Blue" }, StandardLabelPlacementProperties = new CIMStandardLabelPlacementProperties { AllowOverlappingLabels = true, LineOffset = 1.0 }, MaplexLabelPlacementProperties = new CIMMaplexLabelPlacementProperties { AlignLabelToLineDirection = true, AvoidPolygonHoles = true } }; // Create a list of labels List<CIMLabelClass> labelClasses = new List<CIMLabelClass> { greenLabelClass, blueLabelClass }; // Create linked feature description // Linked feature class name string linkedFeatureClassName = "WaterPipe"; // Create fields for water pipe FieldDescription waterPipeGlobalID = FieldDescription.CreateGlobalIDField(); FieldDescription pipeName = FieldDescription.CreateStringField("PipeName", 255); // Create a list of water pipe field descriptions List<FieldDescription> pipeFieldDescriptions = new List<FieldDescription> { waterPipeGlobalID, pipeName }; // Create a linked feature class description FeatureClassDescription linkedFeatureClassDescription = new FeatureClassDescription(linkedFeatureClassName, pipeFieldDescriptions, new ShapeDescription(GeometryType.Polyline, spatialReference)); // Create a SchemaBuilder object SchemaBuilder schemaBuilder = new SchemaBuilder(geodatabase); // Add the creation of the linked feature class to the list of DDL tasks FeatureClassToken linkedFeatureClassToken = schemaBuilder.Create(linkedFeatureClassDescription); // Create an annotation feature class description object to describe the feature class to create AnnotationFeatureClassDescription annotationFeatureClassDescription = new AnnotationFeatureClassDescription(annotationFeatureClassName, fieldDescriptions, shapeDescription, generalPlacementProperties, labelClasses, new FeatureClassDescription(linkedFeatureClassToken)) { IsAutoCreate = true, IsSymbolIDRequired = false, IsUpdatedOnShapeChange = true }; // Add the creation of the annotation feature class to the list of DDL tasks schemaBuilder.Create(annotationFeatureClassDescription); // Execute the DDL bool success = schemaBuilder.Build(); // Inspect error messages if (!success) { IReadOnlyList<string> errorMessages = schemaBuilder.ErrorMessages; //etc. }
var lyr = MapView.Active.Map.GetLayersAsFlattenedList().OfType<FeatureLayer>().FirstOrDefault(f => f.ShapeType == esriGeometryType.esriGeometryPolygon); if (lyr == null) return; QueuedTask.Run(() => { //Get the layer's definition //community sample Data\Admin\AdminSample.aprx var lyrDefn = lyr.GetDefinition() as CIMFeatureLayer; if (lyrDefn == null) return; //Get the label classes - we need the first one var listLabelClasses = lyrDefn.LabelClasses.ToList(); var theLabelClass = listLabelClasses.FirstOrDefault(); //set the label class Expression to use the Arcade expression theLabelClass.Expression = "return $feature.STATE_NAME + TextFormatting.NewLine + $feature.POP2000;"; //Set the label definition back to the layer. lyr.SetDefinition(lyrDefn); });
//Note: call within QueuedTask.Run() //Get the layer's definition var lyrDefn = featureLayer.GetDefinition() as CIMFeatureLayer; //Get the label classes - we need the first one var listLabelClasses = lyrDefn.LabelClasses.ToList(); var theLabelClass = listLabelClasses.FirstOrDefault(); //Set the label classes' symbol to the custom text symbol //Refer to the ProSnippets-TextSymbols wiki page for help with creating custom text symbols. //Example: var textSymbol = await CreateTextSymbolWithHaloAsync(); theLabelClass.TextSymbol.Symbol = textSymbol; lyrDefn.LabelClasses = listLabelClasses.ToArray(); //Set the labelClasses back featureLayer.SetDefinition(lyrDefn); //set the layer's definition //set the label's visiblity featureLayer.SetLabelVisibility(true);
//Note: call within QueuedTask.Run() //Get the layer's definition var lyrDefn = featureLayer.GetDefinition() as CIMFeatureLayer; //Get the label classes - we need the first one var listLabelClasses = lyrDefn.LabelClasses.ToList(); var theLabelClass = listLabelClasses.FirstOrDefault(); //Modify label Placement //Check if the label engine is Maplex or standard. CIMGeneralPlacementProperties labelEngine = MapView.Active.Map.GetDefinition().GeneralPlacementProperties; if (labelEngine is CIMStandardGeneralPlacementProperties) //Current labeling engine is Standard labeling engine theLabelClass.StandardLabelPlacementProperties.PointPlacementMethod = StandardPointPlacementMethod.OnTopPoint; else //Current labeling engine is Maplex labeling engine theLabelClass.MaplexLabelPlacementProperties.PointPlacementMethod = MaplexPointPlacementMethod.CenteredOnPoint; lyrDefn.LabelClasses = listLabelClasses.ToArray(); //Set the labelClasses back featureLayer.SetDefinition(lyrDefn); //set the layer's definition
System.Object
ArcGIS.Core.CIM.CIMObject
ArcGIS.Core.CIM.CIMLabelClass
Target Platforms: Windows 11, Windows 10, Windows 8.1